Python Programming (code) Write a class named "Fraction" that represents a rational number (a number that can be expressed as the quotient of two integers). Implement the following methods: The __init__(self, numerator, denominator) method should accept integer values for the numerator and denominator arguments and set instance attributes of the same name. If the denominator is 0, raise a ZeroDivisionErrorexception. Use the [math.gcd](https://docs.python.org/3/library/ math.html#math.gcd) function to find the greatest common divisor (GCD) of the numerator and de- nominator and then divide each of them by it to "normalize" the fraction. For example, the fraction 28/20 will get normalized to 7/5 since the GCD of 28 and 20 is 4: >>> x = Fraction(28, 20) >>> x Fraction(7, 5) Implement the basic binary operators (+,-,*,/) so that a Fraction can be combined with either another fraction or an integer. All methods should return a new Fraction. Note that you may need to implement "reversed" operators for arithmetic with integers to fully work. The __neg__ method should return a new Fraction instance with the numerator negated. The __repr__ method should return a string of the form Fraction(a, b) where a and b are the numerator and denominator, respectively. -------------------------------------------------------------------------- Few test cases: >>> from fraction import Fraction >>> frac_1 = Fraction(28,20) >>> frac_ Fraction(7, 5) >>> frac_2 = Fraction(1,0) Traceback (most recent call last): raise ZeroDivisionError fraction.ZeroDivisionError: Denominator cannot be zero >>> frac_2 = Fraction(1,5) >>> frac_1 + frac_ Fraction(8, 5) >>> frac_1 * frac_ Fraction(7, 25) >>> frac_2 - frac_ Fraction(-6, 5) >>> frac_2 / frac_ Fraction(1, 7) >>> frac_2 / 2 Fraction(1, 10) >>> frac_1 / 2 Fraction(7, 10) >>> frac_1 * 2 Fraction(14, 5) >>> -frac_ Fraction(-1, 5) >>> 2 - frac_ Fraction(9, 5) >>> 2 - (-frac_2) Fraction(11, 5) -------------------------------------------------------------------------- Required Output: class Fraction: def __init__(self, numerator. denominator): pass def __neg__(): pass def __repr__(): pass
Python Programming (code)
Write a class named "Fraction" that represents a rational number (a number that can be expressed as the quotient of two integers). Implement the following methods:
- The __init__(self, numerator, denominator) method should accept integer values for the numerator and denominator arguments and set instance attributes of the same name. If the denominator is 0, raise a ZeroDivisionErrorexception. Use the [math.gcd](https://docs.python.org/3/library/ math.html#math.gcd) function to find the greatest common divisor (GCD) of the numerator and de- nominator and then divide each of them by it to "normalize" the fraction. For example, the fraction 28/20 will get normalized to 7/5 since the GCD of 28 and 20 is 4:
>>> x = Fraction(28, 20)
>>> x
Fraction(7, 5)
- Implement the basic binary operators (+,-,*,/) so that a Fraction can be combined with either another fraction or an integer. All methods should return a new Fraction. Note that you may need to implement "reversed" operators for arithmetic with integers to fully work.
- The __neg__ method should return a new Fraction instance with the numerator negated.
- The __repr__ method should return a string of the form Fraction(a, b) where a and b are the numerator and denominator, respectively.
--------------------------------------------------------------------------
Few test cases:
>>> from fraction import Fraction
>>> frac_1 = Fraction(28,20)
>>> frac_
Fraction(7, 5)
>>> frac_2 = Fraction(1,0)
Traceback (most recent call last):
raise ZeroDivisionError
fraction.ZeroDivisionError: Denominator cannot be zero
>>> frac_2 = Fraction(1,5)
>>> frac_1 + frac_
Fraction(8, 5)
>>> frac_1 * frac_
Fraction(7, 25)
>>> frac_2 - frac_
Fraction(-6, 5)
>>> frac_2 / frac_
Fraction(1, 7)
>>> frac_2 / 2
Fraction(1, 10)
>>> frac_1 / 2
Fraction(7, 10)
>>> frac_1 * 2
Fraction(14, 5)
>>> -frac_
Fraction(-1, 5)
>>> 2 - frac_
Fraction(9, 5)
>>> 2 - (-frac_2)
Fraction(11, 5)
--------------------------------------------------------------------------
Required Output:
class Fraction:
def __init__(self, numerator. denominator):
pass
def __neg__():
pass
def __repr__():
pass

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

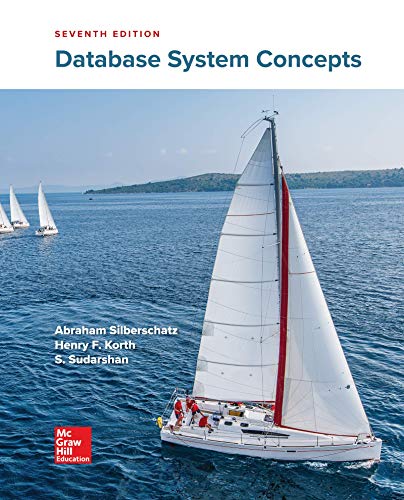
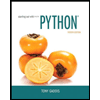
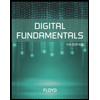
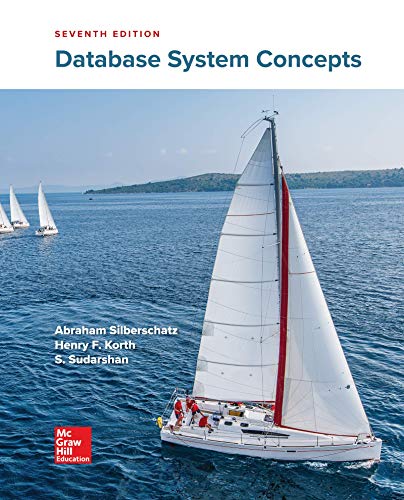
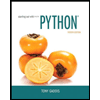
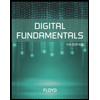
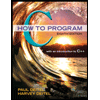
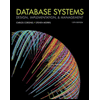
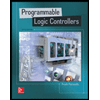