Consider the following code: public class Banker { private BankAccount[] accounts; public Banker(BankAccount[] theAccounts) { accounts = theAccounts; } public BankAccount[] updateOverDueAccounts() { try { for (BankAccount anAccount : accounts) { if (anAccount.getOverdueAmount() <= 250) { anAccount.setOverdueFlag(); } } } catch (Exception ex) { System.out.println ("Exception: " + ex.getMessage()); } return accounts; } } Calculate the following metrics (IMPORTANT: show how each value was calculated): a- The Cyclomatic Complexity of the Banker constructor method b- The Cyclomatic Complexity of the updateOverDueAccounts method c- The WMPC (Weighted-methods-per-class) of the Banker class d- The CBO (Coupling-between-objects) of the Banker class.
Consider the following code:
public class Banker {
private BankAccount[] accounts;
public Banker(BankAccount[] theAccounts) {
accounts = theAccounts;
}
public BankAccount[] updateOverDueAccounts() {
try {
for (BankAccount anAccount : accounts) {
if (anAccount.getOverdueAmount() <= 250) {
anAccount.setOverdueFlag();
}
}
}
catch (Exception ex) {
System.out.println ("Exception: " + ex.getMessage());
}
return accounts;
}
}
Calculate the following metrics (IMPORTANT: show how each value was calculated):
a- The Cyclomatic Complexity of the Banker constructor method
b- The Cyclomatic Complexity of the updateOverDueAccounts method
c- The WMPC (Weighted-methods-per-class) of the Banker class
d- The CBO (Coupling-between-objects) of the Banker class.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

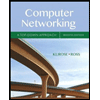
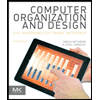
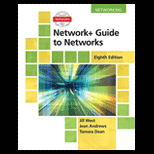
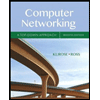
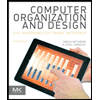
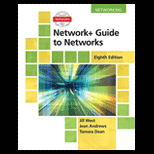
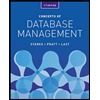
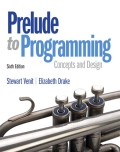
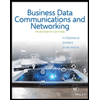