a Programming exercise: Create a class called Collatz Create the main method Inside, create these variables: Create an int called collatzNum and assign it any positive integer from 1-100 (Please refer to the PE Clarification Thread on Ed for example output.) Create an int called numSteps and assign it 0. The number of “steps” corresponds to the number of times you apply one of the operations. Create an int called highestNumReached and assign it the value collatzNum Create an int called initValue and assign it the value collatzNum Print Create a while loop that terminates when collatzNum is equal to 1 6. Within the while loop, use an if-else statement to do the following: If collatzNum is even, collatzNum should be assigned half of its current value Otherwise, (if it’s odd), collatzNum should be three times its value plus one. Check whether the new collatzNum is greater than If so, update its value to collatzNum Print out collatzNum and add 1 to numSteps Close the while loop After the while loop, use three print statements to output: “Initial value: [initValue]” “Number of steps: [numSteps]” “Highest number reached: [highestNumReached]” Finally, use a switch statement to print one of the following statements according to the value of numSteps: 0 steps: “No steps required” 1 step: “Only took one step!” 2 steps: “Two steps” 3 steps: “Three steps” 4 steps: “Four steps” All other amounts: “Wow, [numSteps] steps was a lot of steps!” (For example, if 8 steps were taken, “Wow, 8 steps was a lot of steps!” should be printed without the quotes.) Example Outputs Please refer to the PE clarification thread on Ed for examples. HINT: To help debug your code, try inserting print statements in places where variables are changed. My Code:
Please help me debug my code. Thank you!
Java Programming exercise:
- Create a class called Collatz
- Create the main method
- Inside, create these variables:
- Create an int called collatzNum and assign it any positive integer from 1-100 (Please refer to the PE Clarification Thread on Ed for example output.)
- Create an int called numSteps and assign it 0. The number of “steps” corresponds to the number of times you apply one of the operations.
- Create an int called highestNumReached and assign it the value collatzNum
- Create an int called initValue and assign it the value collatzNum
- Create a while loop that terminates when collatzNum is equal to 1 6. Within the while loop, use an if-else statement to do the following:
- If collatzNum is even, collatzNum should be assigned half of its current value
- Otherwise, (if it’s odd), collatzNum should be three times its value plus one.
- Check whether the new collatzNum is greater than If so, update its value to collatzNum
- Print out collatzNum and add 1 to numSteps
- Close the while loop
- After the while loop, use three print statements to output:
- “Initial value: [initValue]”
- “Number of steps: [numSteps]”
- “Highest number reached: [highestNumReached]”
- Finally, use a switch statement to print one of the following statements according to the value of numSteps:
- 0 steps: “No steps required”
- 1 step: “Only took one step!”
- 2 steps: “Two steps”
- 3 steps: “Three steps”
- 4 steps: “Four steps”
- All other amounts: “Wow, [numSteps] steps was a lot of steps!” (For example, if 8 steps were taken, “Wow, 8 steps was a lot of steps!” should be printed without the quotes.)
Example Outputs
Please refer to the PE clarification thread on Ed for examples.
HINT: To help debug your code, try inserting print statements in places where variables are changed.
My Code:
public class Collatz {
/**
* Main mehtod.
*/
public static void main(String[] args){
/**
*@param numStep.
*/
Random r = new Random();
int collatzNum = r.nextInt(100) + 1;
int numSteps = 0;
int highestNumReached = collatzNum;
int initValue = collatzNum;
/**
* Prints collatzNum.
*/
System.out.println(collatzNum);
/**
*
* While loop that computes equation.
*/
while (collatzNum != 1) {
if (collatzNum %= 2)
collatzNum = collatzNum * 2;
}
else if (collatzNum %= 1){
collatzNum = 3 * collatzNum + 1;
}
else (collatzNum > highestNumReached) {
highestNumReached = collatzNum;
}
System.out.println(collatzNum);
numSteps ++;
}
/**
* Printing statments.
*/
System.out.println("Initial Value: " + initValue);
System.out.println("Number of steps: " + numSteps);
System.out.println("Highest number reached: " + highestNumReached);
/**
*
*@param numSteps
* Switch statement for numSteps.
*/
switch (numSteps) {
case 0:
System.out.println("No steps required!");
break;
case 1:
System.out.println("Only took one step!");
break;
case 2:
System.out.println("Two steps!");
break;
case 3:
System.out.println("Three steps!");
break;
case 4:
System.out.println("Four steps!");
break;
default:
System.out.println("Wow, " + numSteps + " steps was a lot of steps!");
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

How would I do the loop without importing anything? I'm not supposed to import any packages for this exercise. Thank you
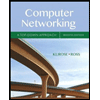
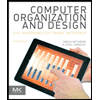
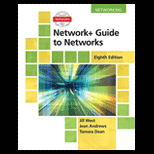
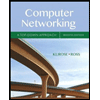
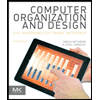
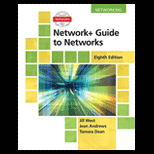
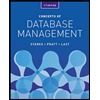
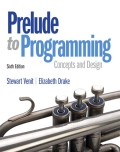
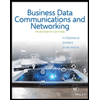