Hello! I am having trouble on how to write out the Java code based on the instructions (in bold //***) that I am given. As a note, this specific Java code is a code that is going to setup the basic framework for a game of Yahtzee. In other words, you will need to understand the rules of Yahtzee and implement the rules into coding. I appreciate the help. Thanks! Also, I am using the IntelliJ IDEA application when writing out this program. Here is what I need help with: * The code given below is going to be modified based on the instructions in bold. public static int calculateThreeOfKind() { int score; score = 0; boolean isThreeKind; isThreeKind = false; System.out.println("In method calculateThreeOfKind"); return score; } //*** //*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE //*** //*** Modify your "calculateThreeOfKind" method as follows: //*** 1) Remove the System.out.println statement. //*** //*** 2) Create an if-else-if structure to determine if the five (5) //*** dice form a valid three-of-a-kind. //*** A) You MUST use an if-else-if structure. //*** B) Your if-statement conditions MUST be compound Boolean expressions. //*** In other words, the if-statement conditions must have multiple //*** expressions joined by && (AND) or || (OR) operators. //*** C) I see a number of valid design approaches so as long as the //*** above conditions are met, I leave the rest of the design //*** decisions to you. //*** //*** 3) In your if-else-if structure, if it is determined that the //*** configuration of the dice form a valid three-of-a-kind, //*** then assign the value of true to the variable "isThreeKind". //*** //*** 4) Write an if-statement that checks if variable "isThreeKind" is true. //*** //*** 5) Code block for if-statement in Step 4: //*** A) Add the values of variables "die1", "die2", "die3", "die4", //*** and "die5" together and assign that value to variable "score". //*** //*** 6) Keep the return statement that returns the variable "score". //*** //*** Hint: Here are the combinations of dice variables that form a valid three-of-a-kind: //*** 1) Variables "die1", "die2" and "die3" are equal //*** 2) Variables "die1", "die2" and "die4" are equal //*** 3) Variables "die1", "die2" and "die5" are equal //*** 4) Variables "die1", "die3" and "die4" are equal //*** 5) Variables "die1", "die3" and "die5" are equal //*** 6) Variables "die1", "die4" and "die5" are equal //*** 7) Variables "die2", "die3" and "die4" are equal //*** 8) Variables "die2", "die3" and "die5" are equal //*** 9) Variables "die2", "die4" and "die5" are equal //*** 10) Variables "die3", "die4" and "die5" are equal //***
Hello! I am having trouble on how to write out the Java code based on the instructions (in bold //***) that I am given. As a note, this specific Java code is a code that is going to setup the basic framework for a game of Yahtzee. In other words, you will need to understand the rules of Yahtzee and implement the rules into coding. I appreciate the help. Thanks! Also, I am using the IntelliJ IDEA application when writing out this program. Here is what I need help with:
* The code given below is going to be modified based on the instructions in bold.
public static int calculateThreeOfKind() {
int score;
score = 0;
boolean isThreeKind;
isThreeKind = false;
System.out.println("In method calculateThreeOfKind");
return score;
}
//***
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE
//***
//*** Modify your "calculateThreeOfKind" method as follows:
//*** 1) Remove the System.out.println statement.
//***
//*** 2) Create an if-else-if structure to determine if the five (5)
//*** dice form a valid three-of-a-kind.
//*** A) You MUST use an if-else-if structure.
//*** B) Your if-statement conditions MUST be compound Boolean expressions.
//*** In other words, the if-statement conditions must have multiple
//*** expressions joined by && (AND) or || (OR) operators.
//*** C) I see a number of valid design approaches so as long as the
//*** above conditions are met, I leave the rest of the design
//*** decisions to you.
//***
//*** 3) In your if-else-if structure, if it is determined that the
//*** configuration of the dice form a valid three-of-a-kind,
//*** then assign the value of true to the variable "isThreeKind".
//***
//*** 4) Write an if-statement that checks if variable "isThreeKind" is true.
//***
//*** 5) Code block for if-statement in Step 4:
//*** A) Add the values of variables "die1", "die2", "die3", "die4",
//*** and "die5" together and assign that value to variable "score".
//***
//*** 6) Keep the return statement that returns the variable "score".
//***
//*** Hint: Here are the combinations of dice variables that form a valid three-of-a-kind:
//*** 1) Variables "die1", "die2" and "die3" are equal
//*** 2) Variables "die1", "die2" and "die4" are equal
//*** 3) Variables "die1", "die2" and "die5" are equal
//*** 4) Variables "die1", "die3" and "die4" are equal
//*** 5) Variables "die1", "die3" and "die5" are equal
//*** 6) Variables "die1", "die4" and "die5" are equal
//*** 7) Variables "die2", "die3" and "die4" are equal
//*** 8) Variables "die2", "die3" and "die5" are equal
//*** 9) Variables "die2", "die4" and "die5" are equal
//*** 10) Variables "die3", "die4" and "die5" are equal
//***

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

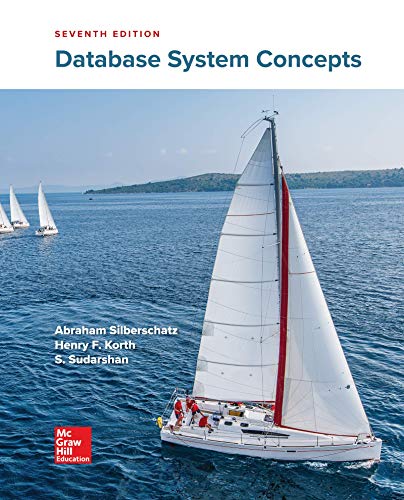
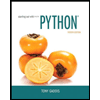
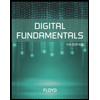
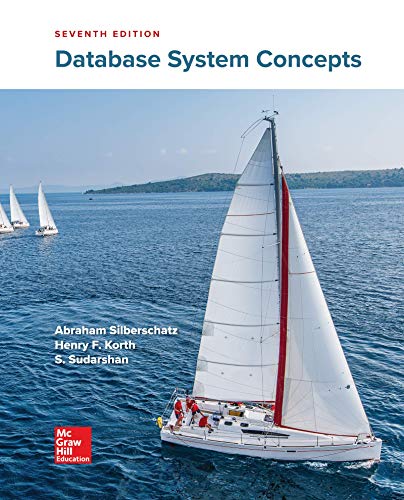
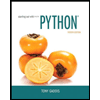
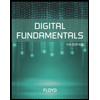
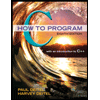
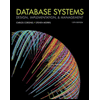
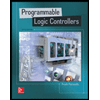