A class Person is given below as your base class. Your task is to create a class Employee which will contain objects of records for an employee and derived from the class Person. Guideline for an employee record: employee's name (which is inherited from the class Person) annual salary represented (type double) year the employee started work (type int) company ID number (type String) with a format of EMPXXX (where X are numbers) Create constructors and accessor methods which you think are needed for this program, as well as another method (print) to print out all the employee’s data once you call that method. Finally, create your TestEmployee class which will contain a main method to fully test your class definition. public class Person{ private String name; public Person(){ name = "No name yet."; } public Person(String n){ name = n; } public void setName(String newName){ name = newName; } public String getName(){ return name; } public void print(){ System.out.println("Name: " + name); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
A class Person is given below as your base class. Your task is to create a class Employee which will contain objects of records for an employee and derived from the class Person.
Guideline for an employee record:
- employee's name (which is inherited from the class Person)
- annual salary represented (type double)
- year the employee started work (type int)
- company ID number (type String) with a format of EMPXXX (where X are numbers)
Create constructors and accessor methods which you think are needed for this program, as well as another method (print) to print out all the employee’s data once you call that method.
Finally, create your TestEmployee class which will contain a main method to fully test your class definition.
public class Person{
private String name;
public Person(){
name = "No name yet.";
}
public Person(String n){
name = n;
}
public void setName(String newName){
name = newName;
}
public String getName(){
return name;
}
public void print(){
System.out.println("Name: " + name);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

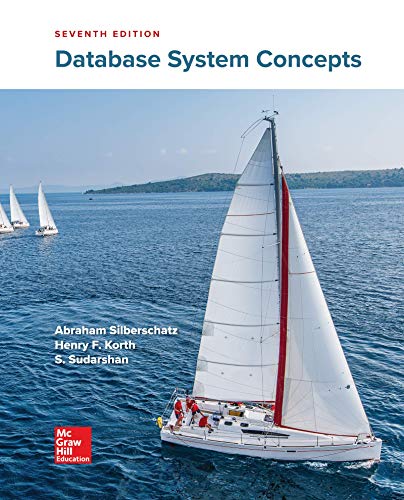
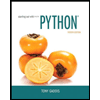
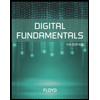
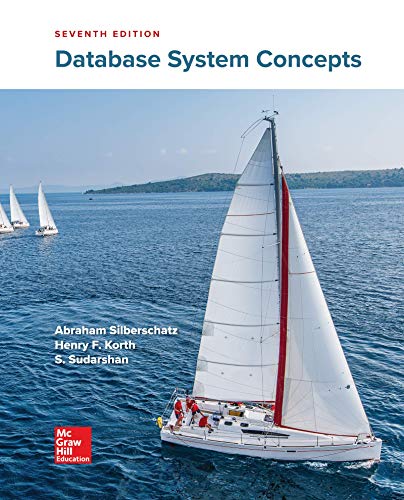
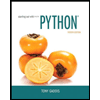
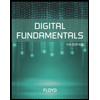
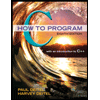
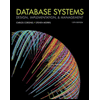
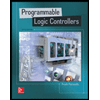