There are a few errors in this java code: can you fix it please, its basically a debugging exercise: public static int sum(int n){ int n; for (int i=1; i<=n;i--){ sum++; } return sum; } }
There are a few errors in this java code: can you fix it please, its basically a debugging exercise: public static int sum(int n){ int n; for (int i=1; i<=n;i--){ sum++; } return sum; } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
There are a few errors in this java code: can you fix it please, its basically a debugging exercise:
public static int sum(int n){
int n;
for (int i=1; i<=n;i--){
sum++;
}
return sum;
}
}
![The image shows a screenshot of the Eclipse IDE with Java code editor open. Below is the transcription and explanation based on the visible content:
### Project Explorer Panel
- **Lab 9**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Apples.java`
- `DoWhileLoops.java`
- `ForLoops.java`
- `WhileLoops.java`
- **Quiz**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Blue.java`
- `Pumpkins.java`
### Java Code Editor
The file `Blue.java` is open in the editor with the following code:
```java
public class Blue {
public static int sum(int n){
int sum;
for (int i = 1; i <= n; i--){
sum++;
}
return sum;
}
}
```
### Error in Console
The console displays an error message:
```
Error: Main method must return a value of type void in Class Blue, please define the main method as:
public static void main(String[] args)
```
### Explanation
- The code defines a `public class Blue` with a `static int` method called `sum`.
- The method `sum` takes an integer `n` as a parameter, initializes an integer `sum`, and attempts to increment it in a `for` loop with a decrementing iterator, which will result in an infinite loop if `i` starts at 1.
- The method returns the value of `sum`, but `sum` is not initialized, which would lead to a compilation error.
- The console error indicates that the `main` method is missing, which is necessary to execute the program.
### Recommendations for Correction
1. **Initialization Error:** Initialize the `sum` variable.
2. **Loop Direction:** Correct the loop condition and iterator modification to `i++` if intended increment within the loop.
3. **Main Method:** Add a `main` method to execute the program, as per console message guidance:
```java
public static void main(String[] args) {
// Example execution
int result = sum(5);
System.out.println("Sum: " + result);
}
```
This setup is suitable for educational purposes to understand common beginner errors in Java programming.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7d848391-a5b1-45af-b291-d5739fd6d88e%2Feb841c67-91ab-4e50-b7ba-efc06f55b1f5%2Fs5hmdj_processed.png&w=3840&q=75)
Transcribed Image Text:The image shows a screenshot of the Eclipse IDE with Java code editor open. Below is the transcription and explanation based on the visible content:
### Project Explorer Panel
- **Lab 9**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Apples.java`
- `DoWhileLoops.java`
- `ForLoops.java`
- `WhileLoops.java`
- **Quiz**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Blue.java`
- `Pumpkins.java`
### Java Code Editor
The file `Blue.java` is open in the editor with the following code:
```java
public class Blue {
public static int sum(int n){
int sum;
for (int i = 1; i <= n; i--){
sum++;
}
return sum;
}
}
```
### Error in Console
The console displays an error message:
```
Error: Main method must return a value of type void in Class Blue, please define the main method as:
public static void main(String[] args)
```
### Explanation
- The code defines a `public class Blue` with a `static int` method called `sum`.
- The method `sum` takes an integer `n` as a parameter, initializes an integer `sum`, and attempts to increment it in a `for` loop with a decrementing iterator, which will result in an infinite loop if `i` starts at 1.
- The method returns the value of `sum`, but `sum` is not initialized, which would lead to a compilation error.
- The console error indicates that the `main` method is missing, which is necessary to execute the program.
### Recommendations for Correction
1. **Initialization Error:** Initialize the `sum` variable.
2. **Loop Direction:** Correct the loop condition and iterator modification to `i++` if intended increment within the loop.
3. **Main Method:** Add a `main` method to execute the program, as per console message guidance:
```java
public static void main(String[] args) {
// Example execution
int result = sum(5);
System.out.println("Sum: " + result);
}
```
This setup is suitable for educational purposes to understand common beginner errors in Java programming.
Expert Solution

Step 1
- To resolve this error you have to declare the main method which is the initial method to be executed.
- In the sum function, you've already defined int n and you're declaring the same variable inside the sum function again.
- Initialize the sum variable.
- You need to increment the i variable in for loop in order to loop over n elements. (i++)
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
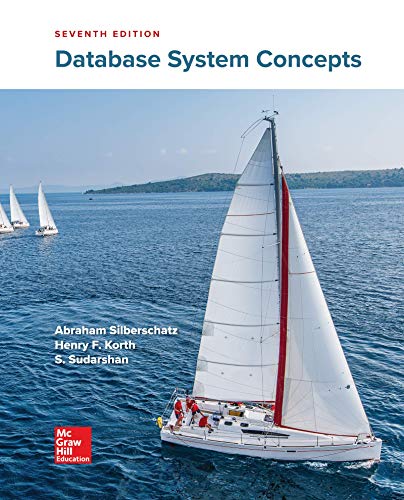
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
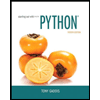
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
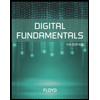
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
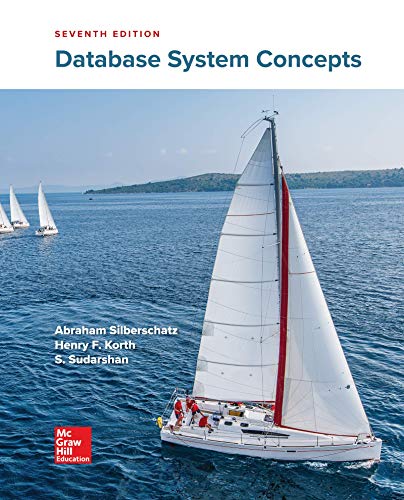
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
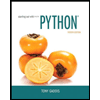
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
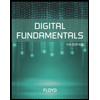
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
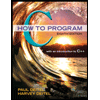
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
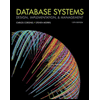
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
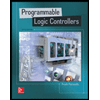
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education