C++ code: How I can make it stop repeating the question "how many gigabytes of data used? When entering an invalid input? I also have the same issue when entering an invalid package it repeats the pick package option again. When entering a letter instead of a number it goes crazy and spams the package questions multiple times at once. My professor also said that I need Fixed, showpoint, and setprecision in my code. I provided pictures of the errors that have been happening.
C++ code: How I can make it stop repeating the question "how many gigabytes of data used? When entering an invalid input? I also have the same issue when entering an invalid package it repeats the pick package option again. When entering a letter instead of a number it goes crazy and spams the package questions multiple times at once. My professor also said that I need Fixed, showpoint, and setprecision in my code. I provided pictures of the errors that have been happening.
Heres the code:
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
int main()
{
//variables and constants
int packageType;
double dataUsed;
double monthlyCharges = 0.0;
// Name of the program
cout << "iMobile Bill Calculator ..." << endl;
// Display the pacakge options for user to pick
while (true)
{
cout << "\nSelect a subscription :" << endl;
cout << "\t\t1. Package A" << endl;
cout << "\t\t2. Package B" << endl;
cout << "\t\t3. Package C" << endl;
cout << "Package: ";
cin >> packageType;
switch (packageType)
{
// Monthtly Charges for Plan A
case 1:
{
// When Gigabytes entered is not valid
while (true)
{
cout << "\nHow many gigabytes of data were used? ";
cin >> dataUsed;
if (dataUsed < 0)
{
cout << "Error ... Invalid gigabytes entered. Try again." << endl;
}
else
break;
}
if (dataUsed >= 0 && dataUsed <= 4)
{
monthlyCharges = 39.99;
}
else
// Monthtly Charges for Plan A with additional data costs
{
monthlyCharges = 39.99 + (dataUsed - 4) * 10;
}
// Display the monthly total amount
cout << "The total amount due is $" << monthlyCharges << endl;
break;
}
// Monthtly Charges for Plan B
case 2:
{
// When Gigabytes entered is not valid
while (true)
{
cout << "\nHow many gigabytes of data were used? ";
cin >> dataUsed;
if (dataUsed < 0)
{
cout << "Error ... Invalid gigabytes entered. Try again." << endl;
}
else
break;
}
if (dataUsed >= 0 && dataUsed <= 8)
{
monthlyCharges = 59.99;
}
// Monthtly Charges for Plan B with additional data costs
else
{
monthlyCharges = 59.99 + (dataUsed - 8) * 5;
}
// Display the monthly total amount
cout << "The total amount due is $" << monthlyCharges << endl;
break;
}
// Monthly Charges for Plan C
case 3:
{
monthlyCharges = 75;
cout << "The total amount due is $" << monthlyCharges << endl;
break;
}
// When a package number is not vaild
default:
cout << "Error ... Invalid package. Try again." << endl;
}
if (packageType > 0 && packageType <= 3)
{
break;
}
}
return 0;
}



I have given errorless code below.
Step by step
Solved in 2 steps with 3 images

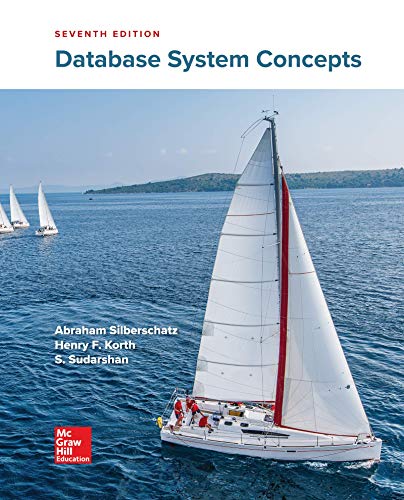
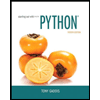
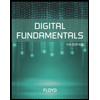
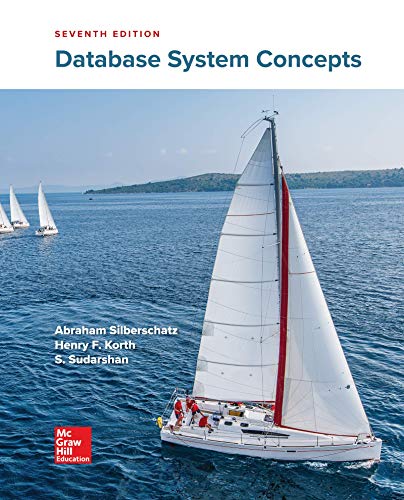
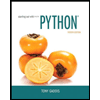
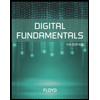
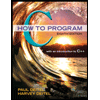
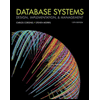
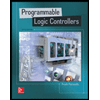