A program's code is split among two source files lib1.c and test1.c. Examine the assembly code and fill in the blanks of the C code. = lib1.s lib1.c
A program's code is split among two source files lib1.c and test1.c. Examine the assembly code and fill in the blanks of the C code. = lib1.s lib1.c
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:## Educational Content on Assembly and C Code Integration
### Overview
This example demonstrates a program whose code is divided into two source files: `lib1.c` and `test1.c`. The task is to examine the assembly code and complete the missing parts of the C code.
### Assembly Code Analysis
#### File: `lib1.s`
1. **Function `ne2`:**
- Compares the value at `%edi` with `2`.
- Sets `%al` to the result of the comparison.
- Moves the result to `%eax`.
- Returns from the function.
2. **Function `check`:**
- Compares the value at `%edi` with `1`.
- Jumps to label `.L4` if equal.
- Calls the `ne2` function.
- Tests `%eax`.
- Jumps to label `.L2` if zero.
- Moves `1` to `%eax`.
- Returns from the function.
#### File: `test1.s`
1. **Function `find`:**
- Initializes stack and registers.
- Copies argument to `%r12`.
- Compares `%ebp` with `2`.
- Jumps to label `.L7` if greater.
- Copies and scales values to `%rbx`.
- Calls the `check` function.
- Tests `%eax`.
- Updates loop index and checks conditions.
- Jumps to labels `.L2`, `.L8`, and `.L1` based on conditions.
### Corresponding C Code
#### File: `lib1.c`
```c
static int x = 1;
int error;
static int ne2(int c) {
return c _____ ; // Complete based on assembly comparison
}
int check(int c) {
return (c ____ x) ____ ne2(c); // Logic derived from comparison and assembly flow
}
```
#### File: `test1.c`
```c
extern int error;
int check(int c);
struct item {
_____ x; // Data type or field name based on assembly operations
int y;
};
long find(struct item *a) {
for (int i = 0; _______ ; i++) { // Loop condition derived from assembly index checks
if (______________________) { // Condition matching assembly test
return _________; // Return based on conditions checked
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
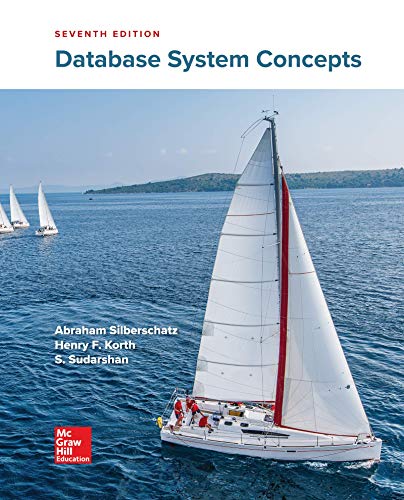
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
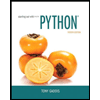
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
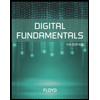
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
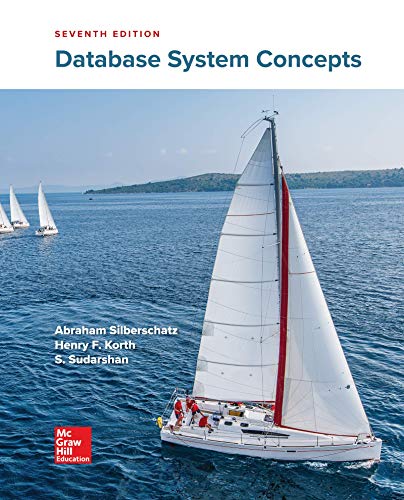
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
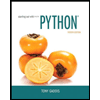
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
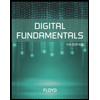
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
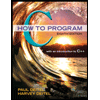
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
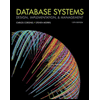
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
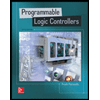
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education