Fancy Integer ArrayList (ONLY NEED HELP WITH WRITING TEST CASES) For example, we have defined a “testRemoveInt” method for you (see below) which tests the “remove” method. Pass the tests provided then write additional tests where it says “// TODO”. You’ll do this for each unit test method in the program. import static org.junit.jupiter.api.Assertions.*; import java.util.ArrayList; import org.junit.jupiter.api.Test; class CustomIntegerArrayListTest { @Test void testSpliceIntInt() { CustomIntegerArrayList arr1 = new CustomIntegerArrayList(); arr1.add(2); arr1.add(3); arr1.add(4); arr1.add(5); arr1.add(6); arr1.splice(3, 2); ArrayList lst1 = new ArrayList(); lst1.add(2); lst1.add(3); lst1.add(4); assertEquals((int) lst1.get(0), (int) arr1.get(0)); assertEquals((int) lst1.get(1), (int) arr1.get(1)); assertEquals((int) lst1.get(2), (int) arr1.get(2)); // TODO write at least 3 additional test cases } @Test void testSpliceIntIntIntArray() { CustomIntegerArrayList arr1 = new CustomIntegerArrayList(); arr1.add(2); arr1.add(3); arr1.add(4); arr1.add(5); arr1.add(6); arr1.splice(3, 1, new int[] { 4, 5 }); ArrayList lst1 = new ArrayList(); lst1.add(2); lst1.add(3); lst1.add(4); lst1.add(4); lst1.add(5); lst1.add(6); assertEquals(lst1, arr1.getArrayList()); // TODO write at least 3 additional test cases
Fancy Integer ArrayList (ONLY NEED HELP WITH WRITING TEST CASES) For example, we have defined a “testRemoveInt” method for you (see below) which tests the “remove” method. Pass the tests provided then write additional tests where it says “// TODO”. You’ll do this for each unit test method in the program. import static org.junit.jupiter.api.Assertions.*; import java.util.ArrayList; import org.junit.jupiter.api.Test; class CustomIntegerArrayListTest { @Test void testSpliceIntInt() { CustomIntegerArrayList arr1 = new CustomIntegerArrayList(); arr1.add(2); arr1.add(3); arr1.add(4); arr1.add(5); arr1.add(6); arr1.splice(3, 2); ArrayList lst1 = new ArrayList(); lst1.add(2); lst1.add(3); lst1.add(4); assertEquals((int) lst1.get(0), (int) arr1.get(0)); assertEquals((int) lst1.get(1), (int) arr1.get(1)); assertEquals((int) lst1.get(2), (int) arr1.get(2)); // TODO write at least 3 additional test cases } @Test void testSpliceIntIntIntArray() { CustomIntegerArrayList arr1 = new CustomIntegerArrayList(); arr1.add(2); arr1.add(3); arr1.add(4); arr1.add(5); arr1.add(6); arr1.splice(3, 1, new int[] { 4, 5 }); ArrayList lst1 = new ArrayList(); lst1.add(2); lst1.add(3); lst1.add(4); lst1.add(4); lst1.add(5); lst1.add(6); assertEquals(lst1, arr1.getArrayList()); // TODO write at least 3 additional test cases
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Fancy Integer ArrayList (ONLY NEED HELP WITH WRITING TEST CASES)
For example, we have defined a “testRemoveInt” method for you (see below) which tests the “remove” method. Pass the tests provided then write additional tests where it says “// TODO”. You’ll do this for each unit test method in the program.
import static org.junit.jupiter.api.Assertions.*;
import java.util.ArrayList;
import org.junit.jupiter.api.Test;
class CustomIntegerArrayListTest {
@Test
void testSpliceIntInt() {
CustomIntegerArrayList arr1 = new CustomIntegerArrayList();
arr1.add(2);
arr1.add(3);
arr1.add(4);
arr1.add(5);
arr1.add(6);
arr1.splice(3, 2);
ArrayList<Integer> lst1 = new ArrayList<Integer>();
lst1.add(2);
lst1.add(3);
lst1.add(4);
assertEquals((int) lst1.get(0), (int) arr1.get(0));
assertEquals((int) lst1.get(1), (int) arr1.get(1));
assertEquals((int) lst1.get(2), (int) arr1.get(2));
// TODO write at least 3 additional test cases
}
@Test
void testSpliceIntIntIntArray() {
CustomIntegerArrayList arr1 = new CustomIntegerArrayList();
arr1.add(2);
arr1.add(3);
arr1.add(4);
arr1.add(5);
arr1.add(6);
arr1.splice(3, 1, new int[] { 4, 5 });
ArrayList<Integer> lst1 = new ArrayList<Integer>();
lst1.add(2);
lst1.add(3);
lst1.add(4);
lst1.add(4);
lst1.add(5);
lst1.add(6);
assertEquals(lst1, arr1.getArrayList());
// TODO write at least 3 additional test cases

Transcribed Image Text:Homework 4: Fancy Integer ArrayList
This assignment is designed to give you practice writing code and applying lessons and
topics for the current module.
This homework deals with the following topics:
ArrayLists
Overloading
• Unit Testing
The Assignment
In this assignment, you will implement a class called Custom IntegerArrayList. This class
represents a fancy ArrayList that stores integers and supports additional operations not
included in Java's built-in ArrayList methods.
For example, the Custom IntegerArrayList class has a "splice" method which removes a
specified number of elements from the Custom IntegerArrayList, starting at a given index. For a
CustomIntegerArrayList that includes: 1, 2, 3, 4, and 5, calling splice(1, 2) will remove 2 items
starting at index 1. This will remove 2 and 3 (the 2nd and 3rd items).
