Create a Class for Roman Numerals Create a class called RomanNumeral which has two private instance variables: the String value of the Roman numeral, and an int for the Arabic value. It should have a one-argument constructor that takes a String as a parameter for the Roman Numeral. For this project we will not do error checking. The constructor should set the Roman numeral instance variable, and convert the Roman numeral to Arabic and set the Arabic integer value. Provide get and set methods for the String RomanNumeral and a get method for the Arabic value. Other methods to include are equals, toString, and compare To. Use linked lists to store and sort RomanNumerals Create a class called RomanNumeralList based on the linked list with head node as shown in lecture. The data in the nodes should be a RomanNumeral. Create a class called Unsorted Roman NumeralList that extends RomanNumeralList. It should have an append method that takes a RomanNumeral as a parameter and adds it to the end of the list. Create a class called Sorted Roman NumeralList that extends RomanNumeralList. It should have a method called add which takes a RomanNumeral as a parameter and inserts that word into the list in a position so that the list remains sorted. Read from the file and add to the sorted list For each line in the input file (same file as in project 1), break the line into individual RomanNumerals (Strings) and insert each RomanNumeral into the unsorted list (using append), and into the sorted list (using add). Display the results in a GUI with a Grid Layout of one row and three columns. The first column, as in project 1, should contain the original RomanNumerals, the second column the unsorted Arabic values, and the third column the sorted Arabic values. Submitting the Project. You should now have the following files to submit for this project: Project2.java RomanNumeral.java RomanNumeralGUI.java RomanNumeralList.java UnsortedRoman NumeralList.java SortedRomanNumeralList.java
Create a Class for Roman Numerals Create a class called RomanNumeral which has two private instance variables: the String value of the Roman numeral, and an int for the Arabic value. It should have a one-argument constructor that takes a String as a parameter for the Roman Numeral. For this project we will not do error checking. The constructor should set the Roman numeral instance variable, and convert the Roman numeral to Arabic and set the Arabic integer value. Provide get and set methods for the String RomanNumeral and a get method for the Arabic value. Other methods to include are equals, toString, and compare To. Use linked lists to store and sort RomanNumerals Create a class called RomanNumeralList based on the linked list with head node as shown in lecture. The data in the nodes should be a RomanNumeral. Create a class called Unsorted Roman NumeralList that extends RomanNumeralList. It should have an append method that takes a RomanNumeral as a parameter and adds it to the end of the list. Create a class called Sorted Roman NumeralList that extends RomanNumeralList. It should have a method called add which takes a RomanNumeral as a parameter and inserts that word into the list in a position so that the list remains sorted. Read from the file and add to the sorted list For each line in the input file (same file as in project 1), break the line into individual RomanNumerals (Strings) and insert each RomanNumeral into the unsorted list (using append), and into the sorted list (using add). Display the results in a GUI with a Grid Layout of one row and three columns. The first column, as in project 1, should contain the original RomanNumerals, the second column the unsorted Arabic values, and the third column the sorted Arabic values. Submitting the Project. You should now have the following files to submit for this project: Project2.java RomanNumeral.java RomanNumeralGUI.java RomanNumeralList.java UnsortedRoman NumeralList.java SortedRomanNumeralList.java
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
HELP WITH JAVA
PLEASE PROVIDE INDENTED CODES SO I CAN COPY N PASTE
MAKE SURE UR OUTPUT COMPILES FINE AND IN A GUI PANEL.
TAKE A SCREENSHOT OF OUR OUTPUT AND PASTE HERE AS WELL
ALSO, For project 2 you need to create a class for the list nodes, RomanNumeralListNode
DO THE FOLLOWING:

Transcribed Image Text:Create a Class for Roman Numerals
Create a class called RomanNumeral which has two private instance variables: the String value of the
Roman numeral, and an int for the Arabic value. It should have a one-argument constructor that takes a
String as a parameter for the Roman Numeral. For this project we will not do error checking. The
constructor should set the Roman numeral instance variable, and convert the Roman numeral to Arabic
and set the Arabic integer value. Provide get and set methods for the String RomanNumeral and a get
method for the Arabic value. Other methods to include are equals, toString, and compare To.
Use linked lists to store and sort Roman Numerals
Create a class called Roman NumeralList based on the linked list with head node as shown in lecture.
The data in the nodes should be a RomanNumeral.
Create a class called Unsorted Roman NumeralList that extends Roman NumeralList. It should have an
append method that takes a RomanNumeral as a parameter and adds it to the end of the list.
Create a class called Sorted Roman NumeralList that extends Roman NumeralList. It should have a
method called add which takes a RomanNumeral as a parameter and inserts that word into the list in a
position so that the list remains sorted.
Read from the file and add to the sorted list
For each line in the input file (same file as in project 1), break the line into individual RomanNumerals
(Strings) and insert each RomanNumeral into the unsorted list (using append), and into the sorted list
(using add).
Display the results in a GUI with a GridLayout of one row and three columns. The first column, as in
project 1, should contain the original RomanNumerals, the second column the unsorted Arabic values,
and the third column the sorted Arabic values.
Submitting the Project.
You should now have the following files to submit for this project:
Project2.java
RomanNumeral.java
RomanNumeralGUI.java
RomanNumeralList.java
UnsortedRomanNumeralList.java
SortedRomanNumeralList.java
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
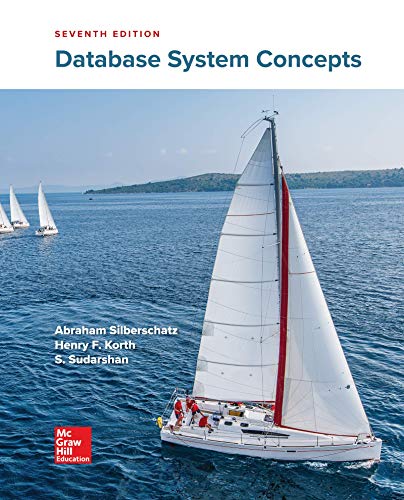
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
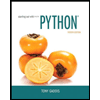
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
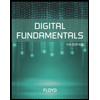
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
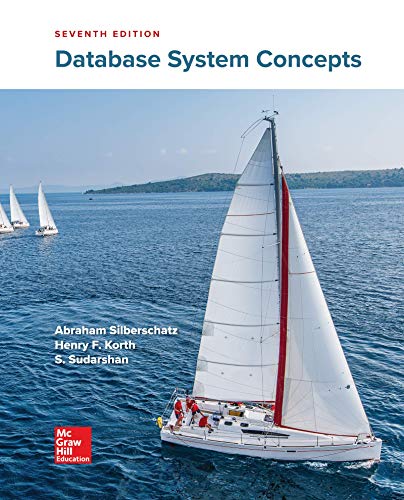
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
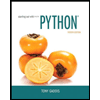
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
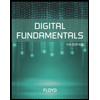
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
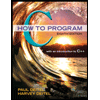
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
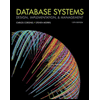
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
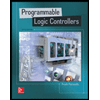
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education