Fix the code so it can run and not have any internal errors used java import java.util.Scanner; class PiggyBank{ private double total=0.0; void AddNickel(int num){ total = total + num*0.05; } void AddDime(int num){ total = total + num*0.10; } void AddQuarter(int num){ total = total + num*0.25; } void ShowTotal(){ System.out.println("YOUR BALANCE ACCOUNT IS $ "+total); } void Withdraw(double mul){ if(mul<=total) total = total-mul; else System.out.println("NOT ENOUGH BALANCE TO WITHDRAW !!"); } } class Main{ public static void main(String arg[]){ int choice1=0,mul=0; double mul2=0.0; Scanner in = new Scanner(System.in); PiggyBank pb = new PiggyBank(); do{ System.out.println(""); System.out.println(" 1. Show total in bank "); System.out.println(" 2. Add a nickel"); System.out.println(" 3. Add a dime"); System.out.println(" 4. Add a Quater"); System.out.println(" 5. Tale a money out of the bank"); System.out.print(" Enter 0 to quit. "); System.out.print(" Enter Your Choice: "); choice1=in.nextInt(); switch(choice1) { case 1: System.out.println(""); pb.ShowTotal(); break; case 2: System.out.println(""); System.out.print("Enter Number of Nickel to add : "); mul=in.nextInt(); pb.AddNickel(mul); break; case 3: System.out.println(""); System.out.print("Enter Number of Dime to add : "); mul=in.nextInt(); pb.AddDime(mul); break; case 4: System.out.println(""); System.out.print("Enter Number of quarter to add : "); mul=in.nextInt(); pb.AddQuarter(mul); break; case 5: System.out.println(""); System.out.print("Enter Amount to withdraw : "); mul2=in.nextDouble(); pb.Withdraw(mul); break; case 0: System.out.println(""); System.out.print("END PROGRAM !! "); break; default: System.out.println("Wronge Choice,try again !"); } }while(choice1!=0); } }
Fix the code so it can run and not have any internal errors
used java
import java.util.Scanner;
class PiggyBank{
private double total=0.0;
void AddNickel(int num){
total = total + num*0.05;
}
void AddDime(int num){
total = total + num*0.10;
}
void AddQuarter(int num){
total = total + num*0.25;
}
void ShowTotal(){
System.out.println("YOUR BALANCE ACCOUNT IS $ "+total);
}
void Withdraw(double mul){
if(mul<=total)
total = total-mul;
else
System.out.println("NOT ENOUGH BALANCE TO WITHDRAW !!");
}
}
class Main{
public static void main(String arg[]){
int choice1=0,mul=0;
double mul2=0.0;
Scanner in = new Scanner(System.in);
PiggyBank pb = new PiggyBank();
do{
System.out.println("");
System.out.println(" 1. Show total in bank ");
System.out.println(" 2. Add a nickel");
System.out.println(" 3. Add a dime");
System.out.println(" 4. Add a Quater");
System.out.println(" 5. Tale a money out of the bank");
System.out.print(" Enter 0 to quit. ");
System.out.print(" Enter Your Choice: ");
choice1=in.nextInt();
switch(choice1)
{
case 1:
System.out.println("");
pb.ShowTotal();
break;
case 2:
System.out.println("");
System.out.print("Enter Number of Nickel to add : ");
mul=in.nextInt();
pb.AddNickel(mul);
break;
case 3:
System.out.println("");
System.out.print("Enter Number of Dime to add : ");
mul=in.nextInt();
pb.AddDime(mul);
break;
case 4:
System.out.println("");
System.out.print("Enter Number of quarter to add : ");
mul=in.nextInt();
pb.AddQuarter(mul);
break;
case 5:
System.out.println("");
System.out.print("Enter Amount to withdraw : ");
mul2=in.nextDouble();
pb.Withdraw(mul);
break;
case 0:
System.out.println("");
System.out.print("END PROGRAM !! ");
break;
default:
System.out.println("Wronge Choice,try again !");
}
}while(choice1!=0);
}
}

Step by step
Solved in 2 steps with 1 images

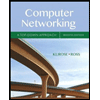
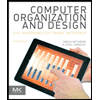
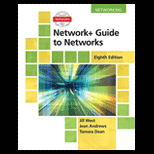
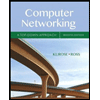
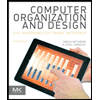
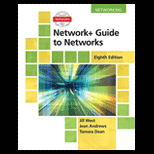
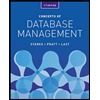
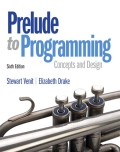
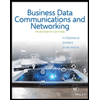