1. In this part you are asked to recognize what a given segment of machine code does, by translating it into assembly code, and annotating it with expressions in a high level language like C. This process, from binary code back to source code, is called "disassembling." Disassemble the following machine code into operations and arguments, e.g., ADD R1, R2, R3. Explain what the whole program does, either in plain English (e.g., it calculates the product of R1 and R2) or in C type of pseudo code (e.g, R3 = R1 * R2.) Note: you need to make up unique labels for branch instructions in your assembly code. Addr: Code Ох00 07FF Ох02 240E Ох04 1E0C Ох06 250A Ох08 03F9 Ox0A OE00 Охос 24C2 ОхОЕ 3C00
1. In this part you are asked to recognize what a given segment of machine code does, by translating it into assembly code, and annotating it with expressions in a high level language like C. This process, from binary code back to source code, is called "disassembling." Disassemble the following machine code into operations and arguments, e.g., ADD R1, R2, R3. Explain what the whole program does, either in plain English (e.g., it calculates the product of R1 and R2) or in C type of pseudo code (e.g, R3 = R1 * R2.) Note: you need to make up unique labels for branch instructions in your assembly code. Addr: Code Ох00 07FF Ох02 240E Ох04 1E0C Ох06 250A Ох08 03F9 Ox0A OE00 Охос 24C2 ОхОЕ 3C00
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![### Instruction Set for a Simple Processor
This instruction set is supported by a simple processor, which has a few new instructions added. Its format is structured as follows:
#### Instruction Format
| bits | 15:14 | 13:10 | 9 | 8:6 | 5:3 | 2:0 |
|------|-------|--------|---|-----|-----|-----|
| field | unused | opcode | w | src1 | src2 | dst |
- **opcode**: Defines the operation to be performed by the processor.
- **w**: Determines if the ALU output should be written back to the register file (1 = yes, 0 = no).
- **src1**: Address of the first ALU operand in the register file.
- **src2**: Address of the second ALU operand in the register file.
- **dst**: Address in the register file where the output is written.
#### Special Cases for BEQ, BLEZ, and JUMP
For the opcodes BEQ, BLEZ, and JUMP, the 6 least significant bits (5:0) provide an address in the instruction memory, which is byte-addressed. The opcode HALT has all operand bits (9:0) as zero. When an instruction has only two operands, the unused operand's field is filled with zeros. For example, in the case of SLL, bits (5:3) are zero since src2 is not used.
#### Opcode and Meaning
The table below lists the opcode, binary encoding, and operations for these instructions:
| Opcode | Binary Encoding | Operation |
|--------|-----------------|----------------------------------------|
| ADD | 0x0 | R[src1] + R[src2] → R[dst] |
| SUB | 0x1 | R[src1] - R[src2] → R[dst] |
| SLL | 0x2 | R[src1] << 1 → R[dst] |
| SRL | 0x3 | R[src1] >> 1 → R[dst] |
| INV | 0x4 | ~R[src1] → R[dst] |
| XOR | 0x5 | R[src1] ^ R[src2] → R[dst] |
| OR](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F915aced1-4dd8-461c-8bbb-113909ad5fd4%2F88ccc27f-25f8-4239-8a1e-76fe94c05647%2Fcwmvtx_processed.png&w=3840&q=75)
Transcribed Image Text:### Instruction Set for a Simple Processor
This instruction set is supported by a simple processor, which has a few new instructions added. Its format is structured as follows:
#### Instruction Format
| bits | 15:14 | 13:10 | 9 | 8:6 | 5:3 | 2:0 |
|------|-------|--------|---|-----|-----|-----|
| field | unused | opcode | w | src1 | src2 | dst |
- **opcode**: Defines the operation to be performed by the processor.
- **w**: Determines if the ALU output should be written back to the register file (1 = yes, 0 = no).
- **src1**: Address of the first ALU operand in the register file.
