Trying this again since part of my question keeps disappearing Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts
Trying this again since part of my question keeps disappearing
Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.
Ex. if the input is:
4 Kale Lettuce Carrots Peanuts
where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list.
The output is:
Kale Lettuce Carrots Peanuts
public class ItemNode {
private String item;
private ItemNode nextNodeRef; // Reference to the next node
public ItemNode() {
item = "";
nextNodeRef = null;
}
// Constructor
public ItemNode(String itemInit) {
this.item = itemInit;
this.nextNodeRef = null;
}
// Constructor
public ItemNode(String itemInit, ItemNode nextLoc) {
this.item = itemInit;
this.nextNodeRef = nextLoc;
}
// Insert node after this node.
public void insertAfter(ItemNode nodeLoc) {
ItemNode tmpNext;
tmpNext = this.nextNodeRef;
this.nextNodeRef = nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
// TODO: Define insertAtEnd() method that inserts a node
// to the end of the linked list
// Get location pointed by nextNodeRef
public ItemNode getNext() {
return this.nextNodeRef;
}
public void printNodeData() {
System.out.println(this.item);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

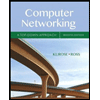
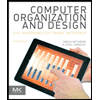
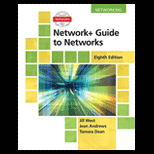
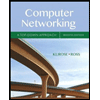
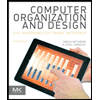
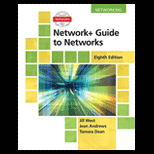
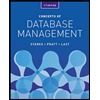
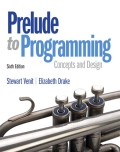
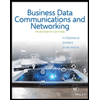