Given the IntNode class, define the findMax() method in the CustomLinkedList class that returns the largest value in the list or returns -99 if the list is empty. Assume all values in the list are non-negative. Ex: If the list contains: head -> 14 -> 191 -> 186 -> 181 findMax(headObj) returns 191. Ex: If the list contains: head -> findMax(headObj) returns -99. public class CustomLinkedList { public static int findMax(IntNode headObj) { if (headObj.getNext() == null) { return -99; // List is empty } int max = headObj.getNext().getData(); IntNode current = headObj.getNext().getNext(); // Traverse the linked list to find the maximum value while (current != null) { int data = current.getData(); if (data > max) { max = data; } current = current.getNext(); } return max; } public static void main(String[] args) { IntNode headObj; IntNode currObj; IntNode lastObj; int i; int max; // Create head node headObj = new IntNode(-1); lastObj = headObj; // Add nodes to the list for (i = 0; i < 20; ++i) { currObj = new IntNode(i); lastObj.insertAfter(currObj); lastObj = currObj; } max = findMax(headObj); System.out.println(max); } } public class IntNode { private int dataVal; // Node data private IntNode nextNodePtr; // Reference to the next node public IntNode() { dataVal = 0; nextNodePtr = null; } // Constructor public IntNode(int dataInit) { this.dataVal = dataInit; this.nextNodePtr = null; } // Constructor public IntNode(int dataInit, IntNode nextLoc) { this.dataVal = dataInit; this.nextNodePtr = nextLoc; } /* Insert node after this node. Before: this -- next After: this -- node -- next */ public void insertAfter(IntNode nodeLoc) { IntNode tmpNext; tmpNext = this.nextNodePtr; this.nextNodePtr = nodeLoc; nodeLoc.nextNodePtr = tmpNext; } // Get location pointed by nextNodePtr public IntNode getNext() { return this.nextNodePtr; } // Get node value public int getNodeData() { return this.dataVal; } // Print node value public void printNodeData() { System.out.println(this.dataVal); } }
Given the IntNode class, define the findMax() method in the CustomLinkedList class that returns the largest value in the list or returns -99 if the list is empty. Assume all values in the list are non-negative.
Ex: If the list contains:
head -> 14 -> 191 -> 186 -> 181
findMax(headObj) returns 191.
Ex: If the list contains:
head ->
findMax(headObj) returns -99.
public class CustomLinkedList {
public static int findMax(IntNode headObj) {
if (headObj.getNext() == null) {
return -99; // List is empty
}
int max = headObj.getNext().getData();
IntNode current = headObj.getNext().getNext();
// Traverse the linked list to find the maximum value
while (current != null) {
int data = current.getData();
if (data > max) {
max = data;
}
current = current.getNext();
}
return max;
}
public static void main(String[] args) {
IntNode headObj;
IntNode currObj;
IntNode lastObj;
int i;
int max;
// Create head node
headObj = new IntNode(-1);
lastObj = headObj;
// Add nodes to the list
for (i = 0; i < 20; ++i) {
currObj = new IntNode(i);
lastObj.insertAfter(currObj);
lastObj = currObj;
}
max = findMax(headObj);
System.out.println(max);
}
}
public class IntNode {
private int dataVal; // Node data
private IntNode nextNodePtr; // Reference to the next node
public IntNode() {
dataVal = 0;
nextNodePtr = null;
}
// Constructor
public IntNode(int dataInit) {
this.dataVal = dataInit;
this.nextNodePtr = null;
}
// Constructor
public IntNode(int dataInit, IntNode nextLoc) {
this.dataVal = dataInit;
this.nextNodePtr = nextLoc;
}
/* Insert node after this node.
Before: this -- next
After: this -- node -- next
*/
public void insertAfter(IntNode nodeLoc) {
IntNode tmpNext;
tmpNext = this.nextNodePtr;
this.nextNodePtr = nodeLoc;
nodeLoc.nextNodePtr = tmpNext;
}
// Get location pointed by nextNodePtr
public IntNode getNext() {
return this.nextNodePtr;
}
// Get node value
public int getNodeData() {
return this.dataVal;
}
// Print node value
public void printNodeData() {
System.out.println(this.dataVal);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 8 images

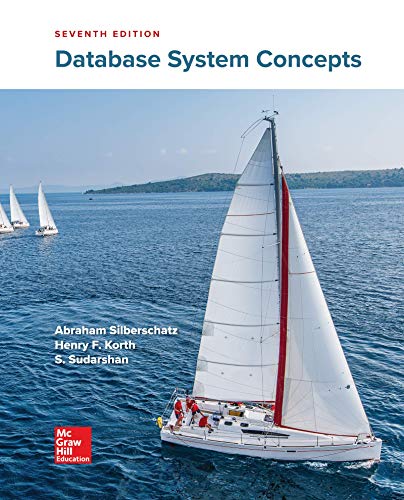
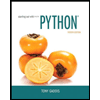
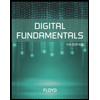
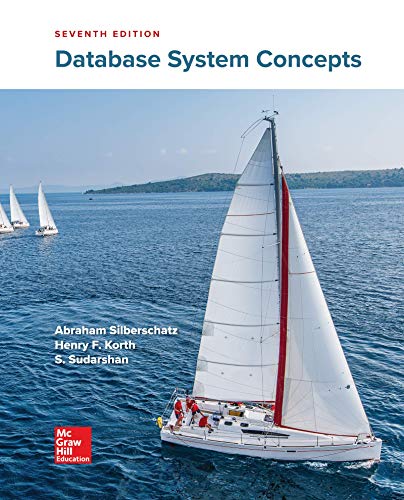
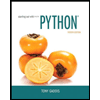
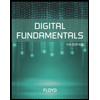
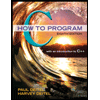
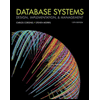
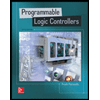