Create a project called Mileage. In it, create a class Car with the following properties. A car has a certain fuelEfficiency (measured in miles/gallon) and a certain amount of fuel (fuelLevel) in the gas tank. Write a constructor for the class that accepts the efficiency value, and sets the initial fuel level to 0. Write the following methods: drive - simulates driving the car for a certain distance, reducing the fuel level in the gas tank. The method should have a parameter for miles - how many miles were driven. It does not return anything. getGasLevel, to return the current fuel level addGas - to add gas to the car. It should have a parameter of how many gallons of gas are being added. Create a car object in main and test your class. Here is a sample run of the program: Car myCar1=newCar(30); // 30 miles per gallon myCar.addGas(20); // Add 20 gallons myCar.drive(100); // Drive 100 milesSystem.out.println(myCar.getGasLevel()); //Print fuel remaining
Create a project called Mileage. In it, create a class Car with the following properties. A car has a certain fuelEfficiency (measured in miles/gallon) and a certain amount of fuel (fuelLevel) in the gas tank. Write a constructor for the class that accepts the efficiency value, and sets the initial fuel level to 0. Write the following methods: drive - simulates driving the car for a certain distance, reducing the fuel level in the gas tank. The method should have a parameter for miles - how many miles were driven. It does not return anything. getGasLevel, to return the current fuel level addGas - to add gas to the car. It should have a parameter of how many gallons of gas are being added. Create a car object in main and test your class. Here is a sample run of the program: Car myCar1=newCar(30); // 30 miles per gallon myCar.addGas(20); // Add 20 gallons myCar.drive(100); // Drive 100 milesSystem.out.println(myCar.getGasLevel()); //Print fuel remaining
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Create a project called Mileage. In it, create a class Car with the following properties. A car has a certain fuelEfficiency (measured in miles/gallon) and a certain amount of fuel (fuelLevel) in the gas tank.
Write a constructor for the class that accepts the efficiency value, and sets the initial fuel level to 0.
Write the following methods:
- drive - simulates driving the car for a certain distance, reducing the fuel level in the gas tank. The method should have a parameter for miles - how many miles were driven. It does not return anything.
- getGasLevel, to return the current fuel level
- addGas - to add gas to the car. It should have a parameter of how many gallons of gas are being added.
Create a car object in main and test your class. Here is a sample run of the program:
Car myCar1=newCar(30); // 30 miles per gallon
myCar.addGas(20); // Add 20 gallons
myCar.drive(100); // Drive 100 miles
System.out.println(myCar.getGasLevel()); //Print fuel remaining
System.out.println(myCar.getGasLevel()); //Print fuel remaining
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
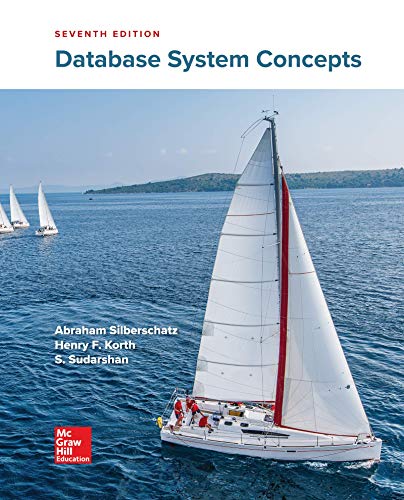
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
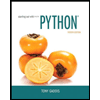
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
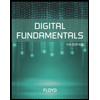
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
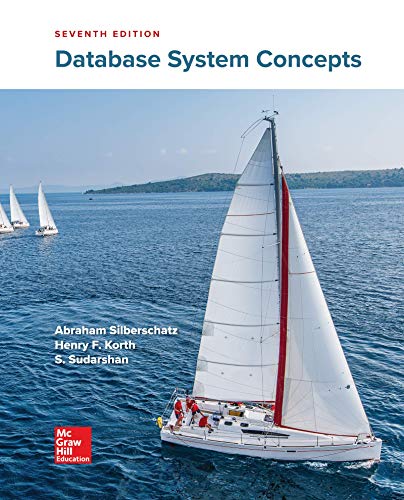
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
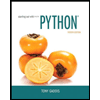
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
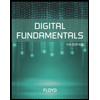
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
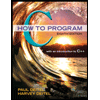
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
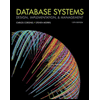
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
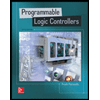
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education