from time import sleep from os import system class Node: def__init__(self, data): self.data=data self.next=None class Stack: def__init__(self): self.top=None defpush(self, data): new_node=Node(data) ifself.topisNone: self.top=new_node else: new_node.next=self.top self.top=new_node defpop(self): ifself.topisNone: returnNone data=self.top.data self.top=self.top.next returndata def dfs(maze, start, end): rows=len(maze) cols=len(maze[0]) visited= [[Nonefor_inrange(cols)] for_inrange(rows)] stack=Stack() stack.push(start) visited[start[0]][start[1]] =start whilestack.top: current=stack.pop() ifcurrent==end: current=visited[current[0]][current[1]] # Retrocede un paso desde el final whilecurrent!=start: ifcurrent!=end: # No reemplace la meta "B" con un punto maze[current[0]][current[1]] ='.' current=visited[current[0]][current[1]] print('\n'.join(''.join(row) forrowinmaze)) # Imprime el laberinto final returnTrue# Termina la ejecución tan pronto como se encuentra el estado objetivo row, col=current fordr, dcin [(1, 0), (-1, 0), (0, 1), (0, -1)]: new_row, new_col=row+dr, col+dc if (0<=new_row<rowsand0<=new_col<colsand visited[new_row][new_col] isNoneand maze[new_row][new_col] !='#'): stack.push((new_row, new_col)) visited[new_row][new_col] =current if (new_row, new_col) !=end: # No reemplace la meta "B" con un punto maze[new_row][new_col] ='.' print('\n'.join(''.join(row) forrowinmaze)) # Imprime el laberinto sleep(0.5) # Pausa por un segundo system("clear") returnFalse def read_maze_from_file(filename): maze= [] start=end=None withopen(filename, 'r') asfile: fori, lineinenumerate(file): row=list(line.rstrip().replace('\t', ' ')) if'A'inrow: start= (i, row.index('A')) if'B'inrow: end= (i, row.index('B')) maze.append(row) returnmaze, start, end maze, start, end = read_maze_from_file('maze22.txt') if dfs(maze, start, end): print("Path found from start to end") Please stop inserting points in the next maze, which is a txt file, when you find the letter B. maze22.txt ### ########## ################### # ## #### # # # ## ################### # # # ## # # # ###################### # # # ## ## # # # ## # ## ### ## ######### # # ## # # ##B# # # ## # ## ################ # # #### ## #### # # #### ############## ## # # # #### ## # # # ####### ######## ####### # # ####### #### # #A ######################
from time import sleep from os import system class Node: def__init__(self, data): self.data=data self.next=None class Stack: def__init__(self): self.top=None defpush(self, data): new_node=Node(data) ifself.topisNone: self.top=new_node else: new_node.next=self.top self.top=new_node defpop(self): ifself.topisNone: returnNone data=self.top.data self.top=self.top.next returndata def dfs(maze, start, end): rows=len(maze) cols=len(maze[0]) visited= [[Nonefor_inrange(cols)] for_inrange(rows)] stack=Stack() stack.push(start) visited[start[0]][start[1]] =start whilestack.top: current=stack.pop() ifcurrent==end: current=visited[current[0]][current[1]] # Retrocede un paso desde el final whilecurrent!=start: ifcurrent!=end: # No reemplace la meta "B" con un punto maze[current[0]][current[1]] ='.' current=visited[current[0]][current[1]] print('\n'.join(''.join(row) forrowinmaze)) # Imprime el laberinto final returnTrue# Termina la ejecución tan pronto como se encuentra el estado objetivo row, col=current fordr, dcin [(1, 0), (-1, 0), (0, 1), (0, -1)]: new_row, new_col=row+dr, col+dc if (0<=new_row<rowsand0<=new_col<colsand visited[new_row][new_col] isNoneand maze[new_row][new_col] !='#'): stack.push((new_row, new_col)) visited[new_row][new_col] =current if (new_row, new_col) !=end: # No reemplace la meta "B" con un punto maze[new_row][new_col] ='.' print('\n'.join(''.join(row) forrowinmaze)) # Imprime el laberinto sleep(0.5) # Pausa por un segundo system("clear") returnFalse def read_maze_from_file(filename): maze= [] start=end=None withopen(filename, 'r') asfile: fori, lineinenumerate(file): row=list(line.rstrip().replace('\t', ' ')) if'A'inrow: start= (i, row.index('A')) if'B'inrow: end= (i, row.index('B')) maze.append(row) returnmaze, start, end maze, start, end = read_maze_from_file('maze22.txt') if dfs(maze, start, end): print("Path found from start to end") Please stop inserting points in the next maze, which is a txt file, when you find the letter B. maze22.txt ### ########## ################### # ## #### # # # ## ################### # # # ## # # # ###################### # # # ## ## # # # ## # ## ### ## ######### # # ## # # ##B# # # ## # ## ################ # # #### ## #### # # #### ############## ## # # # #### ## # # # ####### ######## ####### # # ####### #### # #A ######################
