BST Programming Challenges A - Provide your implementation for all the functions in the following exercises, as well as your implementation of main() in ww3.cp. - Adding private functions (in BST.h only) is allowed but modifying anything else is not allowed - Do not add or remove any files. Ex1. DLL2BST (15 points) Add a constructor that constructs the binary search tree from the given DLList. BST::BST(const DLL& list) Ex2. Find the Second Minimum (25 points) Write a member function called int BST::Find_Second_Minimum() const. The function retrieves the value of the node that has the second minimum value in the current BST. The second minimum is the element just greater than the minimum number. The running time of your implementation should be o(h). Ex3. Get the Longest Path (25 points)
BST Programming Challenges A - Provide your implementation for all the functions in the following exercises, as well as your implementation of main() in ww3.cp. - Adding private functions (in BST.h only) is allowed but modifying anything else is not allowed - Do not add or remove any files. Ex1. DLL2BST (15 points) Add a constructor that constructs the binary search tree from the given DLList. BST::BST(const DLL& list) Ex2. Find the Second Minimum (25 points) Write a member function called int BST::Find_Second_Minimum() const. The function retrieves the value of the node that has the second minimum value in the current BST. The second minimum is the element just greater than the minimum number. The running time of your implementation should be o(h). Ex3. Get the Longest Path (25 points)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
#pragma once
template <class T> class BST;
template <class T>
class BSTNode{
private:
T val;
BSTNode* left;
BSTNode* right;
int depth;
int height;
friend class BST<T>;
public:
BSTNode(const T& v, BSTNode* left, BSTNode* right);
~BSTNode(){};
T get_val() { return val;}
BSTNode* getLeft() { return left; }
BSTNode* getRight(){ return right; }
};
template <class T>
BSTNode<T>::BSTNode(const T& v, BSTNode* left, BSTNode* right){
val = v;
this->left= left;
this->right=right;
depth = height= -1; // Not computed yet
}
can you implement these following codes in the pictures please

Transcribed Image Text:BST Programming Challenges
- Provide your implementation for all the functions in the following exercises, as well as your implementation
of main() in HW3.cpp .
- Adding private functions (in BST.h only) is allowed but modifying anything else is not allowed
- Do not add or remove any files.
Ex1. DLL2BST (15 points)
Add a constructor that constructs the binary search tree from the given DLList.
BST<T>::BST(const DLL<T>& list)
Ex2. Find the Second Minimum (25 points)
Write a member function called int BST<int>::Find_Second_Minimum() const. The function
retrieves the value of the node that has the second minimum value in the current BST. The second
minimum is the element just greater than the minimum number. The running time of your
implementation should be o(h).
Ex3. Get the Longest Path (25 points)
Write a member function called DLList<T> BST<T>:: get_longest_path() that returns a DLL of
the longest path in the tree. For example, the red nodes in the following tree are on the longest path
and should be added to the list. In case there are multiple longest paths, retrieve any of them.

Transcribed Image Text:Ex4. Extract SubTree (25 points)
Write a BST member function BST<T> BST<T>::Copy_subTree(const T& n) that will extract and
return the subtree rooted at the node containing the value n.
For example, on the following tree, the function should return the subtree shown to the right.
The current tree
The returned BST
The current Tree after calling the function
12
12
30
30
9 14
39
39
Ex5. New Traverse (Bonus 20 points)
Write a BST member function void BST<T>::BFT() that will print the tree elements in the way that
is shown in the next figure
100
20
(200
10
30
(150
(300)
Ex6. Main (10 points)
Write a main() function that tests the functions you implemented in exercises 1-5.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Recommended textbooks for you
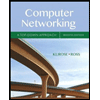
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
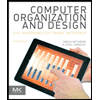
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
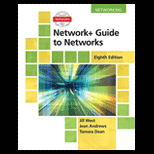
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
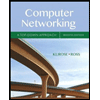
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
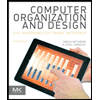
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
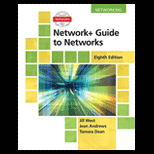
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
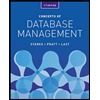
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
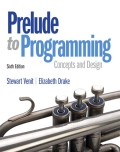
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
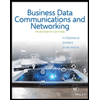
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY