Determine the output of the following program: #include using namespace std; void cdabs(int); void cdabs(float); void cdabs(double); int main(){ cdabs (3); cin.ignore(); return 0; } void cdabs(int x){ cout <« x « " is an int" « endl; } void cdabs(float x){ cout <« x « " is a float" « endl; } void cdabs(double x){ cout <« x << " is a double" <« endl;
Determine the output of the following program: #include using namespace std; void cdabs(int); void cdabs(float); void cdabs(double); int main(){ cdabs (3); cin.ignore(); return 0; } void cdabs(int x){ cout <« x « " is an int" « endl; } void cdabs(float x){ cout <« x « " is a float" « endl; } void cdabs(double x){ cout <« x << " is a double" <« endl;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Sure! Here's a transcription and explanation of the code you provided for an educational website:
---
### Understanding Function Overloading in C++
**Objective:** Determine the output of the following program.
```cpp
#include <iostream>
using namespace std;
void cdabs(int);
void cdabs(float);
void cdabs(double);
int main() {
cdabs(3);
cin.ignore();
return 0;
}
void cdabs(int x) {
cout << x << " is an int" << endl;
}
void cdabs(float x) {
cout << x << " is a float" << endl;
}
void cdabs(double x) {
cout << x << " is a double" << endl;
}
```
**Explanation:**
This C++ program demonstrates function overloading—a feature that allows multiple functions with the same name but with different parameter types.
- **Function Declarations:**
- `void cdabs(int);` - Declares a function that takes an integer parameter.
- `void cdabs(float);` - Declares a function that takes a float parameter.
- `void cdabs(double);` - Declares a function that takes a double parameter.
- **Main Function:**
- Calls the `cdabs` function with the integer argument `3`.
- **Function Definitions:**
- `cdabs(int x)` is called because the argument `3` is an integer. This function outputs: `3 is an int`.
- **Output:**
- The program output will be:
```
3 is an int
```
This illustrates how C++ selects the appropriate function to execute based on the parameter type during a function call. In this case, `cdabs(int x)` is chosen due to the integer argument.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
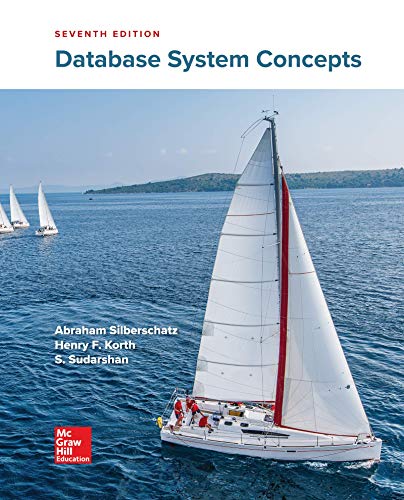
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
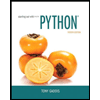
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
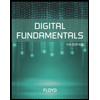
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
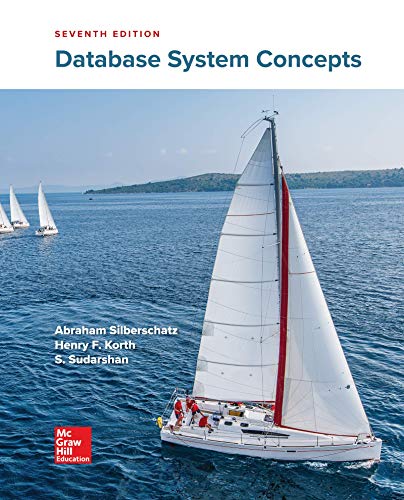
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
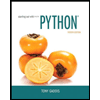
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
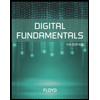
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
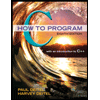
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
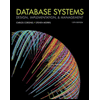
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
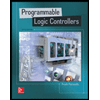
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education