Given main.py and a PersonNode class, complete the PersonList class by writing find_first() and find_last() methods at the end of the PersonList.py file. The find_first() method should find the first occurrence of an age value in the linked list and return the corresponding node. Similarly, the find_last() method should find the last occurrence of the age value in the linked list and return the corresponding node. For both methods, if the age value is not found, None should be returned. The program will replace the name value of each found node with a new name. Ex. If the input is: Alex 23 Tom 41 Michelle 34 Vicky 23 -1 23 Connor 34 Michela main.py(can not edit) from PersonNode import PersonNode from PersonList import PersonList if __name__ == "__main__": person_list = PersonList() # Read input lines until encountering '-1' input_line = input() while input_line != '-1': split_input = input_line.split(' ') name = split_input[0] age = int(split_input[1]) new_node = PersonNode(name, age) person_list.append(new_node) input_line = input() print('List before replacing:') person_list.print_person_list() # Find first find_age = int(input()) found_first = person_list.find_first(find_age) if found_first != None: replace_name = input() found_first.name = replace_name print() print('List after replacing the first occurrence of %s:' % find_age) person_list.print_person_list() else: print('Age value not found in the list.') # Find last find_age = int(input()) found_last = person_list.find_last(find_age) if found_last != None: replace_name = input() found_last.name = replace_name print() print('List after replacing the last occurrence of %s:' % find_age) person_list.print_person_list() else: print('Age value not found in the list.') PersonList.py(need to edit) class PersonList: def __init__(self): self.head = None self.tail = None def append(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: self.tail.next = new_node new_node.prev = self.tail self.tail = new_node def prepend(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: new_node.next = self.head self.head.prev = new_node self.head = new_node def insert_after(self, current_node, new_node): if self.head is None: self.head = new_node self.tail = new_node elif current_node is self.tail: self.tail.next = new_node new_node.prev = self.tail self.tail = new_node else: successor_node = current_node.next new_node.next = successor_node new_node.prev = current_node current_node.next = new_node successor_node.prev = new_node def remove(self, current_node): successor_node = current_node.next predecessor_node = current_node.prev if successor_node is not None: successor_node.prev = predecessor_node if predecessor_node is not None: predecessor_node.next = successor_node if current_node is self.head: self.head = successor_node if current_node is self.tail: self.tail = predecessor_node def print_person_list(self): current_node = self.head while current_node != None: current_node.print_node_data() current_node = current_node.next def find_first(self, age): # TODO: Write findFirst() method # Find the node with the first occurrence of the age # Start with the headNode and traverse forward def find_last(self, age): # TODO: Write findLast() method # Find the node with the last occurrence of the age # Start with the tailNode and traverse backward PersonNode.py(can not edit) class PersonNode: def __init__(self, name = '', age = 0, prev = None, next = None): self.name = name self.age = age self.next = next self.prev = prev def print_node_data(self): print("%s, %d" % (self.name, self.age))
Given main.py and a PersonNode class, complete the PersonList class by writing find_first() and find_last() methods at the end of the PersonList.py file. The find_first() method should find the first occurrence of an age value in the linked list and return the corresponding node. Similarly, the find_last() method should find the last occurrence of the age value in the linked list and return the corresponding node. For both methods, if the age value is not found, None should be returned. The program will replace the name value of each found node with a new name.
Ex. If the input is:
Alex 23 Tom 41 Michelle 34 Vicky 23 -1 23 Connor 34 Michela
main.py(can not edit)
from PersonNode import PersonNode
from PersonList import PersonList
if __name__ == "__main__":
person_list = PersonList()
# Read input lines until encountering '-1'
input_line = input()
while input_line != '-1':
split_input = input_line.split(' ')
name = split_input[0]
age = int(split_input[1])
new_node = PersonNode(name, age)
person_list.append(new_node)
input_line = input()
print('List before replacing:')
person_list.print_person_list()
# Find first
find_age = int(input())
found_first = person_list.find_first(find_age)
if found_first != None:
replace_name = input()
found_first.name = replace_name
print()
print('List after replacing the first occurrence of %s:' % find_age)
person_list.print_person_list()
else:
print('Age value not found in the list.')
# Find last
find_age = int(input())
found_last = person_list.find_last(find_age)
if found_last != None:
replace_name = input()
found_last.name = replace_name
print()
print('List after replacing the last occurrence of %s:' % find_age)
person_list.print_person_list()
else:
print('Age value not found in the list.')
PersonList.py(need to edit)
class PersonList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
new_node.prev = self.tail
self.tail = new_node
def prepend(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
new_node.next = self.head
self.head.prev = new_node
self.head = new_node
def insert_after(self, current_node, new_node):
if self.head is None:
self.head = new_node
self.tail = new_node
elif current_node is self.tail:
self.tail.next = new_node
new_node.prev = self.tail
self.tail = new_node
else:
successor_node = current_node.next
new_node.next = successor_node
new_node.prev = current_node
current_node.next = new_node
successor_node.prev = new_node
def remove(self, current_node):
successor_node = current_node.next
predecessor_node = current_node.prev
if successor_node is not None:
successor_node.prev = predecessor_node
if predecessor_node is not None:
predecessor_node.next = successor_node
if current_node is self.head:
self.head = successor_node
if current_node is self.tail:
self.tail = predecessor_node
def print_person_list(self):
current_node = self.head
while current_node != None:
current_node.print_node_data()
current_node = current_node.next
def find_first(self, age):
# TODO: Write findFirst() method
# Find the node with the first occurrence of the age
# Start with the headNode and traverse forward
def find_last(self, age):
# TODO: Write findLast() method
# Find the node with the last occurrence of the age
# Start with the tailNode and traverse backward
PersonNode.py(can not edit)
class PersonNode:
def __init__(self, name = '', age = 0, prev = None, next = None):
self.name = name
self.age = age
self.next = next
self.prev = prev
def print_node_data(self):
print("%s, %d" % (self.name, self.age))

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

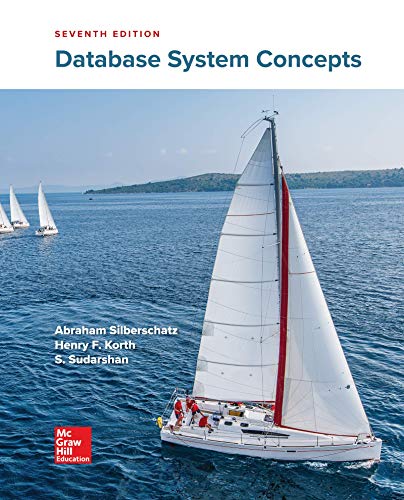
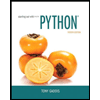
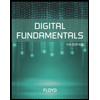
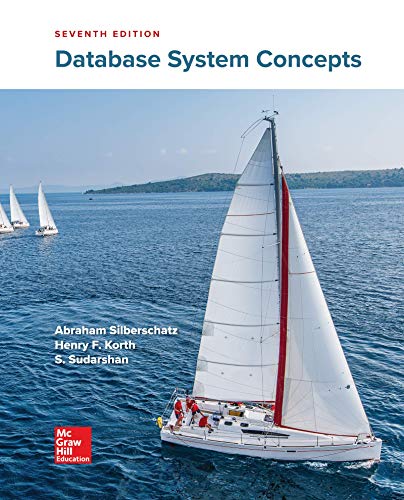
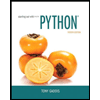
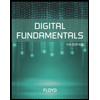
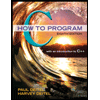
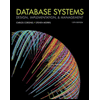
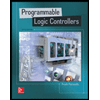