After I wrote and run my code: import java.util.Scanner; /** * Activity1PayStub class is part of Lab 3 and * creates a simple pay stub. * * @author Ahadu Abera * @version 9/27/22 */ public class Activity1PayStub { // constants public static final double OVERTIME_RATE = 1.5; public static final double HOLD = .1; public static final double TAX = .2; // Object used to access the methods public static void main(String[] args) { // Creates an object of Activity1PayStub Scanner sc = new Scanner(System.in); System.out.print("Name: "); String name = sc.nextLine(); System.out.print("SSN: "); String ssn = sc.nextLine(); int regularHours; System.out.print("Regular Hours: "); regularHours = sc.nextInt(); int overtimeHours; System.out.print("Overtime Hours: "); overtimeHours = sc.nextInt(); double hourlypayRate; System.out.print("Hourly pay rate: $"); hourlypayRate = sc.nextDouble(); double regularPay = regularHours * hourlypayRate; double overtimeRate = hourlypayRate * OVERTIME_RATE; double overtimePay = overtimeHours * OVERTIME_RATE * hourlypayRate; double gross = regularPay + overtimePay; double wh = gross * HOLD; double fTax = (gross - wh) * TAX; double net = gross - wh - fTax; System.out.println("________________" + "_________________"); String format = "Name: %-37s SSN: %-11s\n"; System.out.printf(format, name, ssn); format = "Regular Hours: %-8d Reg Rate: $%-8.2f Reg Pay: $%-8.2f\n"; System.out.printf(format, regularHours, hourlypayRate, regularPay); format = "Overtime Hours: %-8dOT Rate: $%-8.2f OT Pay: $%-8.2f\n"; System.out.printf(format, overtimeHours, overtimeRate, overtimePay); format = "Gross Pay: $%-8.2f\n"; System.out.printf(format, gross); format = "SS Withholding: $%-8.2f\n"; System.out.printf(format, wh); format = "Federal Tax: $%-8.2f\n"; System.out.printf(format, fTax); format = "Net Pay: $%-8.2f\n"; System.out.printf(format, net); System.out.println("__________________" + "_____________"); } } These checkstyle errors keep showing up: 1 check style errors found.Starting audit... C:\Users\ahadu\Downloads\Lab 3\Activity1PayStub.java:0: warning: File does not end with a newline. C:\Users\ahadu\Downloads\Lab 3\Activity1PayStub.java:16:5: Missing a Javadoc comment. Audit done. How do I fix these errors so they stop showing up?
After I wrote and run my code:
import java.util.Scanner;
/**
* Activity1PayStub class is part of Lab 3 and
* creates a simple pay stub.
*
* @author Ahadu Abera
* @version 9/27/22
*/
public class Activity1PayStub
{
// constants
public static final double OVERTIME_RATE = 1.5;
public static final double HOLD = .1;
public static final double TAX = .2;
// Object used to access the methods
public static void main(String[] args)
{
// Creates an object of Activity1PayStub
Scanner sc = new Scanner(System.in);
System.out.print("Name: ");
String name = sc.nextLine();
System.out.print("SSN: ");
String ssn = sc.nextLine();
int regularHours;
System.out.print("Regular Hours: ");
regularHours = sc.nextInt();
int overtimeHours;
System.out.print("Overtime Hours: ");
overtimeHours = sc.nextInt();
double hourlypayRate;
System.out.print("Hourly pay rate: $");
hourlypayRate = sc.nextDouble();
double regularPay = regularHours * hourlypayRate;
double overtimeRate = hourlypayRate * OVERTIME_RATE;
double overtimePay = overtimeHours * OVERTIME_RATE * hourlypayRate;
double gross = regularPay + overtimePay;
double wh = gross * HOLD;
double fTax = (gross - wh) * TAX;
double net = gross - wh - fTax;
System.out.println("________________" + "_________________");
String format = "Name: %-37s SSN: %-11s\n";
System.out.printf(format, name, ssn);
format = "Regular Hours: %-8d Reg Rate: $%-8.2f Reg Pay: $%-8.2f\n";
System.out.printf(format, regularHours, hourlypayRate, regularPay);
format = "Overtime Hours: %-8dOT Rate: $%-8.2f OT Pay: $%-8.2f\n";
System.out.printf(format, overtimeHours, overtimeRate, overtimePay);
format = "Gross Pay: $%-8.2f\n";
System.out.printf(format, gross);
format = "SS Withholding: $%-8.2f\n";
System.out.printf(format, wh);
format = "Federal Tax: $%-8.2f\n";
System.out.printf(format, fTax);
format = "Net Pay: $%-8.2f\n";
System.out.printf(format, net);
System.out.println("__________________" + "_____________");
}
}
These checkstyle errors keep showing up:
1 check style errors found.Starting audit...
C:\Users\ahadu\Downloads\Lab 3\Activity1PayStub.java:0: warning: File does not end with a newline.
C:\Users\ahadu\Downloads\Lab 3\Activity1PayStub.java:16:5: Missing a Javadoc comment.
Audit done.
How do I fix these errors so they stop showing up?

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

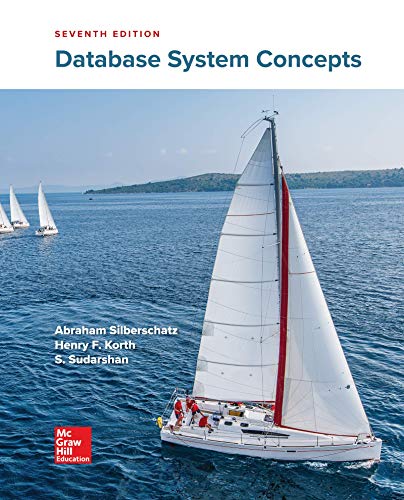
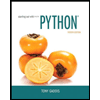
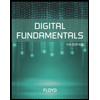
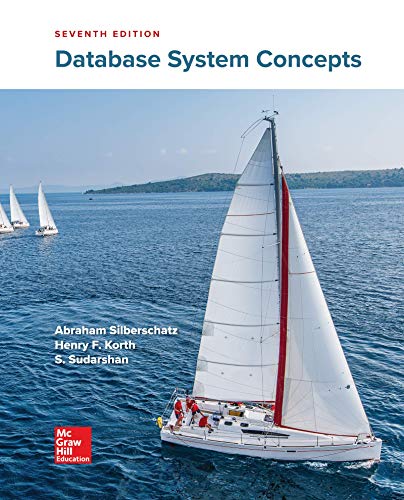
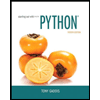
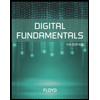
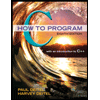
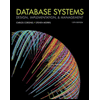
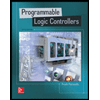