Although it will no longer be used, please leave the earlier global variable with the initialized data in the program. 1. Write a readEmployee function that takes a pointer to an employee struct and fills in the details. It should prompt the user for each field, then read the data from the user and store it in the struct passed in. Be careful to appropriately copy the string values. You do not need to worry about checking for buffer overflow. 2. Define a createEmployee function that will allocate the memory for an employee structure and return it. Before returning the pointer, it should call your readEmployee function to fill in the data. 3. Change your main function to call createEmployee and hold the pointer returned. This pointer should then be passed into your display function. 4. Write a releaseEmployee function that will free all the memory allocated for the employee whose pointer is passed in as the argument. 5. Modify main to call releaseEmployee after the display call. You main function should now include a pointer variable declaration, a call to createEmployee, a call to display and a call to releaseEmployee.
//I need help debugging the C code below the image is what I was following for directions while doing the code//
#include <stdio.h>
#include <stdlib.h>
struct employees
{
char name[20];
int ssn[9];
int yearBorn, salary;
};
// function to read the employee data from the user
void readEmployee(struct employees *emp)
{
printf("Enter name: ");
gets(emp->name);
printf("Enter ssn: ");
for (int i = 0; i < 9; i++)
scanf("%d", &emp->ssn[i]);
printf("Enter birth year: ");
scanf("%d", &emp->yearBorn);
printf("Enter salary: ");
scanf("%d", &emp->salary);
}
// function to create a pointer of employee type
struct employees *createEmployee()
{
// creating the pointer
struct employees *emp = malloc(sizeof(struct employees));
// function to read the data
readEmployee(emp);
// returning the data
return emp;
}
// function to print the employee data to console
void display(struct employees *e)
{
printf("%s", e->name);
printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0], e->ssn[1], e->ssn[2], e->ssn[3], e->ssn[4], e->ssn[5], e->ssn[6], e->ssn[7], e->ssn[8]);
printf(" %d", e->yearBorn);
printf("\n$%d.", e->salary);
}
// function to free the memory of the employee pointer
void releaseEmployee(struct employees *e)
{
free(e);
}
// main method
int main()
{
// creating the employee
struct employees *emp = createEmployee();
// printing the information
display(emp);
// free the memory
releaseEmployee(emp);
return 0;
}


Step by step
Solved in 4 steps with 1 images

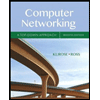
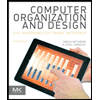
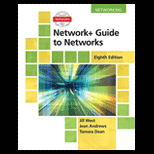
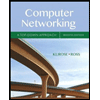
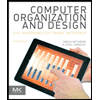
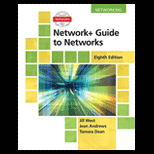
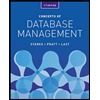
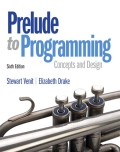
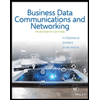