_(self): self.head = None self.tail = None def append(self, new
class Node:
def __init__(self, initial_data):
self.data = initial_data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
def prepend(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
new_node.next = self.head
self.head = new_node
def insert_after(self, current_node, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
elif current_node is self.tail:
self.tail.next = new_node
self.tail = new_node
else:
new_node.next = current_node.next
current_node.next = new_node
def remove_after(self, current_node):
if (current_node == None) and (self.head != None):
succeeding_node = self.head.next
self.head = succeeding_node
if succeeding_node == None: # Remove last item
self.tail = None
elif current_node.next != None:
succeeding_node = current_node.next.next
current_node.next = succeeding_node
if succeeding_node == None: # Remove tail
self.tail = current_node
def display(self):
node = self.head
if (node == None):
print('Empty!')
while node != None:
print(node.data, end=' ')
node = node.next
def remove_smallest_node(self):
#*** Your code goes here!***
# NOTE: this function will print out the information about the smallest node
# before removing it
def Generate_List_of_LinkedLists(n):
LLL = []
#*** Your code goes here!***
# NOTE: this function will read the input from the user for each of the lists and
# generate the list
return LLL
if __name__ == '__main__':
#*** Your code goes here!***
![19.19 LAB: Linear Search
In this lab you are asked to complete the provided code so that it generates a list of singly-linked-lists (note: this will be a python list, where
each element of the list is a singly linked list). Your code should do the following:
To generate a list of singly linked lists, your code should accept the number of linked lists as an input
• Then, for each linked list, it should accept integers as nodes till the user enters -1
It should next display all the linked lists in the lists entered by the user
Next, it should accept a number - this is an index position for the list (a linked list)
• For the linked list in the index position supplied by the user, your code should find the node with the smallest value and delete it
o Your code should display "Empty!", if there is no nodes left in the linked list after removing the smallest node!
Finally, it should print the modified linked list
For example, when the input is:
3
7
-1
3
4
7
9.
-1
1
The output should be:
linked list [ 0 ]:
1 3 5 7
linked list [ 1 ]:
3 4 7 2 9
the smallest node is:
2
List 1 after removing the smallest node:
3 4 79
Note Make sure to check for the following possible user's mistakes
• if the user inputs a negative number as the number of linked lists in the list, your code should display: "Wrong input! try again:"; then,
let the user to input another number
Same for the Empty linked lists](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffe5af5d5-f811-48fd-8e6e-3830a7fa1b94%2F061e07ba-cc1b-485e-a937-1b59096f121e%2Flys5ysg_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

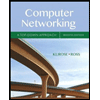
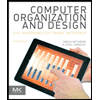
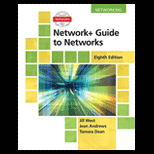
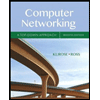
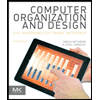
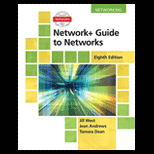
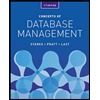
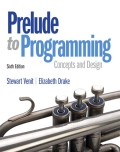
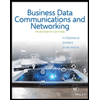