Hello! I am having trouble on how to write out the Java code based on the instructions (in bold //***) that I am given. As a note, this specific Java code is a code that is going to setup the basic framework for a game of Yahtzee. In other words, you will need to understand the rules of Yahtzee and implement the rules into coding. Could you also add comments to each line of code when you write out the codes for this as welI? I appreciate the help. Thanks! Also, I am using the IntelliJ IDEA application when writing out this program. Here is what I need help with: public static void displayScoreSheet() { final String ACES_LABEL = "Aces"; final String TWOS_LABEL = "Twos"; final String THREES_LABEL = "Threes"; final String FOURS_LABEL = "Fours"; final String FIVES_LABEL = "Fives"; final String SIXES_LABEL = "Sixes"; final String THREE_KIND_LABEL = "3 of a kind"; final String FOUR_KIND_LABEL = "4 of a kind"; final String FULL_HOUSE_LABEL = "Full House"; final String SMALL_STRAIGHT_LABEL = "Sm. Straight"; final String LARGE_STRAIGHT_LABEL = "Lg. Straight"; final String YAHTZEE_LABEL = "YAHTZEE"; final String CHANCE_LABEL = "Chance"; final String YAHTZEE_BONUS_LABEL = "YAHTZEE BONUS"; final int BONUS_THRESHOLD = 63; final int BONUS_SCORE = 35; final String UPPER_SECTION_LABEL = "UPPER SECTION"; final String LOWER_SECTION_LABEL = "LOWER SECTION"; final String UPPER_SECTION_SUBTOTAL_LABEL = "TOTAL SCORE"; final String UPPER_SECTION_BONUS_LABEL = "BONUS if >= 63"; final String UPPER_SECTION_TOTAL_LABEL = "TOTAL of Upper Section"; final String LOWER_SECTION_TOTAL_LABEL = "TOTAL of Lower Section"; final String GRAND_TOTAL_LABEL = "GRAND TOTAL"; final String OPTION_SUFFIX_ONE_DIGIT = ") "; final String OPTION_SUFFIX_TWO_DIGIT = ") "; final String FIRST_OPTION_LABEL = "1"; final String SECOND_OPTION_LABEL = "2"; final String THIRD_OPTION_LABEL = "3"; final String FOURTH_OPTION_LABEL = "4"; final String FIFTH_OPTION_LABEL = "5"; final String SIXTH_OPTION_LABEL = "6"; final String SEVENTH_OPTION_LABEL = "7"; final String EIGHTH_OPTION_LABEL = "8"; final String NINTH_OPTION_LABEL = "9"; final String TENTH_OPTION_LABEL = "10"; final String ELEVENTH_OPTION_LABEL = "11"; final String TWELFTH_OPTION_LABEL = "12"; final String THIRTEENTH_OPTION_LABEL = "13"; final String EQUALS_LABEL = " = "; //*** //*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE //*** //*** 1) Declare a variable named "upperScoreTotal" of int datatype. //*** //*** 2) Call method "calculateUpperScore" passing no arguments, assigning //*** this method call to variable "upperScoreTotal". //*** //*** 3) Declare a variable named "lowerScoreTotal" of int datatype. //*** //*** 4) Call method "calculateLowerScore" passing no arguments, assigning //*** this method call to variable "lowerScoreTotal". //*** //*** //*** Your code for Steps 1-4 goes here. //*** System.out.println(); System.out.println(UPPER_SECTION_LABEL); if (aces == SCORE_NO_VALUE) System.out.println(FIRST_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + ACES_LABEL); else System.out.println(FIRST_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + ACES_LABEL + EQUALS_LABEL + aces); //*** //*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE //*** //*** 1) Following the form of the if-statement-block for option Aces (lines 667-671): //*** A) Write an if-statement-block for each of following variables //*** that check the variables for "equality to" SCORE_NO_VALUE: //*** a) "twos" //*** b) "threes" //*** c) "fours" //*** d) "fives" //*** e) "sixes" //*** * In other words, there will be five (5) if-statement-blocks, each one //*** must compare one of the listed variables to SCORE_NO_VALUE. //*** B) Use the correct Java syntax for the "equality" operator. //*** //*** NOTE: 1) Don't forget to use the different OPTION_LABEL constants when //*** writing the code for Step 1. //*** 2) Comment your code following the comment given for Aces. Include //*** the option number and option name. //*** //*** //*** Your code for Step 1 goes here. //***
