Can you change a bit of the code to out put the monthly rent with two decimals. Thanks! #include #include #include using namespace std; class RealProperty{ private: // streetAddress that hold the street address string streetAddress; // squareFoot that hold the square footage int squareFoot; // taxes that hold the taxes double taxes; public: // default constructor of RealProperty class it's initlizes the fields RealProperty(){ streetAddress = ""; squareFoot = 0; taxes = 0.0; } // parameterized constructor of RealProperty class RealProperty(string sAddress, int sqFootage, double tax){ streetAddress = sAddress; squareFoot = sqFootage; taxes = tax; } // setStreetAddress function for set the street address void setStreetAddress(string sAddress){ streetAddress = sAddress; } //setSquareFootage function for set the square footage void setSquareFootage(int sqFootage){ if(sqFootage > 0){ squareFoot = sqFootage; } else cout << "Square footage must be a positive number." << endl; } // setTaxes function for the set taxes void setTaxes(double tax){ taxes = tax; } string getStreetAddress()const; int getSquareFoot()const; double getTaxes()const; }; // return string RealProperty::getStreetAddress()const{ return streetAddress; } // getSquareFootage function return the square footage int RealProperty::getSquareFoot()const{ return squareFoot; } // getTaxes return the taxes double RealProperty::getTaxes()const{ return taxes; } // Apartment class class Apartment:public RealProperty{ private: double rent; public: // constructor of Apartment Apartment():RealProperty(){rent = 0.0;} // Parameter constructor of Apartment Apartment(string sAddress, int sqFootage, double taxes,double mRent) :RealProperty(sAddress,sqFootage,taxes){rent = mRent;} // setMonthlyRent use for changing the monthly rent. void setMonthlyRent(int mRent){ rent = mRent; } // getMonthlyRent retunr the monthly rent double getMonthlyRent()const{ return rent; } }; //****************************************************************************** //* Print the real property information. //* //* Parameter //* rp - a reference to const referencing to caller's RealProperty //* variable. //* //* Return //* void //****************************************************************************** void displayPropertyInfo(const RealProperty &rp) { // Write your code here . . . cout << "Property is located at: " << rp.getStreetAddress() << endl; cout << "Square footage: " << rp.getSquareFoot() << endl; cout << "Taxes: " << rp.getTaxes() << "\n" << endl; } //****************************************************************************** //* Print the apartment information. //* //* Parameter //* rp - a reference to const referencing to caller's Apartment //* variable. //* //* return //* void //****************************************************************************** void displayApartmentInfo(const Apartment &apt) { cout << "Apartment is located at: " << apt.getStreetAddress() << endl; cout << "Square footage: " << apt.getSquareFoot() << endl; cout << "Taxes: " << apt.getTaxes() <
Can you change a bit of the code to out put the monthly rent with two decimals. Thanks!
#include <iostream>
#include <iomanip>
#include <string>
using namespace std;
class RealProperty{
private:
// streetAddress that hold the street address
string streetAddress;
// squareFoot that hold the square footage
int squareFoot;
// taxes that hold the taxes
double taxes;
public:
// default constructor of RealProperty class it's initlizes the fields
RealProperty(){
streetAddress = "";
squareFoot = 0;
taxes = 0.0;
}
// parameterized constructor of RealProperty class
RealProperty(string sAddress, int sqFootage, double tax){
streetAddress = sAddress;
squareFoot = sqFootage;
taxes = tax;
}
// setStreetAddress function for set the street address
void setStreetAddress(string sAddress){
streetAddress = sAddress;
}
//setSquareFootage function for set the square footage
void setSquareFootage(int sqFootage){
if(sqFootage > 0){
squareFoot = sqFootage;
}
else
cout << "Square footage must be a positive number." << endl;
}
// setTaxes function for the set taxes
void setTaxes(double tax){
taxes = tax;
}
string getStreetAddress()const;
int getSquareFoot()const;
double getTaxes()const;
};
// return
string RealProperty::getStreetAddress()const{
return streetAddress;
}
// getSquareFootage function return the square footage
int RealProperty::getSquareFoot()const{
return squareFoot;
}
// getTaxes return the taxes
double RealProperty::getTaxes()const{
return taxes;
}
// Apartment class
class Apartment:public RealProperty{
private:
double rent;
public:
// constructor of Apartment
Apartment():RealProperty(){rent = 0.0;}
// Parameter constructor of Apartment
Apartment(string sAddress, int sqFootage, double taxes,double mRent)
:RealProperty(sAddress,sqFootage,taxes){rent = mRent;}
// setMonthlyRent use for changing the monthly rent.