The CustomIntegerArrayList class has 2 different (overloaded) constructors. (Remember, an
overloaded constructor is a constructor that has the same name, but a different number, type,
or sequence of parameters, as another constructor in the class.) Having 2 different
constructors means you can create an instance of the Custom IntegerArrayList class in 2
different ways, depending on which constructor you call. Both constructors have been
implemented for you. (See below)
Internally, the Custom IntegerArrayList class uses a private ArrayList variable named "arr" to
store its integer elements. The "CustomIntegerArrayList()" constructor initializes the ArrayList
variable "arr" as an empty ArrayList and the "CustomIntegerArrayList(ArrayList<Integer> arr)"
constructor initializes the ArrayList variable "arr" with the elements in the given ArrayList.
(Again, see below)
/**
* Fancy ArrayList that stores integers and supports additional
operations not included in Java's built-in ArrayList methods.
*/
![public class CustomIntegerArrayList {
// instance variables
/**
* Internal ArrayList of elements.
*/
private ArrayList<Integer> arr;
// constructors
/**
* Creates a new empty Custom IntegerArrayList.
*/
public Custom IntegerArrayList ()
}
this.arr = new ArrayList<Integer> ();
/**
* Creates a new Custom IntegerArrayList with the elements in
the given ArrayList.
*
@param arr with elements for the Custom IntegerArrayList
*/
public Custom IntegerArrayList (ArrayList<Integer> arr) {
this.arr = new ArrayList<Integer> (arr);
}
There are 8 (mostly overloaded) methods that need to be implemented in the
CustomIntegerArrayList class. (Again, an overloaded method is a method that has the same
name, but a different number, type, or sequence of parameters, as another method in the same
class.)
getArrayList() - Returns the elements.
•
get(int index) - Returns the element at the specified index from the elements.
add (int element) - Appends the given element to the end of the elements.
•
add (int index, int element) - Inserts the given element at the specified index.
remove(int index) - Removes the element at the specified index.
•
remove(int num, int element) - Removes the specified number of the given element
from all elements.
•
splice (int index, int num) - Removes the specified number of elements from all
elements, starting at the given index.
• splice (int index, int num, int[] otherArray) - Removes the specified number of elements
from all elements, starting at the given index, and inserts new elements in the given
array at the given index.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Feda5bfed-6ef3-495e-84a7-318d55d37402%2F568adc8c-f88c-411a-8566-02705798111f%2F7nof0jj_processed.png&w=3840&q=75)
Transcribed Image Text:public class CustomIntegerArrayList {
// instance variables
/**
* Internal ArrayList of elements.
*/
private ArrayList<Integer> arr;
// constructors
/**
* Creates a new empty Custom IntegerArrayList.
*/
public Custom IntegerArrayList ()
}
this.arr = new ArrayList<Integer> ();
/**
* Creates a new Custom IntegerArrayList with the elements in
the given ArrayList.
*
@param arr with elements for the Custom IntegerArrayList
*/
public Custom IntegerArrayList (ArrayList<Integer> arr) {
this.arr = new ArrayList<Integer> (arr);
}
There are 8 (mostly overloaded) methods that need to be implemented in the
CustomIntegerArrayList class. (Again, an overloaded method is a method that has the same
name, but a different number, type, or sequence of parameters, as another method in the same
class.)
getArrayList() - Returns the elements.
•
get(int index) - Returns the element at the specified index from the elements.
add (int element) - Appends the given element to the end of the elements.
•
add (int index, int element) - Inserts the given element at the specified index.
remove(int index) - Removes the element at the specified index.
•
remove(int num, int element) - Removes the specified number of the given element
from all elements.
•
splice (int index, int num) - Removes the specified number of elements from all
elements, starting at the given index.
• splice (int index, int num, int[] otherArray) - Removes the specified number of elements
from all elements, starting at the given index, and inserts new elements in the given
array at the given index.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
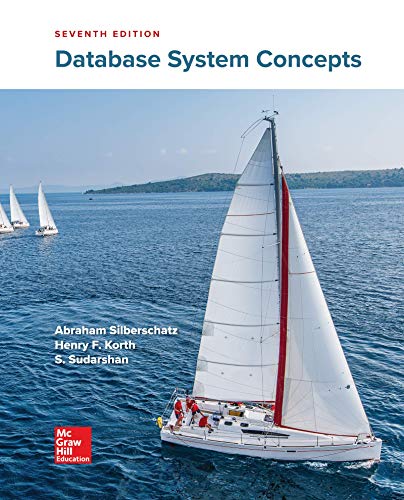
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
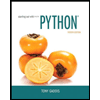
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
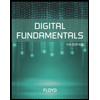
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
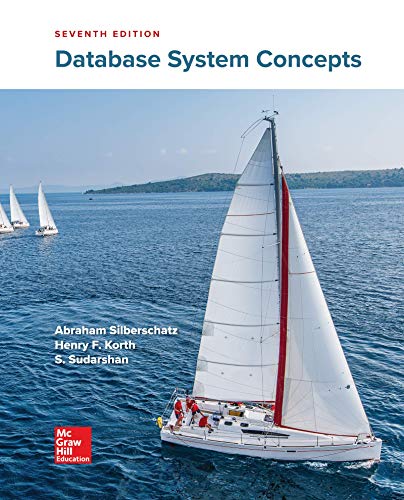
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
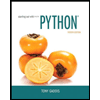
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
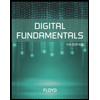
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
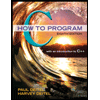
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
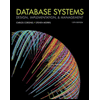
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
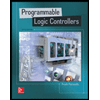
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education