1) Write a class on BlueJ, which must be called BookClub. The class must have exactly three fields: two integer fields called capacity and occupancy and one String field called name. The capacity field will store the maximum number of shoppers allowed and occupancy will store the current number of shoppers. (There must be no additional fields defined in the class, even if you think more are required. Consider using local variables inside methods if you think you need extra fields.) The initial values of the book club name, and capacity must be received as parameters to the class's constructor, while the occupancy must always be set to zero when a BookClub object is created. The class must define getter methods for all of the fields. These must be called getName, getCapacity and getOccupancy. You must not call them anything different. You must not write setter methods for the fields. The class must define a method called addOne that takes no parameters. If the current number of shoppers is less than the club's capacity then the occupancy must be increased by 1, which means that a new person has been allowed to enter the club. Otherwise, the following message must be printed and the current number of shoppers must not be changed: The club is full.
1) Write a class on BlueJ, which must be called BookClub. The class must have exactly three fields: two integer fields called capacity and occupancy and one String field called name. The capacity field will store the maximum number of shoppers allowed and occupancy will store the current number of shoppers. (There must be no additional fields defined in the class, even if you think more are required. Consider using local variables inside methods if you think you need extra fields.) The initial values of the book club name, and capacity must be received as parameters to the class's constructor, while the occupancy must always be set to zero when a BookClub object is created. The class must define getter methods for all of the fields. These must be called getName, getCapacity and getOccupancy. You must not call them anything different. You must not write setter methods for the fields. The class must define a method called addOne that takes no parameters. If the current number of shoppers is less than the club's capacity then the occupancy must be increased by 1, which means that a new person has been allowed to enter the club. Otherwise, the following message must be printed and the current number of shoppers must not be changed: The club is full.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
answer question 1 in blueJ java format

Transcribed Image Text:1) Write a class on BlueJ, which must be called BookClub. The class must have exactly three fields: two integer fields called capacity and occupancy and one String field
called name. The capacity field will store the maximum number of shoppers allowed and occupancy will store the current number of shoppers. (There must be no additional
fields defined in the class, even if you think more are required. Consider using local variables inside methods if you think you need extra fields.) The initial values of
the book club name, and capacity must be received as parameters to the class's constructor, while the occupancy must always be set to zero when a BookClub object is
created. The class must define getter methods for all of the fields. These must be called getName, getCapacity and getOccupancy. You must not call them anything different.
You must not write setter methods for the fields. The class must define a method called addOne that takes no parameters. If the current number of shoppers is less than the
club's capacity then the occupancy must be increased by 1, which means that a new person has been allowed to enter the club. Otherwise, the following message must be
printed and the current number of shoppers must not be changed: The club is full.
2) write if the current number of shoppers is greater than or equal to the number leaving then the number of shoppers is decreased by the number leaving. Otherwise, the
following error message must be printed and the number of shoppers present must be set to 0. There is an error in the number of shoppers. Use System.out.println to print
the message. The class must define a method called leaving that takes a single integer parameter. This represents the number of shoppers leaving the club at a particular
point. You may assume that the parameter's value will always be greater than zero and you do not have to check for that. If the current number of clubbers is greater than
or equal to the number leaving then the number of shoppers is decreased by the number leaving. Otherwise, the following error message must be printed and the number of
clubbers present must be set to 0. There is an error in the number of shoppers. Use System.out.println to print the error message. The class must define a method called is
Space that allows the book club staff to determine whether there is enough capacity for a group to attend. This method must take a single integer parameter representing
the size of the group, and return a boolean result. The method must work as follows: If the value of the parameter is less-than or equal-to then the method must return
false. This case has priority over those following. If the value of the parameter is less-than or equal-to the space left in the book club (use the capacity and occupancy
values in the to work this out) then the method must return true. Otherwise (i.e., if there is not space in the book club for the whole group) then the method must return
false. (Note that the method must only return true if there is space for the whole group to attend. This method must not change the state of the BookClub object. In other
words, both the current number of occupants and the capacity of the club must be exactly the same after it is called as it was before.)
