C++ Write a function that uses a stack to test whether a given string (as a character array) is a palindrome. Exercise 14 asked you to write an algorithm for such a function Use the STL library stack. Although this solution can be solved with simple looping (and it is with the isPlaindromIterative function) - we want to demonstrate an understanding of stack structures to check for a palindrome. Key Topics Input accept a string (as a character array) add characters to the stack Processing push pop peek (top) Output use stack methods to check for palindromes Efficient Correct bool isPalindromeStack(std::string word) { bool returnValue = true; /* Your Code Here*/ return returnValue; } #endif /* STUDENT_SOLUTION_CPP */ Main.cpp #include #include #include #include #include #include "studentSolution.hpp" using namespace std; bool isPlaindromIterative(string word) { int rightSearchIndex = word.length()-1; for(int leftSearchIndex=0; leftSearchIndex
C++
Write a function that uses a stack to test whether a given string (as a character array) is a palindrome. Exercise 14 asked you to write an
Use the STL library stack.
Although this solution can be solved with simple looping (and it is with the isPlaindromIterative function) - we want to demonstrate an understanding of stack structures to check for a palindrome.
Key Topics
Input
- accept a string (as a character array)
- add characters to the stack
Processing
- push
- pop
- peek (top)
Output
- use stack methods to check for palindromes
- Efficient
- Correct
bool isPalindromeStack(std::string word) {
bool returnValue = true;
/* Your Code Here*/
return returnValue;
}
#endif /* STUDENT_SOLUTION_CPP */
Main.cpp
#include <iostream>
#include <string>
#include <stdlib.h>
#include <stdio.h>
#include <time.h>
#include "studentSolution.hpp"
using namespace std;
bool isPlaindromIterative(string word) {
int rightSearchIndex = word.length()-1;
for(int leftSearchIndex=0; leftSearchIndex<rightSearchIndex; leftSearchIndex++, rightSearchIndex--)
if(word[leftSearchIndex] != word[rightSearchIndex])
return false;
return true;
}
int main() {
string testString;
cout << "Test01: Empty word" << endl;
testString = "";
cout << '\t' << testString << " correct answer: " << isPlaindromIterative(testString) << endl;
cout << '\t' << testString << " student answer: " << isPalindromeStack(testString) << endl;
cout << "Test02: Odd number palindrome" << endl;
testString = "racecar";
cout << '\t' << testString << " correct answer: " << isPlaindromIterative(testString) << endl;
cout << '\t' << testString << " student answer: " << isPalindromeStack(testString) << endl;
cout << "Test03: Even number palindrome" << endl;
testString = "tacoocat";
cout << '\t' << testString << " correct answer: " << isPlaindromIterative(testString) << endl;
cout << '\t' << testString << " student answer: " << isPalindromeStack(testString) << endl;
cout << "Test04: Non Palindrome" << endl;
testString = "bananaSlug";
cout << '\t' << testString << " correct answer: " << isPlaindromIterative(testString) << endl;
cout << '\t' << testString << " student answer: " << isPalindromeStack(testString) << endl;

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

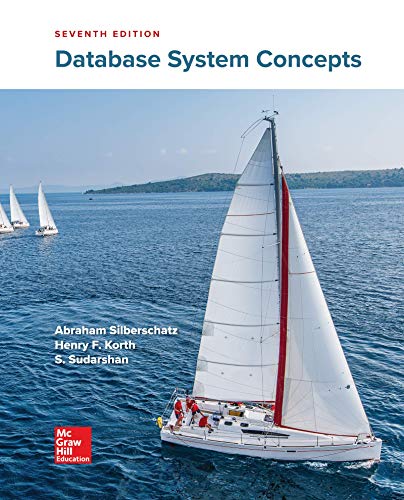
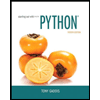
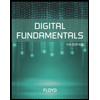
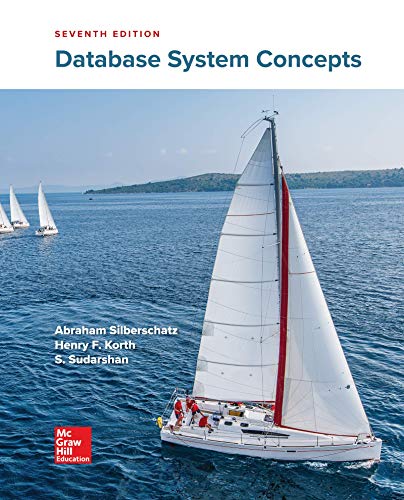
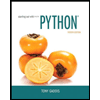
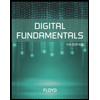
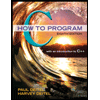
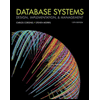
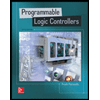