Fill the blanks for C. /*Copy Strings BackwardInput String1: Hello World*/ #include #define str_len __1__ //str_len = hello + space + world + null char LEN __2__ print_string(__3__ str[__4__]); __5__ copy_string(__6__ s1[__7__], __8__ s2[__9__]); int main() { __10__ str1[]= "Hello World"; __11__ str2[__12__]; //loop and copy each character over // until the array reaches null character copy_string(__13__, __14__); //printing back by calling function print_string printf("String1: "); print_string(__15__); printf("\nString2: "); print_string(__16__); } /*Copy one string to another by looping backward. Need 2 index variables to keep track of each string. For ex: string1 = "hello", len = 6 (1 for null character) string2[0] = string1[4] // letter 'o' string2[1] = string2[3] // letter 'l' Notice the index value compare to the len.*/ __17__ copy_string(__18__ s1[__19__], __20__ s2[__21__]) { int idx1, idx2 = 0; //this is the index counter for the 2 strings s1 and s2 /*be careful here. We'll loop from the end of str1 to get letter 'd' normally, for array, last character is size-1, but for string, the last index is the null character, not the last character*/ for(idx1= str_len - __22__; idx1 >= __23__ ; idx1 __24__) { /*Copy each char from string s1 to string s2*/ __25__[__26__] = __27__[__28__]; /*idx1 for s1 is updated with for loop update idx2 to save in the next location of s2.*/ idx2++; } //After reading all characters,need to add something in the last //index position to let the program knows the string has ended. s2[__29__] = '__30__'; } /*Printing all letter until the null character is reached. printf("%s", str) would work, but we are doing this way to make sure we understand how string works. Loop through all character until it reaches null character*/ __31__ print_string(__32__ str[str_len]) { for(int i = 0; str[i] != __33__; i++) printf("%c", __34__); }
Fill the blanks for C.
/*Copy Strings BackwardInput
String1: Hello World*/
#include<stdio.h>
#define str_len __1__ //str_len = hello + space + world + null char LEN
__2__ print_string(__3__ str[__4__]);
__5__ copy_string(__6__ s1[__7__], __8__ s2[__9__]);
int main()
{
__10__ str1[]= "Hello World";
__11__ str2[__12__];
//loop and copy each character over
// until the array reaches null character
copy_string(__13__, __14__);
//printing back by calling function print_string
printf("String1: ");
print_string(__15__);
printf("\nString2: ");
print_string(__16__);
}
/*Copy one string to another by looping backward.
Need 2 index variables to keep track of each string.
For ex: string1 = "hello", len = 6 (1 for null character)
string2[0] = string1[4] // letter 'o'
string2[1] = string2[3] // letter 'l'
Notice the index value compare to the len.*/
__17__ copy_string(__18__ s1[__19__], __20__ s2[__21__])
{
int idx1, idx2 = 0; //this is the index counter for the 2 strings s1 and s2
/*be careful here. We'll loop from the end of str1 to get letter 'd'
normally, for array, last character is size-1, but for string,
the last index is the null character, not the last character*/
for(idx1= str_len - __22__; idx1 >= __23__ ; idx1 __24__)
{
/*Copy each char from string s1 to string s2*/
__25__[__26__] = __27__[__28__];
/*idx1 for s1 is updated with for loop
update idx2 to save in the next location of s2.*/
idx2++;
}
//After reading all characters,need to add something in the last
//index position to let the program knows the string has ended.
s2[__29__] = '__30__';
}
/*Printing all letter until the null character is reached.
printf("%s", str) would work, but we are doing this way to make sure we understand how string works.
Loop through all character until it reaches null character*/
__31__ print_string(__32__ str[str_len])
{
for(int i = 0; str[i] != __33__; i++)
printf("%c", __34__);
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

After using this. s2[idx2] = s1 [idx1].
It is still not working. Would you please run the code before posting the answer? Thank you.
This code does not print anything. Would you please check the code again. Thank you.
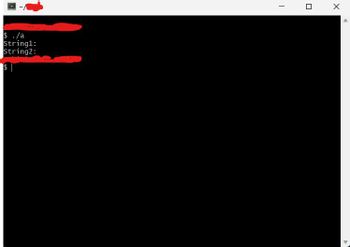
Hi, I asked for the code which Copy Strings BackwardInput. Please refer below instruction.
This task studys strings’ basic. We’ll initialize a string (I’ll use “Hello World”). Then, create a
second string and copy the first string over, but backwards, using a loop. Print both strings.
For example: string 1 is “Hello World”, string 2 would be “dlroW olleH”.
Hint:
• To print or scan a string, null character will be in the last spot of your string.
• When creating the second string, since you don’t initialize it, you’ll need to give a size.
• We’ll create a constant str_len to be the size of string “Hello World”
o Need to count all the spaces in between and one slot for the null character
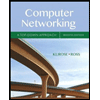
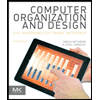
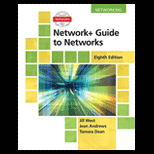
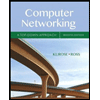
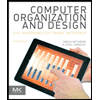
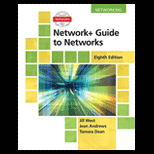
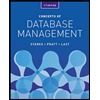
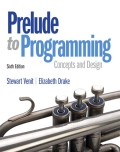
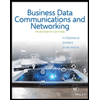