hello! can you help debug my Java program? here are the errors I am getting: line 67: id cannot be resolved to a variableline 102: id cannot be resolved to a variableline 110: id cannot be resolved to a variableline 119: id cannot be resolved to a variable should I re-initialize ID somewhere? is it not a public enough variable? thank you for any insight you can give. here is my code: package automaticTellerMachine; //header importing files import java.text.DecimalFormat; import java.util.Scanner; //class Accountability class Account { public int id; public double balance; public Account() { //where the variables live id = 9; balance = 0; } public Account(int a, double b) { id = a; balance = b; } //defining the methods public double getBalance() { return balance; } //this is the depositing part public void deposit(double a) { //this is depositing at work balance = balance+a; } //this is the withdrawing part public void withdraw(double a) { //if you have enough $$$ if(a<=balance) { //this is the math part balance = balance-a; } //otherwise, error!!! else { //error in action! System.out.println("You do not have enough balance. Transaction ignored. Please try again."); } } } //new class: AutomaticMoneyMachine class AutomaticTellerMachine { //it's the main method! public static void main(String[] args) { //This is the Object of the Class Account[]account=new Account[10]; //terminate if i is greater or equal to 500 for (int i=0; i<500; i++) { //100 is a good starting amount account[id]=new Account(i, 100); } //loop while(true) { //this is the object for the Scanner class to pick up Scanner keyboard=new Scanner(System.in); System.out.println("Enter your account ID:"); int id=keyboard.nextInt(); //while loop repeats - ID must not be 0 and there are only 500 accounts, so while ((id>500)||(id<0)) { //hint for user about what went wrong System.out.println("Please try again. There are only 500 accounts at Longitude Banking. We are very exclusive."); id=keyboard.nextInt(); }} //while loop so that we can show the menu & the choices in it while(true) { //here is the scanner specifically for this menu Scanner sc=new Scanner(System.in); //it is the menu! System.out.println("Welcome!!!"); System.out.println("1: Current balance"); System.out.println("2: Deposit"); System.out.println("3: Withdraw"); System.out.println("4: Exit"); //what value? Let me file that away via Scanner int choice=sc.nextInt(); //balance choice if(choice==1) { System.out.println("You have $"+account[id].getBalance()+" in your account."); } //deposit choice else if(choice==2) { System.out.println("Enter the amount you want to deposit: "); double deposit = sc.nextDouble(); account[id].deposit(deposit); System.out.println("You have deposited"+deposit+"into your account."); } //withdrawing choice else if(choice==3) { System.out.print("Enter the amount you would like to withdraw: "); double withdraw=sc.nextDouble(); account[id].withdraw(withdraw); System.out.println("You have withdrawn"+withdraw+"from your account."); } //exit choice else if(choice==4) { //thank you message System.out.println("Successfully logged out. Thank you for banking with Longitude Banking."); //exit System.exit(0); } } } }
hello! can you help debug my Java program? here are the errors I am getting: line 67: id cannot be resolved to a variableline 102: id cannot be resolved to a variableline 110: id cannot be resolved to a variableline 119: id cannot be resolved to a variable should I re-initialize ID somewhere? is it not a public enough variable? thank you for any insight you can give. here is my code: package automaticTellerMachine; //header importing files import java.text.DecimalFormat; import java.util.Scanner; //class Accountability class Account { public int id; public double balance; public Account() { //where the variables live id = 9; balance = 0; } public Account(int a, double b) { id = a; balance = b; } //defining the methods public double getBalance() { return balance; } //this is the depositing part public void deposit(double a) { //this is depositing at work balance = balance+a; } //this is the withdrawing part public void withdraw(double a) { //if you have enough $$$ if(a<=balance) { //this is the math part balance = balance-a; } //otherwise, error!!! else { //error in action! System.out.println("You do not have enough balance. Transaction ignored. Please try again."); } } } //new class: AutomaticMoneyMachine class AutomaticTellerMachine { //it's the main method! public static void main(String[] args) { //This is the Object of the Class Account[]account=new Account[10]; //terminate if i is greater or equal to 500 for (int i=0; i<500; i++) { //100 is a good starting amount account[id]=new Account(i, 100); } //loop while(true) { //this is the object for the Scanner class to pick up Scanner keyboard=new Scanner(System.in); System.out.println("Enter your account ID:"); int id=keyboard.nextInt(); //while loop repeats - ID must not be 0 and there are only 500 accounts, so while ((id>500)||(id<0)) { //hint for user about what went wrong System.out.println("Please try again. There are only 500 accounts at Longitude Banking. We are very exclusive."); id=keyboard.nextInt(); }} //while loop so that we can show the menu & the choices in it while(true) { //here is the scanner specifically for this menu Scanner sc=new Scanner(System.in); //it is the menu! System.out.println("Welcome!!!"); System.out.println("1: Current balance"); System.out.println("2: Deposit"); System.out.println("3: Withdraw"); System.out.println("4: Exit"); //what value? Let me file that away via Scanner int choice=sc.nextInt(); //balance choice if(choice==1) { System.out.println("You have $"+account[id].getBalance()+" in your account."); } //deposit choice else if(choice==2) { System.out.println("Enter the amount you want to deposit: "); double deposit = sc.nextDouble(); account[id].deposit(deposit); System.out.println("You have deposited"+deposit+"into your account."); } //withdrawing choice else if(choice==3) { System.out.print("Enter the amount you would like to withdraw: "); double withdraw=sc.nextDouble(); account[id].withdraw(withdraw); System.out.println("You have withdrawn"+withdraw+"from your account."); } //exit choice else if(choice==4) { //thank you message System.out.println("Successfully logged out. Thank you for banking with Longitude Banking."); //exit System.exit(0); } } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
hello! can you help debug my Java program?
