io.h> #include
Please fill in the blanks for C.
/* This program will create a doubly linked list of full names. */
#include<stdio.h>
#include<stdlib.h>
#define LEN 100 //max length
//Declare a node of string that contain a person's full name
// each node has a value and 2 pointers that points to the next node and previous node
struct node
{
__1__ value[__2__]; //to save the string (full name)
__3__ __4__ next; //pointer points to next node
__5__ __6__ prev; //pointer points to previous node
};
/* - This function takes the head pointer and iterates through the list
to get one full name at a time from the user, and save it to our list
- The function uses getchar() to get 1 character at a time, and only stops when user presses Enter.
- During each iteration, check if user inputs a name that is longer than max size.
+ Remember, if maxLen is 100, then the longest valid inputted string len is 98, because 99th index is for null character.
+ So we should stop (invalid) if at any point, the current index reaches index 99, and it's still not the enter key.
- When done scanning 1 name, make sure to end the string using the pointer.
- Update the pointer to point to the next node.*/
__7__ scanLL(__8__ __9__ head)
{
int i;
while(__10__ __11__ __12__) //this condition makes sure the list is not at the end
{
printf("Please put in the name: ");
char curC = __13__(); //call a function to get a character
for(i = 0; curC __14__ __15__; i++) //loop runs as long as user hasnt' clicked Enter key
{
//Check if current index is valid, if not, print a message and exit the program
if(__16__ == __17__ - __18__)
{
printf("Your name is too long. Bye\n");
__19__; //terminate the program, no space
}
__20__ -> __21__ = __22__;// save the character as part of the string, no space
curC = __23__(); //call a function to get another character
}
__24__ -> __25__ = __26__; //end the current string, no space
head = __27__; //move to next node, no space
}
printf("\n");
}
/*This function prints the list forwards.*/
__28__ printForwards(__29__ __30__ head)
{
while(__31__ __32__ __33__)//this condition makes sure the list is not at the end
{
printf("%s\n", __34__); //print current node's string value, no space
head = __35__; //move to next node
}
printf("\n");
}
//PART 2
/*This function prints the list backwards.*/
__36__ printBackwards(__37__ __38__ head)
{
while(__39__ __40__ __41__)
{
printf("__42__\n", __43__);//print current node's string value
head = __44__; //move to previous node
}
printf("\n");
}
int main()
{
//PART 1
//Create 5 character nodes, and link a head pointer to first node.
__45__ __46__ n1,n2,n3,n4; //nodes
__47__ __48__ head = __49__; //head pointer points to first node n1, no spaces
//Link all nodes to its next, no spaces
// we'll do in this order n1 -> n2 -> n3 -> n4
__50__ = __51__; //make a link from n1 to n2
__52__ = __53__; //make a link from n2 to n3
__54__ = __55__; //make a link from n3 to n4
__56__ = __57__; //make a link to show n4 is the end
scanLL(head); //call the function to scan the strings for our linked list
printf("\nPrinting back the inputted linked ost:\n");
printForwards(head); //call the function to print back our list
//PART 2
char userIn;
//Link all nodes to its previous, no spaces
// n4 -> n3 -> n2 -> n1
__58__ = __59__; //make a link from n4 to n3
__60__ = __61__; //make a link from n3 to n2
__62__ = __63__; //make a link from n2 to n1
__64__ = __65__; //make a link to show n1 is the end
//Get a character from user to print (B)ackwards or (F)orward
printf("Enter F/f to print forward and B/b to print backward: ");
scanf("%c", &userIn);
//use switch case to execute accordingly based on user's choice
switch(__66__)
{
case 'F':
case 'f':
head = __67__; //set the head to correct node so it'll print forwards
printf("\nPrinting Forwards:\n");
printForwards(__68__);
break;
case 'B':
case 'b':
head = __69__;//set the head to correct node so it'll print backwards
printf("\nPrinting Backwards:\n");
printBackwards(__70__);
break;
default:
printf("Invalid input. Bye\n");
break;
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

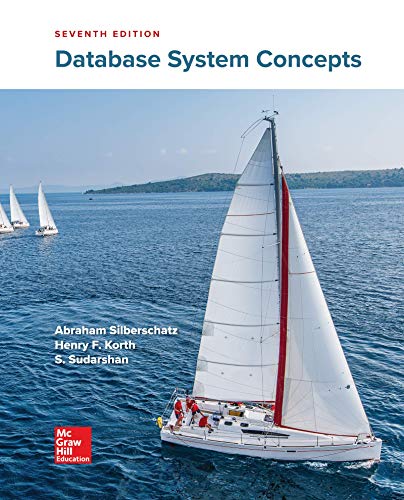
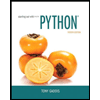
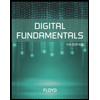
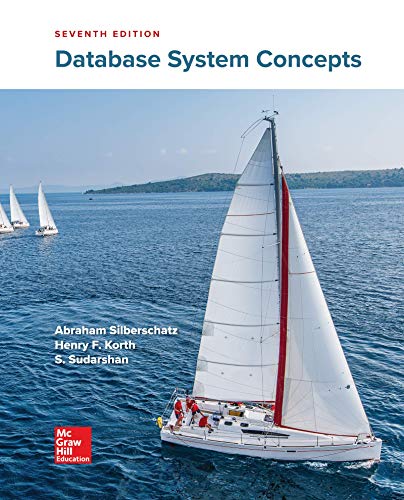
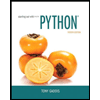
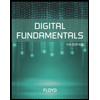
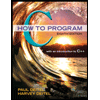
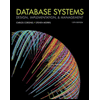
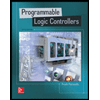