- **src2**: Address of the second ALU operand in the register file.
- **dst**: Address in the register file where the output is written.
#### Special Cases for BEQ, BLEZ, and JUMP
For the opcodes BEQ, BLEZ, and JUMP, the 6 least significant bits (5:0) provide an address in the instruction memory, which is byte-addressed. The opcode HALT has all operand bits (9:0) as zero. When an instruction has only two operands, the unused operand's field is filled with zeros. For example, in the case of SLL, bits (5:3) are zero since src2 is not used.
#### Opcode and Meaning
The table below lists the opcode, binary encoding, and operations for these instructions:
| Opcode | Binary Encoding | Operation |
|--------|-----------------|----------------------------------------|
| ADD | 0x0 | R[src1] + R[src2] → R[dst] |
| SUB | 0x1 | R[src1] - R[src2] → R[dst] |
| SLL | 0x2 | R[src1] << 1 → R[dst] |
| SRL | 0x3 | R[src1] >> 1 → R[dst] |
| INV | 0x4 | ~R[src1] → R[dst] |
| XOR | 0x5 | R[src1] ^ R[src2] → R[dst] |
| OR

Transcribed Image Text:### Machine Code Disassembly Exercise
1. **Objective**:
This exercise involves translating a segment of machine code into assembly code and annotating it with expressions in a high-level language like C. This process is called "disassembling," where you convert binary code back to source code. Your task is to disassemble the following machine code into operations and arguments (e.g., ADD R1, R2, R3) and explain the program's function in plain English or C-like pseudocode. Unique labels should be created for branch instructions in the assembly code.
### Machine Code
```
Addr: Code
--------------------
0x00 07FE
0x02 240E
0x04 1E0C
0x06 250A
0x08 03F9
0x0A 0E00
0x0C 24C2
0x0E 3C00
```
- **Explanation Task**:
- Convert the above machine code into assembly instructions.
- Provide a high-level explanation or pseudocode of what the entire segment accomplishes (e.g., "it calculates the product of R1 and R2" or "R3 = R1 * R2").
> **Note**: Ensure to create unique labels for any branch instructions during the disassembly process.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
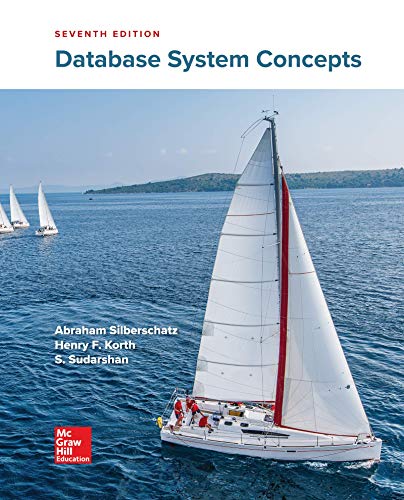
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
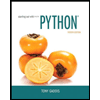
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
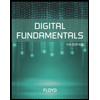
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
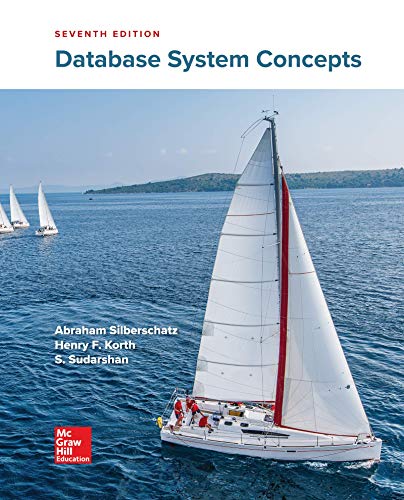
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
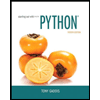
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
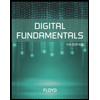
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
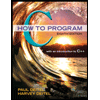
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
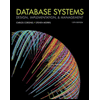
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
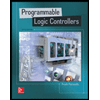
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education