Consider the following skeletal C program: void fun1(void); /* prototype */ void fun2(void); /* prototype */ void fun3(void); /* prototype */ void main() { int a, b, c; . . . } void fun1(void) { int b, c, d; . . . } void fun2(void) { int c, d, e; . . . } void fun3(void) { int d, e, f; . . . } Given the following calling sequences and assuming that dynamic scoping is used, what variables are visible during the execution of the last function? Include with each visible variable the name of the function in which it was defined. a. main calls fun1; fun1 calls fun2; fun2 calls fun3. b. main calls fun1; fun1 calls fun3. c. main calls fun2; fun2 calls fun3; fun3 calls fun1. d. main calls fun3; fun3 calls fun1. e. main calls fun1; fun1 calls fun3; fun3 calls fun2. Consider the following program, written in JavaScript-like syntax: // main program var x, y, z; function sub1() { var a, y, z; . . . } function sub2() { var a, b, z; . . . } function sub3() { var a, x, w; . . . } Given the following calling sequences and assuming that dynamic scoping is used, what variables are visible during the execution of the last subprogram activated? Please include the unit's name where it is declared with each visible variable. a. main calls sub1; sub1 calls sub2; sub2 calls sub3. b. main calls sub1; sub1 calls sub3. c. main calls sub2; sub2 calls sub3; sub3 calls sub1. d. main calls sub3; sub3 calls sub1. e. main calls sub3; sub3 calls sub2; sub2 calls sub1.
Consider the following skeletal C
void fun1(void); /* prototype */
void fun2(void); /* prototype */
void fun3(void); /* prototype */
void main() {
int a, b, c;
. . .
}
void fun1(void) {
int b, c, d;
. . .
}
void fun2(void) {
int c, d, e;
. . .
}
void fun3(void) {
int d, e, f;
. . .
}
Given the following calling sequences and assuming that dynamic scoping is used, what
variables are visible during the execution of the last function? Include with each visible variable
the name of the function in which it was defined.
a. main calls fun1; fun1 calls fun2; fun2 calls fun3.
b. main calls fun1; fun1 calls fun3.
c. main calls fun2; fun2 calls fun3; fun3 calls fun1.
d. main calls fun3; fun3 calls fun1.
e. main calls fun1; fun1 calls fun3; fun3 calls fun2.
Consider the following program, written in JavaScript-like syntax:
// main program
var x, y, z;
function sub1() {
var a, y, z;
. . .
}
function sub2() {
var a, b, z;
. . .
}
function sub3() {
var a, x, w;
. . .
}
Given the following calling sequences and assuming that dynamic scoping is used, what
variables are visible during the execution of the last subprogram activated? Please include the
unit's name where it is declared with each visible variable.
a. main calls sub1; sub1 calls sub2; sub2 calls sub3.
b. main calls sub1; sub1 calls sub3.
c. main calls sub2; sub2 calls sub3; sub3 calls sub1.
d. main calls sub3; sub3 calls sub1.
e. main calls sub3; sub3 calls sub2; sub2 calls sub1.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

For the first problem, in parts b and c, how are the variables being taken from functions that they are not defined in? To clarify, in part c, how is the variable "b" taken from fun2 when it is not defined in that in function. Shouldn't the answer there just be "b(fun1)"
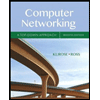
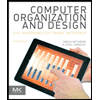
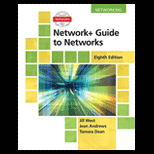
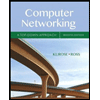
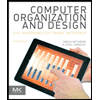
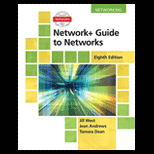
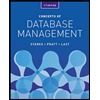
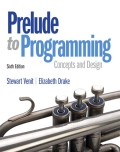
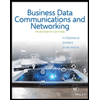