C++ Program Use the FlightInfo class in the attached FlightInfo.h file for this assignment. Task 1: Modify the FlightInfo.h file to include the following (empty) Exception Classes. Invalid Direction Invalid Fuel Level Invalid Altitude Invalid Speed Modify the setter methods to throw an exception for invalid data. Note that these setters are void. They create exceptions instead of returning a value for invalid data. Task 2: Create a driver program that will instantiate a FlightInfo object. Driver Program steps. Prompt the user for the following inputs values Speed Direction Altitude Fuel Level Call the flightInfo's showAll() method. Catch these exceptions in the driver program and display a message anytime invalid data is given indicating the issue. The valid ranges are commented in the FlightInfo.h file. As usual, work on a small section like Invalid Direction and confirm that works as expected. Once that is good, the rest are very similar. 6 point Bonus. Create an additional Exception Class named FuelWarning if the Fuel < 10 and Altitude > 1000. Throw this exception where appropriate and catch in the driver program. FlightInfo.h: #ifndef FlightInfo_H #define FlightInfo_H #include using namespace std; class FlightInfo { private: int absoluteAltitude = 0; int speed = 0; int fuelLevel = 0; int direction = 0; public: // setters //valid 0-10000 void setAbsoluteAltitude(int alt) { absoluteAltitude = alt; } //valid 0 - 650 void setSpeed(int currentSpeed) { speed = currentSpeed; } // Valid 0 - 100 void setFuelLevel(int level) { fuelLevel = level; } // valid 0-360 void setDirection(int heading) { direction = heading; } // getters int getAbsoluteAltitude() { return absoluteAltitude; } int getSpeed() { return speed; } int getFuelLevel() { return fuelLevel; } int getDirection() { return direction; } void showAll() { cout <<"Altitude = " << getAbsoluteAltitude() << endl; cout <<"Speed = " << getSpeed() << endl; cout <<"FuelLevel = " << getFuelLevel() << endl; cout <<"Direction = " << getDirection() << endl; } }; #endif
C++
Use the FlightInfo class in the attached FlightInfo.h file for this assignment.
Task 1: Modify the FlightInfo.h file to include the following (empty) Exception Classes.
- Invalid Direction
- Invalid Fuel Level
- Invalid Altitude
- Invalid Speed
- Modify the setter methods to throw an exception for invalid data. Note that these setters are void. They create exceptions instead of returning a value for invalid data.
Task 2: Create a driver program that will instantiate a FlightInfo object.
Driver Program steps.
- Prompt the user for the following inputs values
- Speed
- Direction
- Altitude
- Fuel Level
- Call the flightInfo's showAll() method.
- Catch these exceptions in the driver program and display a message anytime invalid data is given indicating the issue. The valid ranges are commented in the FlightInfo.h file.
As usual, work on a small section like Invalid Direction and confirm that works as expected. Once that is good, the rest are very similar.
6 point Bonus. Create an additional Exception Class named FuelWarning if the Fuel < 10 and Altitude > 1000. Throw this exception where appropriate and catch in the driver program.
FlightInfo.h:
#ifndef FlightInfo_H
#define FlightInfo_H
#include <iostream>
using namespace std;
class FlightInfo
{
private:
int absoluteAltitude = 0;
int speed = 0;
int fuelLevel = 0;
int direction = 0;
public:
// setters
//valid 0-10000
void setAbsoluteAltitude(int alt) {
absoluteAltitude = alt;
}
//valid 0 - 650
void setSpeed(int currentSpeed) {
speed = currentSpeed;
}
// Valid 0 - 100
void setFuelLevel(int level) {
fuelLevel = level;
}
// valid 0-360
void setDirection(int heading) {
direction = heading;
}
// getters
int getAbsoluteAltitude() {
return absoluteAltitude;
}
int getSpeed() {
return speed;
}
int getFuelLevel() {
return fuelLevel;
}
int getDirection() {
return direction;
}
void showAll() {
cout <<"Altitude = " << getAbsoluteAltitude() << endl;
cout <<"Speed = " << getSpeed() << endl;
cout <<"FuelLevel = " << getFuelLevel() << endl;
cout <<"Direction = " << getDirection() << endl;
}
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

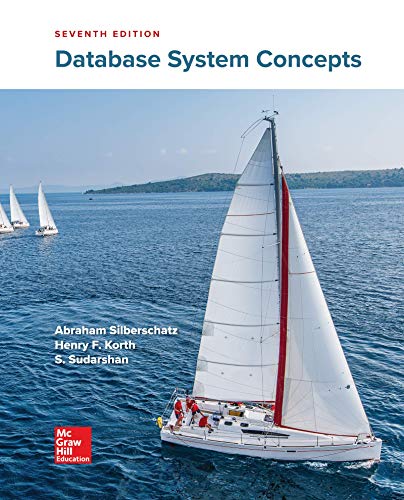
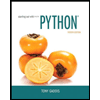
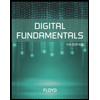
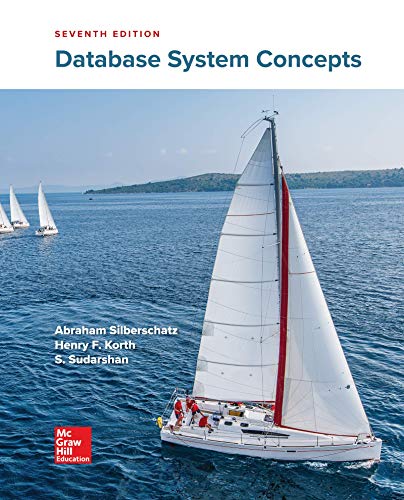
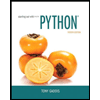
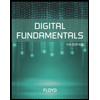
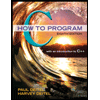
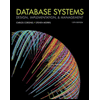
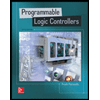