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
from time import sleep
from os import system
class Node:
def__init__(self, data):
self.data=data
self.next=None
class Stack:
def__init__(self):
self.top=None
defpush(self, data):
new_node=Node(data)
ifself.topisNone:
self.top=new_node
else:
new_node.next=self.top
self.top=new_node
defpop(self):
ifself.topisNone:
returnNone
data=self.top.data
self.top=self.top.next
returndata
def dfs(maze, start, end):
rows=len(maze)
cols=len(maze[0])
visited= [[Nonefor_inrange(cols)] for_inrange(rows)]
stack=Stack()
stack.push(start)
visited[start[0]][start[1]] =start
whilestack.top:
current=stack.pop()
ifcurrent==end:
current=visited[current[0]][current[1]] # Retrocede un paso desde el final
whilecurrent!=start:
ifcurrent!=end: # No reemplace la meta "B" con un punto
maze[current[0]][current[1]] ='.'
current=visited[current[0]][current[1]]
print('\n'.join(''.join(row) forrowinmaze)) # Imprime el laberinto final
returnTrue# Termina la ejecución tan pronto como se encuentra el estado objetivo
row, col=current
fordr, dcin [(1, 0), (-1, 0), (0, 1), (0, -1)]:
new_row, new_col=row+dr, col+dc
if (0<=new_row<rowsand0<=new_col<colsand
visited[new_row][new_col] isNoneand
maze[new_row][new_col] !='#'):
stack.push((new_row, new_col))
visited[new_row][new_col] =current
if (new_row, new_col) !=end: # No reemplace la meta "B" con un punto
maze[new_row][new_col] ='.'
print('\n'.join(''.join(row) forrowinmaze)) # Imprime el laberinto
sleep(0.5) # Pausa por un segundo
system("clear")
returnFalse
def read_maze_from_file(filename):
maze= []
start=end=None
withopen(filename, 'r') asfile:
fori, lineinenumerate(file):
row=list(line.rstrip().replace('\t', ' '))
if'A'inrow:
start= (i, row.index('A'))
if'B'inrow:
end= (i, row.index('B'))
maze.append(row)
returnmaze, start, end
maze, start, end = read_maze_from_file('maze22.txt')
if dfs(maze, start, end):
print("Path found from start to end")
Please stop inserting points in the next maze, which is a txt file, when you find the letter B.
maze22.txt
### #########
# ################### # #
# #### # # # #
# ################### # # # #
# # # # #
##################### # # # #
# ## # # # #
# # ## ### ## ######### # # #
# # # ##B# # # #
# # ## ################ # # #
### ## #### # # #
### ############## ## # # # #
### ## # # # #
###### ######## ####### # # #
###### #### # #
A ######################
# ################### # #
# #### # # # #
# ################### # # # #
# # # # #
##################### # # # #
# ## # # # #
# # ## ### ## ######### # # #
# # # ##B# # # #
# # ## ################ # # #
### ## #### # # #
### ############## ## # # # #
### ## # # # #
###### ######## ####### # # #
###### #### # #
A ######################
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
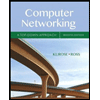
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
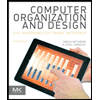
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
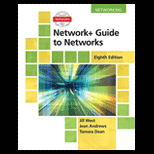
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
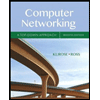
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
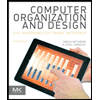
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
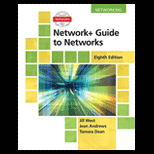
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
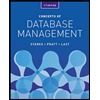
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
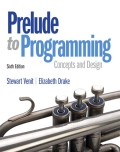
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
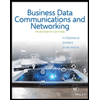
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY