Can you tell me what lines of code in this is an algorithm? // Abstract class for a vehicle abstract class Vehicle { // Common attributes shared by all vehicles protected int year; protected String make; protected String model; protected double price; // Constructor to initialize the common attributes public Vehicle(int year, String make, String model, double price) { this.year = year; this.make = make; this.model = model; this.price = price; } // Common method to print the details of a vehicle public void printDetails() { System.out.println("Year: " + year); System.out.println("Make: " + make); System.out.println("Model: " + model); System.out.println("Price: $" + price); } } // Class for a car class Car extends Vehicle { // Attribute specific to cars private int numDoors; // Constructor to initialize the common and specific attributes public Car(int year, String make, String model, double price, int numDoors) { super(year, make, model, price); this.numDoors = numDoors; } // Method specific to cars public int getNumDoors() { return numDoors; } public void printDetails() { System.out.println("Year: " + year); System.out.println("Make: " + make); System.out.println("Model: " + model); System.out.println("Price: $" + price); System.out.println("Numer of Doors: " + numDoors); } } // Class for a truck class Truck extends Vehicle { // Attribute specific to trucks private int payloadCapacity; // Constructor to initialize the common and specific attributes public Truck(int year, String make, String model, double price, int payloadCapacity) { super(year, make, model, price); this.payloadCapacity = payloadCapacity; } // Method specific to trucks public int getPayloadCapacity() { return payloadCapacity; } public void printDetails() { System.out.println("Year: " + year); System.out.println("Make: " + make); System.out.println("Model: " + model); System.out.println("Price: $" + price); System.out.println("PayloadCapacity " + payloadCapacity); } } // Class for an SUV class SUV extends Vehicle { // Attribute specific to SUVs private int numSeats; // Constructor to initialize the common and specific attributes public SUV(int year, String make, String model, double price, int numSeats) { super(year, make, model, price); this.numSeats = numSeats; } // Method specific to SUVs public int getNumSeats() { return numSeats; } public void printDetails() { System.out.println("Year: " + year); System.out.println("Make: " + make); System.out.println("Model: " + model); System.out.println("Price: $" + price); System.out.println("Number of Seats " + numSeats); } } class Maruti_5 extends Vehicle{ // Attribute specific to Maruti_4 private int numSeats; // Constructor to initialize the common and specific attributes public Maruti_5(int year, String make, String model, double price, int numSeats) { super(year, make, model, price); this.numSeats = numSeats; } public void printDetails() { System.out.println("Year: " + year); System.out.println("Make: " + make); System.out.println("Model: " + model); System.out.println("Price: $" + price); System.out.println("Number of Seats " + numSeats); } } class Maruti_4 extends Vehicle{ // Attribute specific to Maruti_4 private int numSeats; // Constructor to initialize the common and specific attributes public Maruti_4(int year, String make, String model, double price, int numSeats) { super(year, make, model, price); this.numSeats = numSeats; } public void printDetails() { System.out.println("Year: " + year); System.out.println("Make: " + make); System.out.println("Model: " + model); System.out.println("Price: $" + price); System.out.println("Number of Seats " + numSeats); } } class Baleno extends Vehicle{ // Attribute specific to Maruti_4 private int numSeats; // Constructor to initialize the common and specific attributes public Baleno(int year, String make, String model, double price, int numSeats) { super(year, make, model, price); this.numSeats = numSeats; } public void printDetails() { System.out.println("I am using Baleno basic model car"); System.out.println("Number of Seats " + numSeats); } } class Baleno_Alpha extends Vehicle{ // Attribute specific to Maruti_4 private int numSeats; // Constructor to initialize the common and specific attributes public Baleno_Alpha(int year, String make, String model, double price, int numSeats) { super(year, make, model, price); this.numSeats = numSeats;
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Can you tell me what lines of code in this is an
// Abstract class for a vehicle
abstract class Vehicle {
// Common attributes shared by all vehicles
protected int year;
protected String make;
protected String model;
protected double price;
// Constructor to initialize the common attributes
public Vehicle(int year, String make, String model, double price) {
this.year = year;
this.make = make;
this.model = model;
this.price = price;
}
// Common method to print the details of a vehicle
public void printDetails() {
System.out.println("Year: " + year);
System.out.println("Make: " + make);
System.out.println("Model: " + model);
System.out.println("Price: $" + price);
}
}
// Class for a car
class Car extends Vehicle {
// Attribute specific to cars
private int numDoors;
// Constructor to initialize the common and specific attributes
public Car(int year, String make, String model, double price, int numDoors) {
super(year, make, model, price);
this.numDoors = numDoors;
}
// Method specific to cars
public int getNumDoors() {
return numDoors;
}
public void printDetails() {
System.out.println("Year: " + year);
System.out.println("Make: " + make);
System.out.println("Model: " + model);
System.out.println("Price: $" + price);
System.out.println("Numer of Doors: " + numDoors);
}
}
// Class for a truck
class Truck extends Vehicle {
// Attribute specific to trucks
private int payloadCapacity;
// Constructor to initialize the common and specific attributes
public Truck(int year, String make, String model, double price, int payloadCapacity) {
super(year, make, model, price);
this.payloadCapacity = payloadCapacity;
}
// Method specific to trucks
public int getPayloadCapacity() {
return payloadCapacity;
}
public void printDetails() {
System.out.println("Year: " + year);
System.out.println("Make: " + make);
System.out.println("Model: " + model);
System.out.println("Price: $" + price);
System.out.println("PayloadCapacity " + payloadCapacity);
}
}
// Class for an SUV
class SUV extends Vehicle {
// Attribute specific to SUVs
private int numSeats;
// Constructor to initialize the common and specific attributes
public SUV(int year, String make, String model, double price, int numSeats) {
super(year, make, model, price);
this.numSeats = numSeats;
}
// Method specific to SUVs
public int getNumSeats() {
return numSeats;
}
public void printDetails() {
System.out.println("Year: " + year);
System.out.println("Make: " + make);
System.out.println("Model: " + model);
System.out.println("Price: $" + price);
System.out.println("Number of Seats " + numSeats);
}
}
class Maruti_5 extends Vehicle{
// Attribute specific to Maruti_4
private int numSeats;
// Constructor to initialize the common and specific attributes
public Maruti_5(int year, String make, String model, double price, int numSeats) {
super(year, make, model, price);
this.numSeats = numSeats;
}
public void printDetails() {
System.out.println("Year: " + year);
System.out.println("Make: " + make);
System.out.println("Model: " + model);
System.out.println("Price: $" + price);
System.out.println("Number of Seats " + numSeats);
}
}
class Maruti_4 extends Vehicle{
// Attribute specific to Maruti_4
private int numSeats;
// Constructor to initialize the common and specific attributes
public Maruti_4(int year, String make, String model, double price, int numSeats) {
super(year, make, model, price);
this.numSeats = numSeats;
}
public void printDetails() {
System.out.println("Year: " + year);
System.out.println("Make: " + make);
System.out.println("Model: " + model);
System.out.println("Price: $" + price);
System.out.println("Number of Seats " + numSeats);
}
}
class Baleno extends Vehicle{
// Attribute specific to Maruti_4
private int numSeats;
// Constructor to initialize the common and specific attributes
public Baleno(int year, String make, String model, double price, int numSeats) {
super(year, make, model, price);
this.numSeats = numSeats;
}
public void printDetails() {
System.out.println("I am using Baleno basic model car");
System.out.println("Number of Seats " + numSeats);
}
}
class Baleno_Alpha extends Vehicle{
// Attribute specific to Maruti_4
private int numSeats;
// Constructor to initialize the common and specific attributes
public Baleno_Alpha(int year, String make, String model, double price, int numSeats) {
super(year, make, model, price);
this.numSeats = numSeats;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

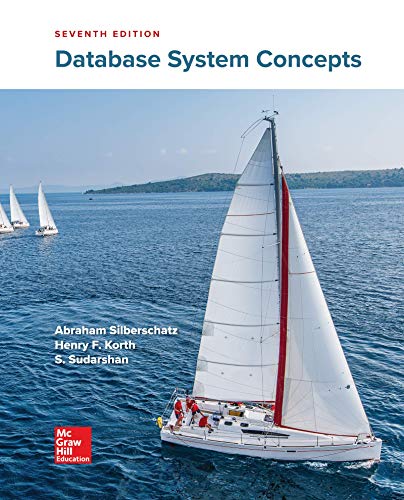
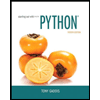
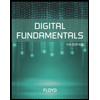
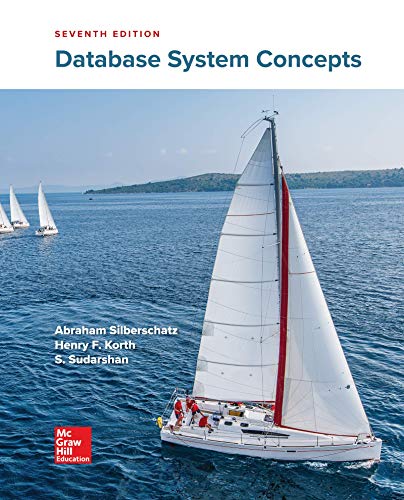
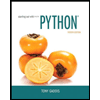
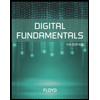
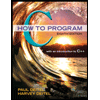
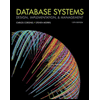
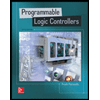