Below is interpreter.java & there's errors in the code, so make sure to fix those errors. Attached is information of what the code must have. Interpreter.java import java.util.*; public class Interpreter { privateHashMap variables; publicvoidinterpretFunction(FunctionNode fn){ variables = new HashMap(); for(Node constantNode : fn.getConstants()){ Object constant = constantNode.getValue(); variables.put(constantNode.getName(), constant); } for(Node localVarNode : fn.getVariables()){ Object localVar =null; variables.put(localVarNode.getName(), localVar); } interpretBlock(fn.getStatements(), variables); } publicvoidinterpretBlock(List statementNodes,HashMap variables){ for(StatementNode statement : statementNodes){ if(statement instanceofIfNode){ interpretIfNode((IfNode) statement, variables); }elseif(statement instanceofVariableReferenceNode){ interpretVariableReferenceNode((VariableReferenceNode) statement, variables); }elseif(statement instanceofMathOpNode){ interpretMathOpNode((MathOpNode) statement, variables); }elseif(statement instanceofBooleanCompareNode){ interpretBooleanCompareNode((BooleanCompareNode) statement, variables); }elseif(statement instanceofForNode){ interpretForNode((ForNode) statement, variables); }elseif(statement instanceofRepeatNode){ interpretRepeatNode((RepeatNode) statement, variables); }elseif(statement instanceofConstantNode){ interpretConstantNode((ConstantNode) statement, variables); }elseif(statement instanceofWhileNode){ interpretWhileNode((WhileNode) statement, variables); }elseif(statement instanceofAssignmentNode){ interpretAssignmentNode((AssignmentNode) statement, variables); }elseif(statement instanceofFunctionCallNode){ interpretFunctionCallNode((FunctionCallNode) statement, variables); } } } publicvoidinterpretIfNode(IfNode ifNode,HashMap variables){ Object result =expression(ifNode.getBooleanCompare(), variables); if(result !=null&&(result instanceofBoolean&&(Boolean) result)){ interpretBlock(ifNode.getStatements(), variables); }elseif(ifNode.getNextIfNode()!=null){ interpretIfNode(ifNode.getNextIfNode(), variables); } } publicObjectinterpretVariableReferenceNode(VariableReferenceNode varRefNode,HashMap variables){ String name = varRefNode.getName(); if(variables.containsKey(name)){ return variables.get(name); }else{ thrownewRuntimeException("Variable '"+ name +"' does not exist."); } } publicObjectinterpretMathOpNode(MathOpNode mathOpNode,HashMap variables){ Object leftNode =expression(mathOpNode.getLeft(), variables); Object rightNode =expression(mathOpNode.getRight(), variables); if(!(leftNode instanceofDouble)||!(rightNode instanceofDouble)){ thrownewRuntimeException("Cannot add/subtract/multiply/divide/modulo "+ leftNode +" and "+ rightNode); } Double left =(Double) leftNode; Double right =(Double) rightNode; Object result =null; switch(mathOpNode.getOp()){ case"+": result = left + right; break; case"-": result = left - right; break; case"*": result = left * right; break; case"/": result = left / right; break; case"%": result = left % right; break; default: thrownewRuntimeException(); } } }
