Example 2: create a Chess Piece class that represents a chess piece. We'll use a getter and setter to control the position of the piece on the board and validate each move to ensure it's within the rules of chess. class Chess Piece: def __init__(self, name, color, position): self.name = name # Name of the piece (e.g., Queen, Rook) self.color = color # Color of the piece (e.g., White, Black) self._position = position # Position of the piece on the board (private) # Getter for position def get_position(self): return self._position # Setter for position with validation def set_position(self, new_position): if self.__is_valid_position (new_position): self._position = new_position print(f"{(self.name} moved to {self._position}") else: print(f"Invalid move for (self.name}") # Private method to validate position def _is_valid_position(self, position): # Check if the position is within 'a1' to 'h8' (standard chess board limits) if len(position) != 2: return False column, row = position[0], position [1] return column in 'abcdefgh' and row in '12345678' queen = ChessPiece("Queen", "White", "d1") print("Initial Position:", queen.get_position()) # Output: d1 queen.set_position("d4") print("New Position:", queen.get_position()) # Output: d4 queen.set_position("j9") # Output: Invalid move for Queen exparint mple 4: Timer apsed time, whe import time
Example 2: create a Chess Piece class that represents a chess piece. We'll use a getter and setter to control the position of the piece on the board and validate each move to ensure it's within the rules of chess. class Chess Piece: def __init__(self, name, color, position): self.name = name # Name of the piece (e.g., Queen, Rook) self.color = color # Color of the piece (e.g., White, Black) self._position = position # Position of the piece on the board (private) # Getter for position def get_position(self): return self._position # Setter for position with validation def set_position(self, new_position): if self.__is_valid_position (new_position): self._position = new_position print(f"{(self.name} moved to {self._position}") else: print(f"Invalid move for (self.name}") # Private method to validate position def _is_valid_position(self, position): # Check if the position is within 'a1' to 'h8' (standard chess board limits) if len(position) != 2: return False column, row = position[0], position [1] return column in 'abcdefgh' and row in '12345678' queen = ChessPiece("Queen", "White", "d1") print("Initial Position:", queen.get_position()) # Output: d1 queen.set_position("d4") print("New Position:", queen.get_position()) # Output: d4 queen.set_position("j9") # Output: Invalid move for Queen exparint mple 4: Timer apsed time, whe import time
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![Example 2: create a Chess Piece class that represents a chess piece. We'll use a getter and setter to control the position
of the piece on the board and validate each move to ensure it's within the rules of chess.
class Chess Piece:
def __init__(self, name, color, position):
self.name = name # Name of the piece (e.g., Queen, Rook)
self.color = color # Color of the piece (e.g., White, Black)
self._position = position # Position of the piece on the board (private)
# Getter for position
def get_position(self):
return self._position
# Setter for position with validation
def set_position(self, new_position):
if self.__is_valid_position (new_position):
self._position = new_position
print(f"{(self.name} moved to {self._position}")
else:
print(f"Invalid move for (self.name}")
# Private method to validate position
def _is_valid_position(self, position):
# Check if the position is within 'a1' to 'h8' (standard chess board limits)
if len(position) != 2:
return False
column, row = position[0], position [1]
return column in 'abcdefgh' and row in '12345678'
queen = ChessPiece("Queen", "White", "d1")
print("Initial Position:", queen.get_position())
# Output: d1
queen.set_position("d4")
print("New Position:", queen.get_position())
# Output: d4
queen.set_position("j9")
# Output: Invalid move for Queen
exparint
mple 4: Timer
apsed time, whe
import time](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fab0ba513-ff83-44e4-8162-ac01cf3c31a0%2F0ffd9f52-362b-4b4b-9a25-52174dea30ba%2Flvl0psq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Example 2: create a Chess Piece class that represents a chess piece. We'll use a getter and setter to control the position
of the piece on the board and validate each move to ensure it's within the rules of chess.
class Chess Piece:
def __init__(self, name, color, position):
self.name = name # Name of the piece (e.g., Queen, Rook)
self.color = color # Color of the piece (e.g., White, Black)
self._position = position # Position of the piece on the board (private)
# Getter for position
def get_position(self):
return self._position
# Setter for position with validation
def set_position(self, new_position):
if self.__is_valid_position (new_position):
self._position = new_position
print(f"{(self.name} moved to {self._position}")
else:
print(f"Invalid move for (self.name}")
# Private method to validate position
def _is_valid_position(self, position):
# Check if the position is within 'a1' to 'h8' (standard chess board limits)
if len(position) != 2:
return False
column, row = position[0], position [1]
return column in 'abcdefgh' and row in '12345678'
queen = ChessPiece("Queen", "White", "d1")
print("Initial Position:", queen.get_position())
# Output: d1
queen.set_position("d4")
print("New Position:", queen.get_position())
# Output: d4
queen.set_position("j9")
# Output: Invalid move for Queen
exparint
mple 4: Timer
apsed time, whe
import time
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
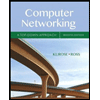
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
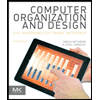
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
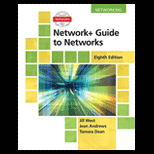
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
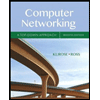
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
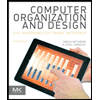
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
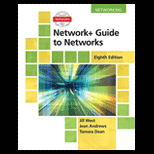
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
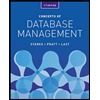
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
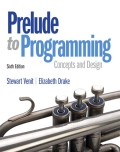
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
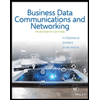
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY