ANSWER IN JAVA STANDARD OUTPUT: Enter·length·of·rectangle:Enter·width·of·rectangle:Area:·22.5,·Perimeter:·21.0↵ Create a class Rectangle with double attributes length and width. The default constructor should set these attributes to 1. Provide methods that calculate the rectangle's perimeter and area, as well as accessors and mutators for both data fields. The mutator methods for length and width should verify that the number being passed in is larger than 0.0 and less than 20.0 -- if it doesn't fit those criteria, the value of the field should not be changed. Write a Driver class in the same file to test your Rectangle class. It should prompt the user to enter a length and width of a rectangle, and then print out the area and perimeter of the rectangle. (Use the mutators to set the length and width of the rectangle, not the constructor.)
ANSWER IN JAVA
STANDARD OUTPUT: Enter·length·of·rectangle:Enter·width·of·rectangle:Area:·22.5,·Perimeter:·21.0↵
Create a class Rectangle with double attributes length and width. The default constructor should set these attributes to 1. Provide methods that calculate the rectangle's perimeter and area, as well as accessors and mutators for both data fields. The mutator methods for length and width should verify that the number being passed in is larger than 0.0 and less than 20.0 -- if it doesn't fit those criteria, the value of the field should not be changed.
Write a Driver class in the same file to test your Rectangle class. It should prompt the user to enter a length and width of a rectangle, and then print out the area and perimeter of the rectangle. (Use the mutators to set the length and width of the rectangle, not the constructor.)

here
Rectangle class is made with two attributes length and width of type double.
public class Rectangle
{
double length;
double width;
Default constructor sets value to 1
Rectangle()
{
length = 1.0;
width = 1.0;
}
mutators are ther
void setLength(double l)
{ if(l<20.0 && l>0.0)
length = l;
}
double getLength()
{
return length;
}
void setWidth(double w)
{ if(w<20.0 && w>0.0)
width = w;
}
double getWidth()
{
return width;
}
methods are there for calculating area and perimeter of rectangle
double calculatePerimeter()
{
return 2 * (length + width);
}
double calculateArea()
{
return length * width;
}
main method
public static void main(String[] args) {
Rectangle r = new Rectangle();
Scanner sc = new Scanner(System.in);
System.out.println("Enter length of Rectangle:");
double length = sc.nextDouble();
System.out.println("Enter width of Rectangle:");
double width = sc.nextDouble();
r.setLength(length);
r.setWidth(width);
System.out.println("Area: "+ r.calculateArea() + ",Perimeter:" + r.calculatePerimeter());
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

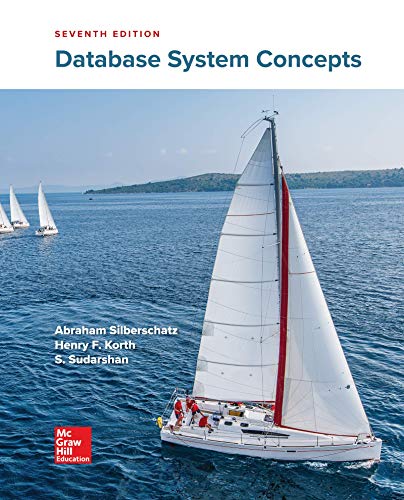
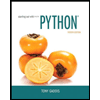
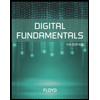
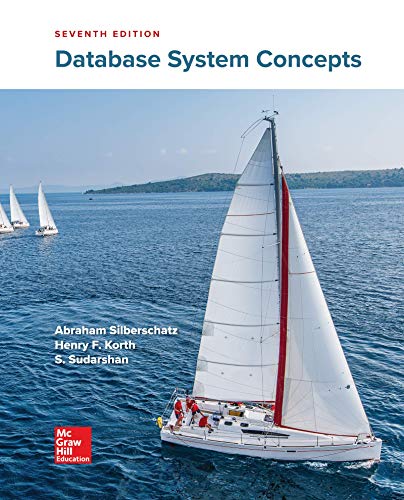
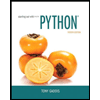
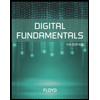
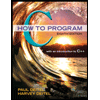
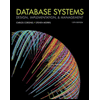
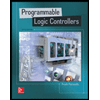