Hello! I am having trouble on how to write out the Java code based on the instructions (in bold //***) that I am given. As a note, this specific Java code is a code that is going to setup the basic framework for a game of Yahtzee. In other words, you will need to understand the rules of Yahtzee and implement the rules into coding. Could you also add comments to each line of code when you write out the codes for this as welI? I appreciate the help. Thanks! Also, I am using the IntelliJ IDEA application when writing out this program. Here is what I need help with:
public static void displayScoreSheet() {
final String ACES_LABEL = "Aces";
final String TWOS_LABEL = "Twos";
final String THREES_LABEL = "Threes";
final String FOURS_LABEL = "Fours";
final String FIVES_LABEL = "Fives";
final String SIXES_LABEL = "Sixes";
final String THREE_KIND_LABEL = "3 of a kind";
final String FOUR_KIND_LABEL = "4 of a kind";
final String FULL_HOUSE_LABEL = "Full House";
final String SMALL_STRAIGHT_LABEL = "Sm. Straight";
final String LARGE_STRAIGHT_LABEL = "Lg. Straight";
final String YAHTZEE_LABEL = "YAHTZEE";
final String CHANCE_LABEL = "Chance";
final String YAHTZEE_BONUS_LABEL = "YAHTZEE BONUS";
final int BONUS_THRESHOLD = 63;
final int BONUS_SCORE = 35;
final String UPPER_SECTION_LABEL = "UPPER SECTION";
final String LOWER_SECTION_LABEL = "LOWER SECTION";
final String UPPER_SECTION_SUBTOTAL_LABEL = "TOTAL SCORE";
final String UPPER_SECTION_BONUS_LABEL = "BONUS if >= 63";
final String UPPER_SECTION_TOTAL_LABEL = "TOTAL of Upper Section";
final String LOWER_SECTION_TOTAL_LABEL = "TOTAL of Lower Section";
final String GRAND_TOTAL_LABEL = "GRAND TOTAL";
final String OPTION_SUFFIX_ONE_DIGIT = ") ";
final String OPTION_SUFFIX_TWO_DIGIT = ") ";
final String FIRST_OPTION_LABEL = "1";
final String SECOND_OPTION_LABEL = "2";
final String THIRD_OPTION_LABEL = "3";
final String FOURTH_OPTION_LABEL = "4";
final String FIFTH_OPTION_LABEL = "5";
final String SIXTH_OPTION_LABEL = "6";
final String SEVENTH_OPTION_LABEL = "7";
final String EIGHTH_OPTION_LABEL = "8";
final String NINTH_OPTION_LABEL = "9";
final String TENTH_OPTION_LABEL = "10";
final String ELEVENTH_OPTION_LABEL = "11";
final String TWELFTH_OPTION_LABEL = "12";
final String THIRTEENTH_OPTION_LABEL = "13";
final String EQUALS_LABEL = " = ";
//***
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE
//***
//*** 1) Declare a variable named "upperScoreTotal" of int datatype.
//***
//*** 2) Call method "calculateUpperScore" passing no arguments, assigning
//*** this method call to variable "upperScoreTotal".
//***
//*** 3) Declare a variable named "lowerScoreTotal" of int datatype.
//***
//*** 4) Call method "calculateLowerScore" passing no arguments, assigning
//*** this method call to variable "lowerScoreTotal".
//***
//***
//*** Your code for Steps 1-4 goes here.
//***
System.out.println();
System.out.println(UPPER_SECTION_LABEL);
if (aces == SCORE_NO_VALUE)
System.out.println(FIRST_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + ACES_LABEL);
else
System.out.println(FIRST_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + ACES_LABEL + EQUALS_LABEL + aces);
//***
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE
//***
//*** 1) Following the form of the if-statement-block for option Aces (lines 667-671):
//*** A) Write an if-statement-block for each of following variables
//*** that check the variables for "equality to" SCORE_NO_VALUE:
//*** a) "twos"
//*** b) "threes"
//*** c) "fours"
//*** d) "fives"
//*** e) "sixes"
//*** * In other words, there will be five (5) if-statement-blocks, each one
//*** must compare one of the listed variables to SCORE_NO_VALUE.
//*** B) Use the correct Java syntax for the "equality" operator.
//***
//*** NOTE: 1) Don't forget to use the different OPTION_LABEL constants when
//*** writing the code for Step 1.
//*** 2) Comment your code following the comment given for Aces. Include
//*** the option number and option name.
//***
//***
//*** Your code for Step 1 goes here.
//***

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

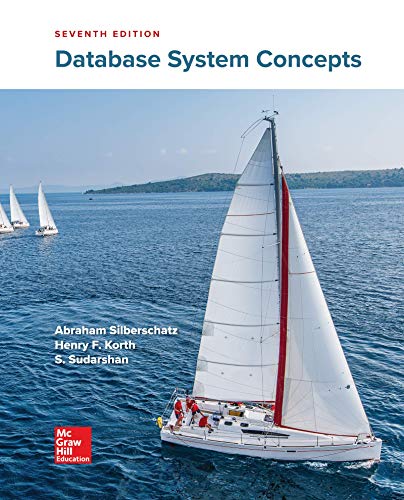
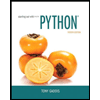
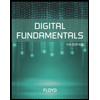
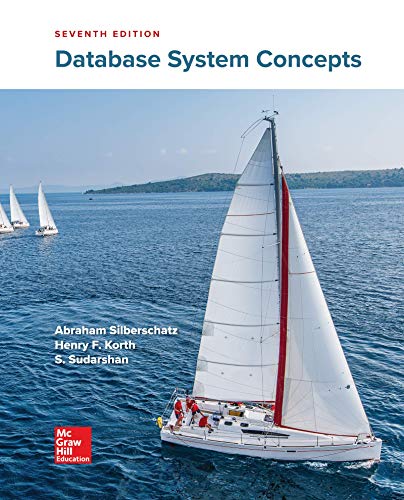
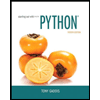
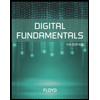
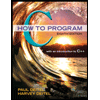
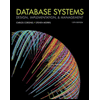
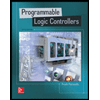