here are the errors I am getting:
line 67: id cannot be resolved to a variable
line 102: id cannot be resolved to a variable
line 110: id cannot be resolved to a variable
line 119: id cannot be resolved to a variable
should I re-initialize ID somewhere? is it not a public enough variable? thank you for any insight you can give.
here is my code:
package automaticTellerMachine;
//header importing files
import java.text.DecimalFormat;
import java.util.Scanner;
//class Accountability
class Account
{
public int id;
public double balance;
public Account()
{
//where the variables live
id = 9;
balance = 0;
}
public Account(int a, double b)
{
id = a;
balance = b;
}
//defining the methods
public double getBalance()
{
return balance;
}
//this is the depositing part
public void deposit(double a)
{
//this is depositing at work
balance = balance+a;
}
//this is the withdrawing part
public void withdraw(double a)
{
//if you have enough $$$
if(a<=balance)
{
//this is the math part
balance = balance-a;
}
//otherwise, error!!!
else
{
//error in action!
System.out.println("You do not have enough balance. Transaction ignored. Please try again.");
}
}
}
//new class: AutomaticMoneyMachine
class AutomaticTellerMachine
{
//it's the main method!
public static void main(String[] args)
{
//This is the Object of the Class
Account[]account=new Account[10];
//terminate if i is greater or equal to 500
for (int i=0; i<500; i++)
{
//100 is a good starting amount
account[id]=new Account(i, 100);
}
//loop
while(true)
{
//this is the object for the Scanner class to pick up
Scanner keyboard=new Scanner(System.in);
System.out.println("Enter your account ID:");
int id=keyboard.nextInt();
//while loop repeats - ID must not be 0 and there are only 500 accounts, so
while ((id>500)||(id<0))
{
//hint for user about what went wrong
System.out.println("Please try again. There are only 500 accounts at Longitude Banking. We are very exclusive.");
id=keyboard.nextInt();
}}
//while loop so that we can show the menu & the choices in it
while(true)
{
//here is the scanner specifically for this menu
Scanner sc=new Scanner(System.in);
//it is the menu!
System.out.println("Welcome!!!");
System.out.println("1: Current balance");
System.out.println("2: Deposit");
System.out.println("3: Withdraw");
System.out.println("4: Exit");
//what value? Let me file that away via Scanner
int choice=sc.nextInt();
//balance choice
if(choice==1)
{
System.out.println("You have $"+account[id].getBalance()+" in your account.");
}
//deposit choice
else if(choice==2)
{
System.out.println("Enter the amount you want to deposit: ");
double deposit = sc.nextDouble();
account[id].deposit(deposit);
System.out.println("You have deposited"+deposit+"into your account.");
}
//withdrawing choice
else if(choice==3)
{
System.out.print("Enter the amount you would like to withdraw: ");
double withdraw=sc.nextDouble();
account[id].withdraw(withdraw);
System.out.println("You have withdrawn"+withdraw+"from your account.");
}
//exit choice
else if(choice==4)
{
//thank you message
System.out.println("Successfully logged out. Thank you for banking with Longitude Banking.");
//exit
System.exit(0);
}
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
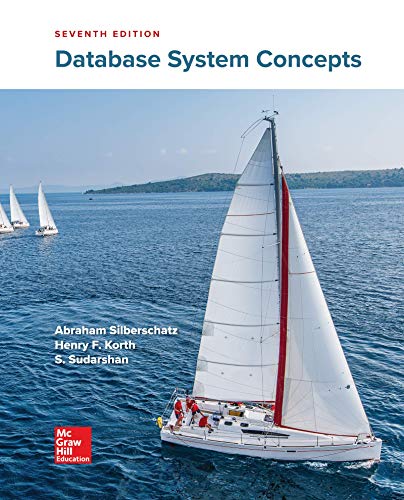
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
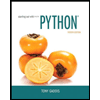
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
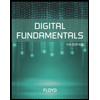
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
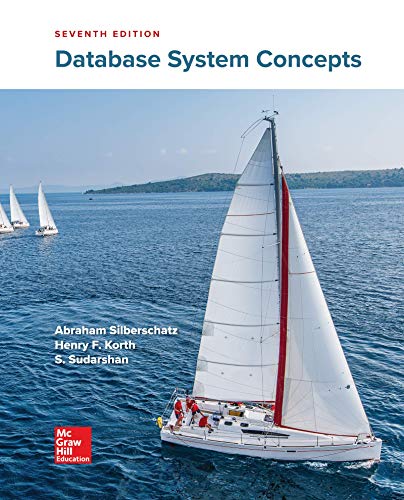
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
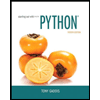
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
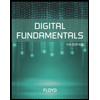
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
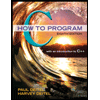
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
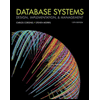
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
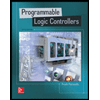
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education