Create a UML class hierarchy (three connected UML diagrams) that has all three classes you created above. Make sure each UML diagram is a one-to-one to the code, it matches the Java code exactly. Make sure that both Child Classes point to their Parent. Hint: your subclasses are siblings.
Create a UML class hierarchy (three connected UML diagrams) that has all three classes you
created above. Make sure each UML diagram is a one-to-one to the code, it matches the Java
code exactly. Make sure that both Child Classes point to their Parent.
Hint: your subclasses are siblings.
Hint: You need to create three UML diagrams for three created Java classes and connect them
using arrows, where each Child Class points to its Parent. You can either draw this by hand and
take a picture or use a tool / software of your choice.
class Desktop extends Computer {
private int width;
private int height;
public Desktop() {
super();
width = height = 0;
}
public Desktop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, int width, int height) {
super(manufacturer, diskSize, manufacturingDate, numberOfCores);
this.width = width;
this.height = height;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public void setWidth(int width) {
this.width = width;
}
public void setHeight(int height) {
this.height = height;
}
@Override
public String toString() {
return super.toString() + "\nWidth: " + width + " cm" + "\nHeight: " + height + " cm";
}
}
class Laptop extends Computer {
private double weight;
public Laptop() {
super();
weight = 0.0;
}
public Laptop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, double weight) {
super(manufacturer, diskSize, manufacturingDate, numberOfCores);
this.weight = weight;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
@Override
public String toString() {
return super.toString() + "\nWeight: " + weight + " kg";
}
}

![➡ eclipse-workspace - project2/src/project2/TestComputer.java - Eclipse IDE
File Edit Source Refactor Navigate Search Project Run Window Help
Q
- 2
C
음...
TestPrintPri...
1 package project2;
2 public class TestComputer
3 {
{
project.java
4 public static void main(String[] args)
5
6
7
8
9
10
11
12 System.out.println (cl);
13 System.out.println();
14 System.out.println (c2);
}
49°F
Clear
15 System.out.println();
16
System.out.println (c3);
17
18
19 }
20
21
#
Difference.java
//Printing the information
▬▬
▬▬▬
Table.java
//Creating 3 objects.
Computer cl = new Computer ("Dell", "1189160321024", "April 1, 2020", 2);
Computer c2 = new Computer ("Apple Inc", "269283712040", "March 31, 2020", 4);
Computer c3 = new Computer ("Microsoft", "267950430223", "june 30, 2021", 6);
Q Search
↓➡
MoreArith.java
Herjames_Pr...
Writable
a
Computer.java
Smart Insert
TestCompute... XDesktop.java
16:26: 537
Laptop.java
89
a
8:02 PM
4/3/2023
X
* * * + @ 隐旦
8
%
鼎
2
凰
@](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F467946bb-e41e-4beb-a6a7-a7fc7a246d66%2F5e95fb3d-acc4-4957-aec9-d5b370db21e6%2Fvtcypm9_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

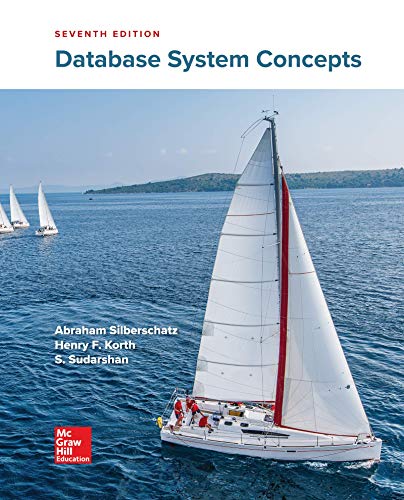
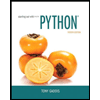
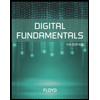
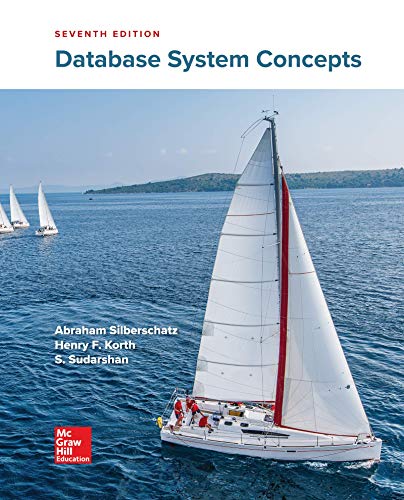
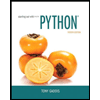
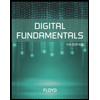
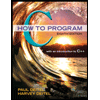
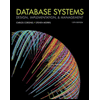
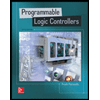