Below is interpreter.java & there's errors in the code, so make sure to fix those errors. Attached is information of what the code must have. Interpreter.java import java.util.*; public class Interpreter { privateHashMap variables; publicvoidinterpretFunction(FunctionNode fn){ variables = new HashMap(); for(Node constantNode : fn.getConstants()){ Object constant = constantNode.getValue(); variables.put(constantNode.getName(), constant); } for(Node localVarNode : fn.getVariables()){ Object localVar =null; variables.put(localVarNode.getName(), localVar); } interpretBlock(fn.getStatements(), variables); } publicvoidinterpretBlock(List statementNodes,HashMap variables){ for(StatementNode statement : statementNodes){ if(statement instanceofIfNode){ interpretIfNode((IfNode) statement, variables); }elseif(statement instanceofVariableReferenceNode){ interpretVariableReferenceNode((VariableReferenceNode) statement, variables); }elseif(statement instanceofMathOpNode){ interpretMathOpNode((MathOpNode) statement, variables); }elseif(statement instanceofBooleanCompareNode){ interpretBooleanCompareNode((BooleanCompareNode) statement, variables); }elseif(statement instanceofForNode){ interpretForNode((ForNode) statement, variables); }elseif(statement instanceofRepeatNode){ interpretRepeatNode((RepeatNode) statement, variables); }elseif(statement instanceofConstantNode){ interpretConstantNode((ConstantNode) statement, variables); }elseif(statement instanceofWhileNode){ interpretWhileNode((WhileNode) statement, variables); }elseif(statement instanceofAssignmentNode){ interpretAssignmentNode((AssignmentNode) statement, variables); }elseif(statement instanceofFunctionCallNode){ interpretFunctionCallNode((FunctionCallNode) statement, variables); } } } publicvoidinterpretIfNode(IfNode ifNode,HashMap variables){ Object result =expression(ifNode.getBooleanCompare(), variables); if(result !=null&&(result instanceofBoolean&&(Boolean) result)){ interpretBlock(ifNode.getStatements(), variables); }elseif(ifNode.getNextIfNode()!=null){ interpretIfNode(ifNode.getNextIfNode(), variables); } } publicObjectinterpretVariableReferenceNode(VariableReferenceNode varRefNode,HashMap variables){ String name = varRefNode.getName(); if(variables.containsKey(name)){ return variables.get(name); }else{ thrownewRuntimeException("Variable '"+ name +"' does not exist."); } } publicObjectinterpretMathOpNode(MathOpNode mathOpNode,HashMap variables){ Object leftNode =expression(mathOpNode.getLeft(), variables); Object rightNode =expression(mathOpNode.getRight(), variables); if(!(leftNode instanceofDouble)||!(rightNode instanceofDouble)){ thrownewRuntimeException("Cannot add/subtract/multiply/divide/modulo "+ leftNode +" and "+ rightNode); } Double left =(Double) leftNode; Double right =(Double) rightNode; Object result =null; switch(mathOpNode.getOp()){ case"+": result = left + right; break; case"-": result = left - right; break; case"*": result = left * right; break; case"/": result = left / right; break; case"%": result = left % right; break; default: thrownewRuntimeException(); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Below is interpreter.java & there's errors in the code, so make sure to fix those errors. Attached is information of what the code must have.
Interpreter.java
import java.util.*;
public class Interpreter {
privateHashMap<String, Object> variables;
publicvoidinterpretFunction(FunctionNode fn){
variables = new HashMap<String, Object>();
for(Node constantNode : fn.getConstants()){
Object constant = constantNode.getValue();
variables.put(constantNode.getName(), constant);
}
for(Node localVarNode : fn.getVariables()){
Object localVar =null;
variables.put(localVarNode.getName(), localVar);
}
interpretBlock(fn.getStatements(), variables);
}
publicvoidinterpretBlock(List<StatementNode> statementNodes,HashMap<String, Object> variables){
for(StatementNode statement : statementNodes){
if(statement instanceofIfNode){
interpretIfNode((IfNode) statement, variables);
}elseif(statement instanceofVariableReferenceNode){
interpretVariableReferenceNode((VariableReferenceNode) statement, variables);
}elseif(statement instanceofMathOpNode){
