Create a UML class hierarchy (three connected UML diagrams) that has all three classes you created above. Make sure each UML diagram is a one-to-one to the code, it matches the Java code exactly. Make sure that both Child Classes point to their Parent. Hint: your subclasses are siblings. Hint: You need to create three UML diagrams for three created Java classes and connect them using arrows, where each Child Class points to its Parent. You can either draw this by hand and take a picture or use a tool / software of your choice. class Desktop extends Computer { private int width; private int height; public Desktop() { super(); width = height = 0; } public Desktop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, int width, int height) { super(manufacturer, diskSize, manufacturingDate, numberOfCores); this.width = width; this.height = height; } public int getWidth() { return width; } public int getHeight() { return height; } public void setWidth(int width) { this.width = width; } public void setHeight(int height) { this.height = height; } @Override public String toString() { return super.toString() + "\nWidth: " + width + " cm" + "\nHeight: " + height + " cm"; } } class Laptop extends Computer { private double weight; public Laptop() { super(); weight = 0.0; } public Laptop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, double weight) { super(manufacturer, diskSize, manufacturingDate, numberOfCores); this.weight = weight; } public double getWeight() { return weight; } public void setWeight(double weight) { this.weight = weight; } @Override public String toString() { return super.toString() + "\nWeight: " + weight + " kg"; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create a UML class hierarchy (three connected UML diagrams) that has all three classes you
created above. Make sure each UML diagram is a one-to-one to the code, it matches the Java
code exactly. Make sure that both Child Classes point to their Parent.
Hint: your subclasses are siblings.
Hint: You need to create three UML diagrams for three created Java classes and connect them
using arrows, where each Child Class points to its Parent. You can either draw this by hand and
take a picture or use a tool / software of your choice.
class Desktop extends Computer {
private int width;
private int height;
public Desktop() {
super();
width = height = 0;
}
public Desktop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, int width, int height) {
super(manufacturer, diskSize, manufacturingDate, numberOfCores);
this.width = width;
this.height = height;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public void setWidth(int width) {
this.width = width;
}
public void setHeight(int height) {
this.height = height;
}
@Override
public String toString() {
return super.toString() + "\nWidth: " + width + " cm" + "\nHeight: " + height + " cm";
}
}
class Laptop extends Computer {
private double weight;
public Laptop() {
super();
weight = 0.0;
}
public Laptop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, double weight) {
super(manufacturer, diskSize, manufacturingDate, numberOfCores);
this.weight = weight;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
@Override
public String toString() {
return super.toString() + "\nWeight: " + weight + " kg";
}
}

![➡ eclipse-workspace - project2/src/project2/TestComputer.java - Eclipse IDE
File Edit Source Refactor Navigate Search Project Run Window Help
- 2
C
95.
TestPrintPri...
1 package project2;
2 public class TestComputer
3
4
5
6
7
8
9
25
26 }
27
28
project.java
{
public static void main(String[] args) {
29
30 |
10
11
12
13
14
15
16 System.out.println (11);
17 System.out.println();
18 System.out.println (12);
19
20 System.out.println("\nDesktops....");
21 System.out.println(dl);
22 System.out.println();
23
System.out.println (d2);
24
}
#
Difference.java
49°F
Clear
//Two new Desktop
Desktop dl = new Desktop ("Microsoft",
Desktop d2 = new Desktop ("Apple Inc",
//Printing the details
System.out.println("Laptops....");
//Two new laptops
Laptop 11 = new Laptop ("Dell", "1122334455", "April 1, 2020", 4, 5);
Laptop 12 = new Laptop ("Apple Inc", "222222222", "May 15, 2022", 8, 7);
▬▬
▬▬▬
Table.java
MoreArith.java
Q Search
▾▾ ♡
Herjames_Pr...
"1122334455", "August 13, 2019", 4, 20, 30);
"222222222", "December 05, 2022", 8, 25, 35);
Writable
a
- P
Computer.java
Smart Insert
TestCompute... XDesktop.java
30:1:777
Laptop.java
89
a
O
X
* * * + @ 隐旦
8
%
鼎
2
@
@
8:37 PM
4/4/2023](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F467946bb-e41e-4beb-a6a7-a7fc7a246d66%2F0cd36600-9daa-414e-a76d-37b707072b68%2F9jujxpd_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

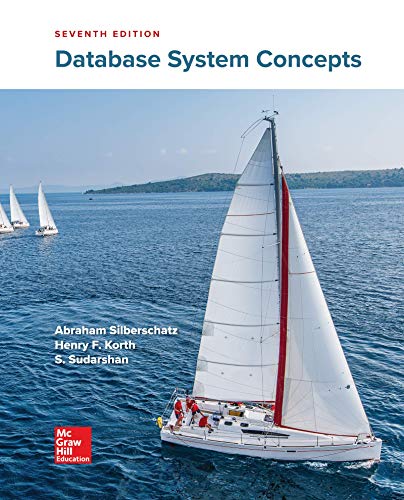
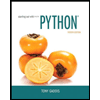
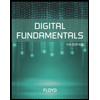
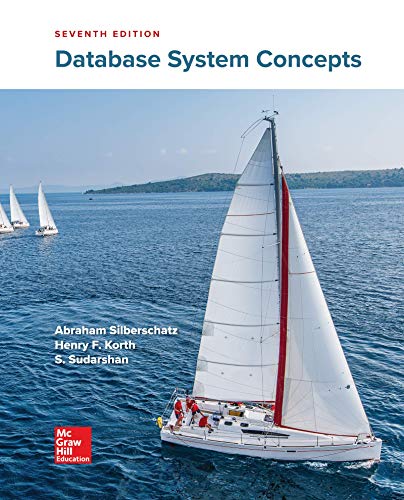
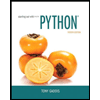
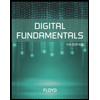
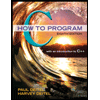
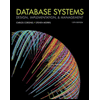
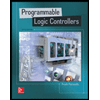