Can someone help me with the following program? I need to add the following changes to my code A “Reset” button should clear the input and result values shown. A “reverse conversion” checkbox to make the conversion to work in reverse Here's my code PROGRAM Converter.java import javax.swing.JFrame; /** * The main driver program for the GUI based conversion program. * * @author mdixon */ public class Converter { public static void main(String[] args) { JFrame frame = new JFrame("Converter"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); MainPanel panel = new MainPanel(); // frame.setJMenuBar(panel.setupMenu()); frame.getContentPane().add(panel); frame.pack(); frame.setVisible(true); } } MainPanel.java import java.awt.Color; import java.awt.Dimension; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.BufferedReader; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStreamReader; import java.util.Vector; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JTextField; @SuppressWarnings("serial") public class MainPanel extends JPanel { // private final static String[] list = { "inches/cm" }; private JTextField textField; private JLabel label; // Declaring combo of type currency private JComboBox combo; /* * Method to load currency data from file */ private void initCombo(String file) { // creating vector to provide as data of combobox Vector model = new Vector<>(); try { // Reading data from file BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream(file), "UTF8")); String line; // iterating through the file line by line while ((line = br.readLine()) != null) { // splitting line using comma String arr[] = line.split(","); // creating instance of currency object Currency currency = new Currency(); // setting values to currency object currency.setName(arr[0].trim()); currency.setValue(Double.parseDouble(arr[1].trim())); currency.setSymbol(arr[2].trim()); // adding currency object to model model.add(currency); } // closing reader br.close(); } catch (IOException e) { e.printStackTrace(); } // initializing combo box with elements in the Vector. combo = new JComboBox(model); } MainPanel() { ActionListener listener = new ConvertListener(); initCombo("currency.txt"); // calling method to create combo box combo.addActionListener(listener); // convert values when option changed JLabel inputLabel = new JLabel("Enter value:"); JButton convertButton = new JButton("Convert"); convertButton.addActionListener(listener); // convert values when pressed label = new JLabel("---"); textField = new JTextField(5); add(combo); add(inputLabel); add(textField); add(convertButton); add(label); setPreferredSize(new Dimension(800, 80)); setBackground(Color.LIGHT_GRAY); } private class ConvertListener implements ActionListener { @Override public void actionPerformed(ActionEvent event) { String text = textField.getText().trim(); if (text.isEmpty() == false) { double value = Double.parseDouble(text); // the factor applied during the conversion double factor = 0; // the offset applied during the conversion. double offset = 0; // Setup the correct factor/offset values depending on required conversion // switch (combo.getSelectedIndex()) { // // case 0: // inches/cm // factor = 2.54; // break; // } // // Setting factor using the value of selected item factor = ((Currency) combo.getSelectedItem()).getValue(); double result = factor * value + offset; // Setting symbol using the symbol of selected item label.setText(((Currency) combo.getSelectedItem()).getSymbol() + " " + Double.toString(result)); } } } /** * Class to keep currency data read from the file * */ private class Currency { private String name; // name of currency private Double value; // value of currency private String symbol; // symbol of currency // Getters and Setters of the class public String getName() { return name; } public void setName(String name) { this.name = name; } public Double getValue() { return value; } public void setValue(Double value) { this.value = value; } public String getSymbol() { return symbol; } public void setSymbol(String symbol) { this.symbol = symbol; } /* * Overriding toString, the value returned from this method will be shown inside * combobox */ @Override public String toString() { return name; } } } Currency file currency.txt Euro (EUR), 1.06, € US Dollars (USD), 1.14, $ Australian Dollars (AUD), 1.52, $ Canadian Dollars (CAD), 1.77, $ Icelandic króna (ISK), 130.62, kr United Arab Emirates Dirham (AED), 4.60, د.إ South African Rand (ZAR), 17.96, R Thai Baht (THB), 44.28, ฿
Can someone help me with the following program? I need to add the following changes to my code A “Reset” button should clear the input and result values shown. A “reverse conversion” checkbox to make the conversion to work in reverse Here's my code PROGRAM Converter.java import javax.swing.JFrame; /** * The main driver program for the GUI based conversion program. * * @author mdixon */ public class Converter { public static void main(String[] args) { JFrame frame = new JFrame("Converter"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); MainPanel panel = new MainPanel(); // frame.setJMenuBar(panel.setupMenu()); frame.getContentPane().add(panel); frame.pack(); frame.setVisible(true); } } MainPanel.java import java.awt.Color; import java.awt.Dimension; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.BufferedReader; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStreamReader; import java.util.Vector; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JTextField; @SuppressWarnings("serial") public class MainPanel extends JPanel { // private final static String[] list = { "inches/cm" }; private JTextField textField; private JLabel label; // Declaring combo of type currency private JComboBox combo; /* * Method to load currency data from file */ private void initCombo(String file) { // creating vector to provide as data of combobox Vector model = new Vector<>(); try { // Reading data from file BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream(file), "UTF8")); String line; // iterating through the file line by line while ((line = br.readLine()) != null) { // splitting line using comma String arr[] = line.split(","); // creating instance of currency object Currency currency = new Currency(); // setting values to currency object currency.setName(arr[0].trim()); currency.setValue(Double.parseDouble(arr[1].trim())); currency.setSymbol(arr[2].trim()); // adding currency object to model model.add(currency); } // closing reader br.close(); } catch (IOException e) { e.printStackTrace(); } // initializing combo box with elements in the Vector. combo = new JComboBox(model); } MainPanel() { ActionListener listener = new ConvertListener(); initCombo("currency.txt"); // calling method to create combo box combo.addActionListener(listener); // convert values when option changed JLabel inputLabel = new JLabel("Enter value:"); JButton convertButton = new JButton("Convert"); convertButton.addActionListener(listener); // convert values when pressed label = new JLabel("---"); textField = new JTextField(5); add(combo); add(inputLabel); add(textField); add(convertButton); add(label); setPreferredSize(new Dimension(800, 80)); setBackground(Color.LIGHT_GRAY); } private class ConvertListener implements ActionListener { @Override public void actionPerformed(ActionEvent event) { String text = textField.getText().trim(); if (text.isEmpty() == false) { double value = Double.parseDouble(text); // the factor applied during the conversion double factor = 0; // the offset applied during the conversion. double offset = 0; // Setup the correct factor/offset values depending on required conversion // switch (combo.getSelectedIndex()) { // // case 0: // inches/cm // factor = 2.54; // break; // } // // Setting factor using the value of selected item factor = ((Currency) combo.getSelectedItem()).getValue(); double result = factor * value + offset; // Setting symbol using the symbol of selected item label.setText(((Currency) combo.getSelectedItem()).getSymbol() + " " + Double.toString(result)); } } } /** * Class to keep currency data read from the file * */ private class Currency { private String name; // name of currency private Double value; // value of currency private String symbol; // symbol of currency // Getters and Setters of the class public String getName() { return name; } public void setName(String name) { this.name = name; } public Double getValue() { return value; } public void setValue(Double value) { this.value = value; } public String getSymbol() { return symbol; } public void setSymbol(String symbol) { this.symbol = symbol; } /* * Overriding toString, the value returned from this method will be shown inside * combobox */ @Override public String toString() { return name; } } } Currency file currency.txt Euro (EUR), 1.06, € US Dollars (USD), 1.14, $ Australian Dollars (AUD), 1.52, $ Canadian Dollars (CAD), 1.77, $ Icelandic króna (ISK), 130.62, kr United Arab Emirates Dirham (AED), 4.60, د.إ South African Rand (ZAR), 17.96, R Thai Baht (THB), 44.28, ฿
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
JAVA HELP NEEDED!
Can someone help me with the following program? I need to add the following changes to my code
-
A “Reset” button should clear the input and result values shown.
-
A “reverse conversion” checkbox to make the conversion to work in reverse
Here's my code
PROGRAM
Converter.java
import javax.swing.JFrame; /** * The main driver program for the GUI based conversion program. * * @author mdixon */ public class Converter { public static void main(String[] args) { JFrame frame = new JFrame("Converter"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); MainPanel panel = new MainPanel(); // frame.setJMenuBar(panel.setupMenu()); frame.getContentPane().add(panel); frame.pack(); frame.setVisible(true); } }MainPanel.java
import java.awt.Color; import java.awt.Dimension; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.BufferedReader; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStreamReader; import java.util.
Currency file currency.txt
Euro (EUR), 1.06, €
US Dollars (USD), 1.14, $
Australian Dollars (AUD), 1.52, $
Canadian Dollars (CAD), 1.77, $
Icelandic króna (ISK), 130.62, kr
United Arab Emirates Dirham (AED), 4.60, د.إ
South African Rand (ZAR), 17.96, R
Thai Baht (THB), 44.28, ฿
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
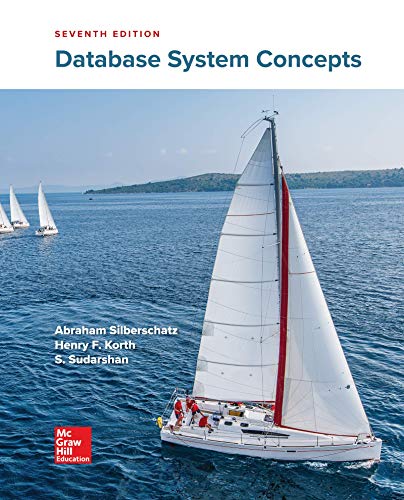
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
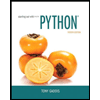
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
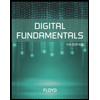
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
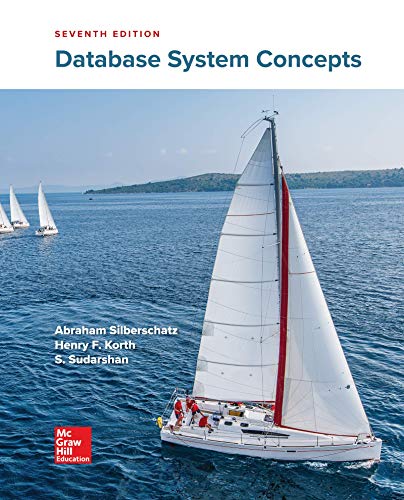
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
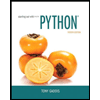
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
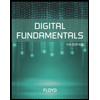
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
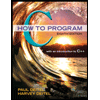
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
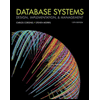
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
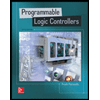
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education