interpretMathOpNode((MathOpNode) statement, variables);
}elseif(statement instanceofBooleanCompareNode){
interpretBooleanCompareNode((BooleanCompareNode) statement, variables);
}elseif(statement instanceofForNode){
interpretForNode((ForNode) statement, variables);
}elseif(statement instanceofRepeatNode){
interpretRepeatNode((RepeatNode) statement, variables);
}elseif(statement instanceofConstantNode){
interpretConstantNode((ConstantNode) statement, variables);
}elseif(statement instanceofWhileNode){
interpretWhileNode((WhileNode) statement, variables);
}elseif(statement instanceofAssignmentNode){
interpretAssignmentNode((AssignmentNode) statement, variables);
}elseif(statement instanceofFunctionCallNode){
interpretFunctionCallNode((FunctionCallNode) statement, variables);
}
}
}
publicvoidinterpretIfNode(IfNode ifNode,HashMap<String, Object> variables){
Object result =expression(ifNode.getBooleanCompare(), variables);
if(result !=null&&(result instanceofBoolean&&(Boolean) result)){
interpretBlock(ifNode.getStatements(), variables);
}elseif(ifNode.getNextIfNode()!=null){
interpretIfNode(ifNode.getNextIfNode(), variables);
}
}
publicObjectinterpretVariableReferenceNode(VariableReferenceNode varRefNode,HashMap<String, Object> variables){
String name = varRefNode.getName();
if(variables.containsKey(name)){
return variables.get(name);
}else{
thrownewRuntimeException("Variable '"+ name +"' does not exist.");
}
}
publicObjectinterpretMathOpNode(MathOpNode mathOpNode,HashMap<String, Object> variables){
Object leftNode =expression(mathOpNode.getLeft(), variables);
Object rightNode =expression(mathOpNode.getRight(), variables);
if(!(leftNode instanceofDouble)||!(rightNode instanceofDouble)){
thrownewRuntimeException("Cannot add/subtract/multiply/divide/modulo "+ leftNode +" and "+ rightNode);
}
Double left =(Double) leftNode;
Double right =(Double) rightNode;
Object result =null;
switch(mathOpNode.getOp()){
case"+":
result = left + right;
break;
case"-":
result = left - right;
break;
case"*":
result = left * right;
break;
case"/":
result = left / right;
break;
case"%":
result = left % right;
break;
default:
thrownewRuntimeException();
}
}
}

Transcribed Image Text:Rubric
Comments
Variable/Function
naming
interpretFunction
interpretBlock
expression
booleanCompare
variableReferenceNode
mathOpNode
ifNode
forNode
repeatNode
constantNodes
whileNode
assignmentNode
Poor
None/Excessive
(0)
Single letters
everywhere (0)
Not handled (0)
Not handled (0)
Not handled (0)
Not handled (0)
Not handled (0)
Not handled(0)
Not handled(0)
Not handled (0)
Not handled (0)
Not handled (0)
Not handled (0)
Not handled (0)
OK
"What" not
"Why", few (5)
Lots of
abbreviations (5)
Good
Some "what" comments
or missing some (7)
Full words most of the
time (8)
Great
Anything not obvious has reasoning
(10)
Full words, descriptive (10)
Creates variables and calls
interpretBlock (10)
Loops over the statement nodes and
calls methods for each (5)
Handles mathOpNode,
constantNodes, variable references
(10)
Calls expression(), then compares (5)
Looks up nodes and returns IDT (5)
Calls expression (), then calculates (5)
Calls booleanCompare and chains (10)
Loops over the range and calls
interpretBlock(10)
Calls booleanCompare and
interpretBlock correctly (5)
Returns a new IDT (5)
Calls booleanCompare and
interpretBlock correctly (5)
calls expression() and replaces the IDT
entry for the variable(5)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I ran the code and there are lots of errors in the code. Attached are images of the errors. Make sure to fix those errors and there must be no error in the code at all.
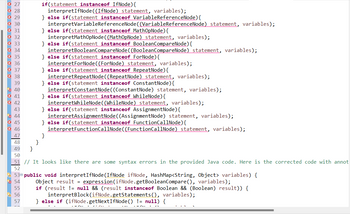
Transcribed Image Text:27
43
5 44 45 46 48 49 5
45
47
if(statement instanceof IfNode) {
interpretIfNode ((IfNode) statement, variables);
} else if (statement instanceof VariableReferenceNode) {
interpretVariable ReferenceNode ((VariableReferenceNode) statement, variables);
} else if (statement instanceof MathOpNode) {
56
57
interpretMathOpNode ((MathOpNode) statement, variables);
} else if(statement instanceof BooleanCompareNode) {
interpretBooleanCompareNode((BooleanCompareNode) statement, variables);
} else if (statement instanceof ForNode) {
interpretForNode((ForNode) statement, variables);
} else if(statement instanceof RepeatNode) {
interpretRepeatNode ((RepeatNode) statement, variables);
} else if(statement instanceof ConstantNode) {
interpretConstantNode((ConstantNode) statement, variables);
} else if (statement instanceof WhileNode) {
interpretWhileNode((WhileNode) statement, variables);
} else if (statement instanceof AssignmentNode) {
interpretAssignmentNode((AssignmentNode) statement, variables);
} else if(statement instanceof FunctionCallNode) {
interpretFunctionCallNode((FunctionCallNode) statement, variables);
}
50
51 // It looks like there are some syntax errors in the provided Java code. Here is the corrected code with annot
52
530 public void interpretIfNode (IfNode ifNode, HashMap<String, Object> variables) {
54
Object result = expression (ifNode.getBooleanCompare(), variables);
if (result != null && (result instanceof Boolean && (Boolean) result)) {
interpretBlock (ifNode.getStatements(), variables);
} else if (ifNode.getNextIfNode() != null) {
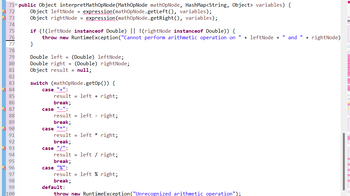
Transcribed Image Text:71 public Object interpretMathOpNode (MathOpNode mathOpNode, HashMap<String, Object> variables) {
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
Object leftNode = expression (mathOpNode.getLeft(), variables);
Object rightNode = expression (mathOpNode.getRight(), variables);
if (!(leftNode instanceof Double) || !(rightNode instanceof Double)) {
11
throw new RuntimeException("Cannot perform arithmetic operation on + leftNode + " and " + rightNode)
}
Double left = (Double) leftNode;
Double right = (Double) rightNode;
Object result = null;
switch (mathOpNode.get0p()) {
case "+":
result = left + right;
break;
case "_".
result = left - right;
break;
"*".
case
result = left * right;
break;
case :
result = left / right;
break;
case "%".
result = left % right;
break;
default:
throw new RuntimeException ("Unrecognized arithmetic operation");
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
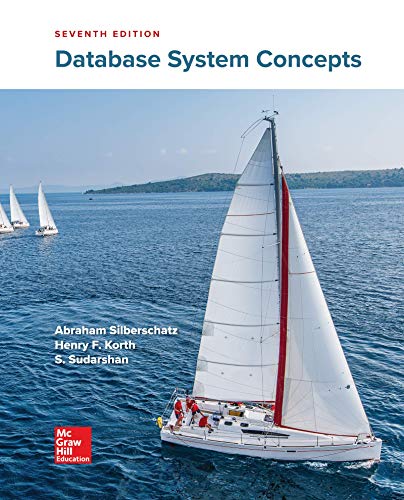
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
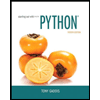
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
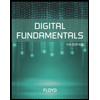
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
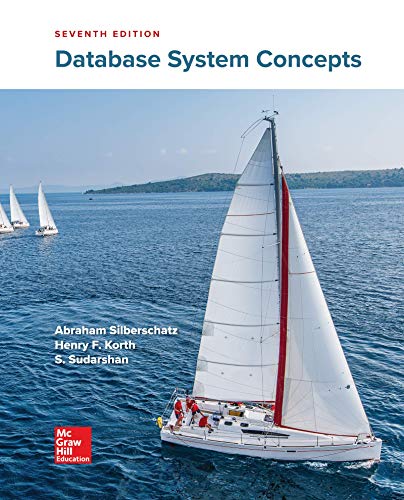
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
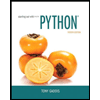
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
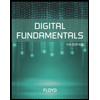
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
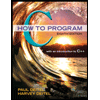
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
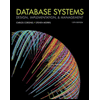
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
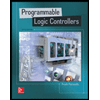
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education