3) The class must define a method called isSpace that allows the book club staff to determine whether there is enough capacity for a group to attend. This method must take
a single integer parameter representing the size of the group, and return a boolean result. The method must work as follows: If the value of the parameter is less-than or
equal-to then the method must return false. This case has priority over those following. If the value of the parameter is less-than or equal-to the space left in the
book club (use the capacity and occupancy values in the to work this out) then the method must return true. Otherwise (i.e., if there is not space in the book club for the
whole group) then the method must return false. This method must not change the state of the BookClub object. In other words, both the current number of occupants and the
capacity of the club must be exactly the same after it is called as it was before (Note that the return type of this method must be boolean. The method has to return
either true or false and that does not mean that it should print or return the strings "true" or "false). The class must define a method called printDetails that prints
the current details (using System.out.println) of the book club in the following format: The Venue: 50 shoppers. Capacity 150. Where "The Venue" is the book club's name;
50 shoppers are currently present and the club's capacity is 150. Of course, different BookClub objects will have different names, number of clubbers and different
capacities. (Take note of the spacing between the different parts, the punctuation, and the order of the things to be printed) Write a method called about that has a void
return type and takes no parameter. When called, this must print: BookClub written by login where you must replace login with for instance: BookClub written by djb247
Expert Solution

Step 1: Algorithm for implementing the BookClub class in BlueJ, following the specified requirements:
Initialization:
- Create a class named BookClub.
- Define three fields: name (String), capacity (int), and occupancy (int).
Constructor:
- Create a constructor for the BookClub class that takes two parameters: name (String) and capacity (int).
- Initialize the name field with the value provided in the constructor.
- Initialize the capacity field with the value provided in the constructor.
- Initialize the occupancy field to 0.
Getter Methods:
- Define three getter methods: getName(), getCapacity(), and getOccupancy().
- getName() should return the value of the name field.
- getCapacity() should return the value of the capacity field.
- getOccupancy() should return the value of the occupancy field.
addOne Method:
- Define a method called addOne that takes no parameters.
- Inside the addOne method, check if the occupancy is less than the capacity.
- If it is, increment the occupancy by 1 (indicating that a new person has entered the club).
- If it's not, print the message "The club is full" using System.out.println.
Main Method (for demonstration):
- You can create a main method for testing the BookClub class.
- In the main method, create an instance of the BookClub class with initial values for name and capacity.
- Use the getter methods to retrieve the values of name, capacity, and occupancy.
- Use the addOne method to simulate people entering the club.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
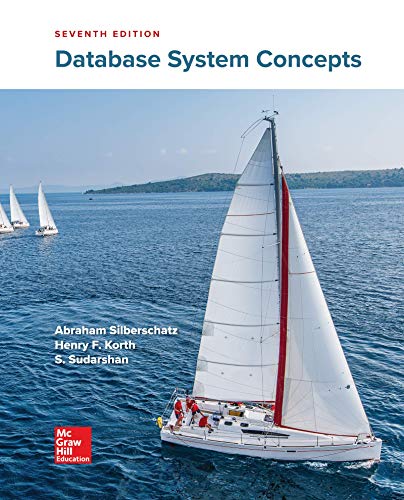
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
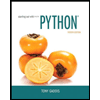
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
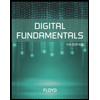
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
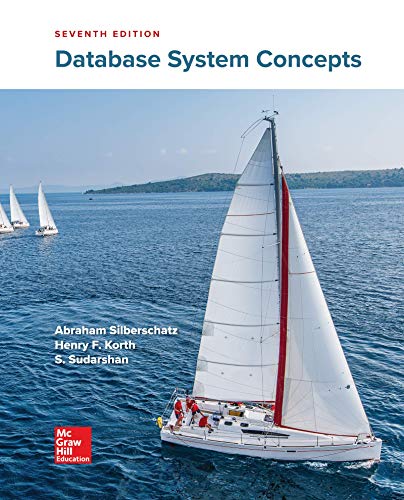
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
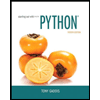
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
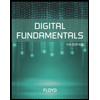
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
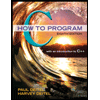
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
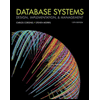
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
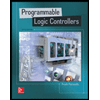
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education