void setMonthlyRent(int mRent){
rent = mRent;
}
// getMonthlyRent retunr the monthly rent
double getMonthlyRent()const{
return rent;
}
};
//******************************************************************************
//* Print the real property information.
//*
//* Parameter
//* rp - a reference to const referencing to caller's RealProperty
//* variable.
//*
//* Return
//* void
//******************************************************************************
void displayPropertyInfo(const RealProperty &rp) {
// Write your code here . . .
cout << "Property is located at: " << rp.getStreetAddress() << endl;
cout << "Square footage: " << rp.getSquareFoot() << endl;
cout << "Taxes: " << rp.getTaxes() << "\n" << endl;
}
//******************************************************************************
//* Print the apartment information.
//*
//* Parameter
//* rp - a reference to const referencing to caller's Apartment
//* variable.
//*
//* return
//* void
//******************************************************************************
void displayApartmentInfo(const Apartment &apt) {
cout << "Apartment is located at: " << apt.getStreetAddress() << endl;
cout << "Square footage: " << apt.getSquareFoot() << endl;
cout << "Taxes: " << apt.getTaxes() <<endl;
cout << "Monthly rent: " << apt.getMonthlyRent() << endl;
}
int main() {
Apartment lakeside("Cupertino",1200,200,2550);
// call displayPropertyInfo function
displayPropertyInfo(lakeside);
// call the displayApartmentInfo function
displayApartmentInfo(lakeside);
return 0;
}


Step by step
Solved in 3 steps with 1 images

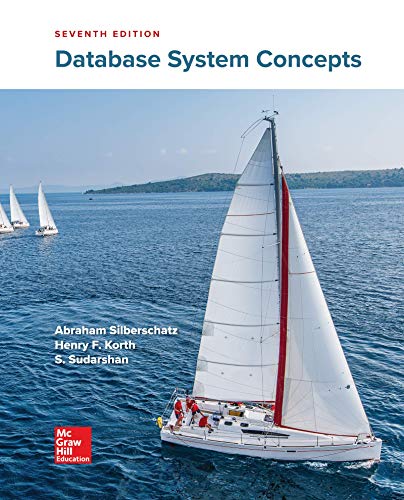
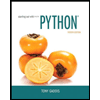
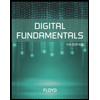
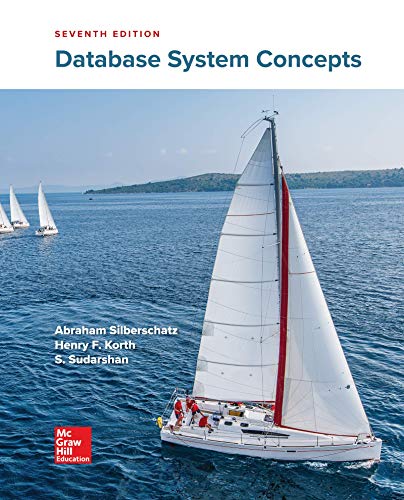
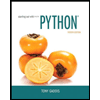
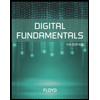
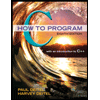
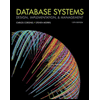
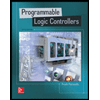