Consider the following method. // precondition: arr contains no duplicates; // the elements in arr are in sorted order; // 0 ≤ low ≤ arr.length; low - 1 ≤ high < arr.length public static int mystery(int[] arr, int low, int high, int num) { int mid = (low + high) / 2; if (low > high) { return low; } else if (arr[mid] < num) { return mystery(arr, mid + 1, high, num); } else if (arr[mid] > num) { return mystery(arr, low, mid - 1, num); } else // arr{mid] == num { return mid; } } How many calls to mystery (including the initial call) are made as a result of the callmystery(arr, 0, arr.length - 1, 14) if arr is the following array? 0 1 2 3 4 5 6 7 arr 11 13 25 26 29 30 31 32 A) 1 B) 2 C) 4 D) 7 E) 8
Consider the following method.
// precondition: arr contains no duplicates;
// the elements in arr are in sorted order;
// 0 ≤ low ≤ arr.length; low - 1 ≤ high < arr.length
public static int mystery(int[] arr, int low, int high, int num)
{
int mid = (low + high) / 2;
if (low > high)
{
return low;
}
else if (arr[mid] < num)
{
return mystery(arr, mid + 1, high, num);
}
else if (arr[mid] > num)
{
return mystery(arr, low, mid - 1, num);
}
else // arr{mid] == num
{
return mid;
}
}
How many calls to mystery (including the initial call) are made as a result of the callmystery(arr, 0, arr.length - 1, 14) if arr is the following array?
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | |
arr | 11 | 13 | 25 | 26 | 29 | 30 | 31 | 32 |
A) 1
B) 2
C) 4
D) 7
E) 8

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

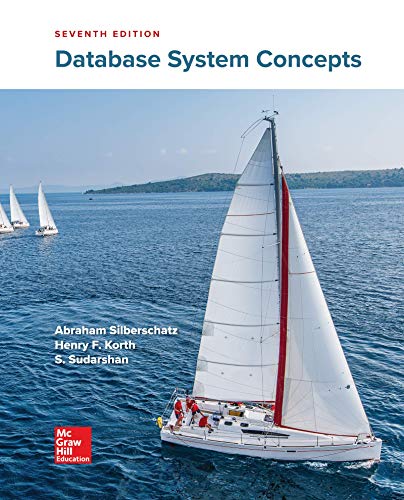
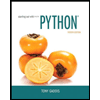
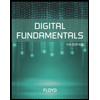
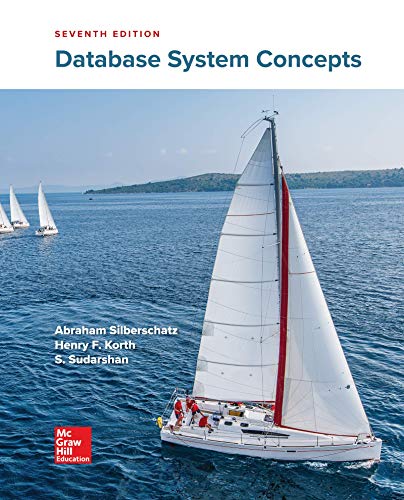
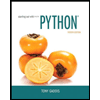
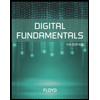
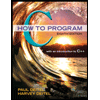
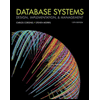
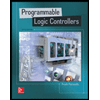