Students can graduate with honors if their GPA is at or above 3.0. Complete the Course class by implementing the count_honors() instance method, which returns the number of students with a GPA at or above 3.0. The file main.py contains: • The main function for testing the program. • Class Course represents a course, which contains a list of Student objects as a course roster. (Type your code in here.) • Class Student represents a classroom student, which has three attributes: first name, last name, and GPA. Hint: Refer to the Student class to explore the available instance methods that can be used for implementing the count_honors() method. Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Cabot 3.2 Brenda Stern 2.9 Lynda Robison 3.6 Jane Flynn 1.8. the output Honors count: 2
Students can graduate with honors if their GPA is at or above 3.0. Complete the Course class by implementing the count_honors() instance method, which returns the number of students with a GPA at or above 3.0. The file main.py contains: • The main function for testing the program. • Class Course represents a course, which contains a list of Student objects as a course roster. (Type your code in here.) • Class Student represents a classroom student, which has three attributes: first name, last name, and GPA. Hint: Refer to the Student class to explore the available instance methods that can be used for implementing the count_honors() method. Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Cabot 3.2 Brenda Stern 2.9 Lynda Robison 3.6 Jane Flynn 1.8. the output Honors count: 2
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 7PE
Related questions
Question
Looking for output HONORS COUNT : 2

Transcribed Image Text:14.26 LAB: Count honors
Students can graduate with honors if their GPA is at or above 3.0. Complete the Course class by implementing the count_honors() instance
method, which returns the number of students with a GPA at or above 3.0.
The file main.py contains:
• The main function for testing the program.
• Class Course represents a course, which contains a list of Student objects as a course roster. (Type your code in here.)
• Class Student represents a classroom student, which has three attributes: first name, last name, and GPA.
Hint: Refer to the Student class to explore the available instance methods that can be used for implementing the count_honors() method.
Note: For testing purposes, different student values will be used.
Ex. For the following students:
Henry Cabot 3.2
Brenda Stern 2.9
Lynda Robison 3.6
Jane Flynn 1.8
the output is:
Honors count: 2

Transcribed Image Text:class Student:
definit__(self, first, last, gpa):
self.first = first # first name
self.last = last
self.gpa = gpa
def get_gpa(self):
return self.gpa
def get_last(self):
return self.last
class Course:
definit__(self):
self.roster
def add_student(self, student):
def course_size(self):
self.roster.append(student)
#last name
#grade point average
# Type your code here
#list of Student objects
return len(self.roster)
if __name__
course Course()
"__main__":
course.add_student (Student('Henry', 'Cabot', 3.2))
course.add_student (Student('Brenda', 'Stern', 2.9))
course.add_student (Student('Lynda', 'Robison', 3.6))
course.add_student (Student('Jane', 'Flynn', 1.8))
honors_count = course.count_honors()
print(f'Honors count: { honors_count }')
Expert Solution

Step 1
The Python code is given below with output screenshot
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
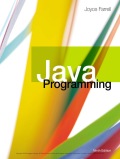
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
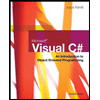
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
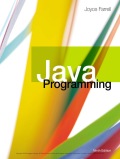
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
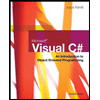
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage