#include #include #include "llcpInt.h" using namespace std; // definition of DelOddCopEven of Assignment 5 Part 1 // (put at near top to facilitate printing and grading) //Algorithm should: /*NOT destroy any of the originally even-valued node. This means that the originally even-valued nodes should be retained as part of the resulting list. Destroy ONLY nodes that are originally odd-valued. Create new nodes and copy data between nodes ONLY when duplicating originally even-valued nodes. Only ONE new node should be created for each originally even-valued node. Creating pointer-to-node's (to provide temporary storage for node addresses) does not constitute creating new nodes or copying of items. NOT make any temporary copies of any of the data items (using any kind of data structures including but not limited to arrays, linked lists and trees). This means that the algorithm should largely involve the manipulation of pointers. Function must be iterative (NOT recursive) - use only looping construct(s), without the function calling itself (directly or indirectly). Function should not call any other functions to help in performing the task. */
#include <iostream>
#include <cstdlib>
#include "llcpInt.h"
using namespace std;
// definition of DelOddCopEven of Assignment 5 Part 1
// (put at near top to facilitate printing and grading)
//
/*NOT destroy any of the originally even-valued node.
This means that the originally even-valued nodes should be retained as part of the resulting list.
Destroy ONLY nodes that are originally odd-valued.
Create new nodes and copy data between nodes ONLY when duplicating originally even-valued nodes.
Only ONE new node should be created for each originally even-valued node.
Creating pointer-to-node's (to provide temporary storage for node addresses) does not constitute creating new nodes or copying of items.
NOT make any temporary copies of any of the data items (using any kind of data structures including but not limited to arrays, linked lists and trees).
This means that the algorithm should largely involve the manipulation of pointers.
Function must be iterative (NOT recursive) - use only looping construct(s), without the function calling itself (directly or indirectly).
Function should not call any other functions to help in performing the task.
*/
#ifndef LLCP_INT_H
#define LLCP_INT_H
#include <iostream>
struct Node
{
int data;
Node *link;
};
bool DelOddCopEven(Node* headPtr);
int FindListLength(Node* headPtr);
bool IsSortedUp(Node* headPtr);
void InsertAsHead(Node*& headPtr, int value);
void InsertAsTail(Node*& headPtr, int value);
void InsertSortedUp(Node*& headPtr, int value);
bool DelFirstTargetNode(Node*& headPtr, int target);
bool DelNodeBefore1stMatch(Node*& headPtr, int target);
void ShowAll(std::ostream& outs, Node* headPtr);
void FindMinMax(Node* headPtr, int& minValue, int& maxValue);
double FindAverage(Node* headPtr);
void ListClear(Node*& headPtr, int noMsg = 0);
// prototype of DelOddCopEven of Assignment 5 Part 1 #endif
================================
passed test on empty list
================================
test case 1 of 1000000
initial: 6 7 5 3
ought2b: 6 6
outcome: 6 6
================================
test case 2 of 1000000
initial: 6 2 9 1 2 7
ought2b: 6 6 2 2 2 2
outcome: 6 6 2 2 2 2
================================
test case 3 of 1000000
initial: 9
ought2b:
outcome:
================================
test case 4 of 1000000
initial: 6 0 6 2
ought2b: 6 6 0 0 6 6 2 2
outcome: 6 6 0 0 6 6 2 2
================================
test case 5 of 1000000
initial: 1 8 7 9 2 0 2
ought2b: 8 8 2 2 0 0 2 2
outcome: 8 8 2 2 0 0 2 2
================================
test case 40000 of 1000000
initial: 5 5 1
ought2b:
outcome:
================================
test case 80000 of 1000000
initial: 2
ought2b: 2 2
outcome: 2 2
================================
test case 120000 of 1000000
initial: 4 4 1 8
ought2b: 4 4 4 4 8 8
outcome: 4 4 4 4 8 8
================================
test case 160000 of 1000000
initial: 0 4 6
ought2b: 0 0 4 4 6 6
outcome: 0 0 4 4 6 6
================================
test case 200000 of 1000000
initial: 1 7 0 3 7 7 3
ought2b: 0 0
outcome: 0 0
================================
test case 240000 of 1000000
initial: 6 2 3 8
ought2b: 6 6 2 2 8 8
outcome: 6 6 2 2 8 8
================================
test case 280000 of 1000000
initial: 8 6 7 9 7 1 1 9 9
ought2b: 8 8 6 6
outcome: 8 8 6 6
================================
test case 320000 of 1000000
initial: 7 5
ought2b:
outcome:
================================
test case 360000 of 1000000
initial: 2 9 6 9 3 7 7
ought2b: 2 2 6 6
outcome: 2 2 6 6
================================
test case 400000 of 1000000
initial: 8
ought2b: 8 8
outcome: 8 8
================================
test case 440000 of 1000000
initial: 6 1 1 1 9 9 7 6 8
ought2b: 6 6 6 6 8 8
outcome: 6 6 6 6 8 8
================================
test case 480000 of 1000000
initial: 8 3 4
ought2b: 8 8 4 4
outcome: 8 8 4 4
================================
test case 520000 of 1000000
initial: 3 6 2 1 6 4 2
ought2b: 6 6 2 2 6 6 4 4 2 2
outcome: 6 6 2 2 6 6 4 4 2 2
================================
test case 560000 of 1000000
initial: 3 3 8 8 4
ought2b: 8 8 8 8 4 4
outcome: 8 8 8 8 4 4
================================
test case 600000 of 1000000
initial: 7 8 6 6 6 0 7 8 5
ought2b: 8 8 6 6 6 6 6 6 0 0 8 8
outcome: 8 8 6 6 6 6 6 6 0 0 8 8
================================
test case 640000 of 1000000
initial: 6 7 0
ought2b: 6 6 0 0
outcome: 6 6 0 0
================================
test case 680000 of 1000000
initial: 5 8 0 3 7
ought2b: 8 8 0 0
outcome: 8 8 0 0
================================
test case 720000 of 1000000
initial: 4 7 9 5 9 3 4 1 4 4
ought2b: 4 4 4 4 4 4 4 4
outcome: 4 4 4 4 4 4 4 4



Step by step
Solved in 3 steps

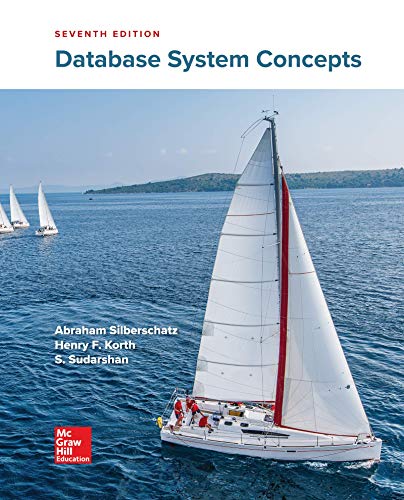
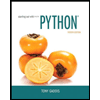
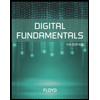
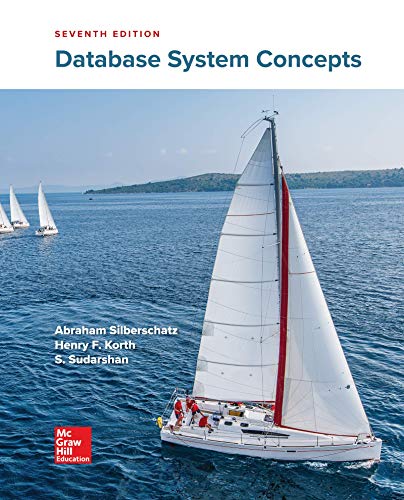
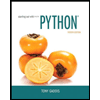
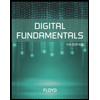
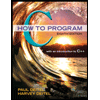
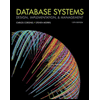
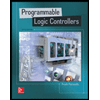