3.5.2: Recursive function: Writing the recursive case. Write code to complete factorial_str()'s recursive case. Sample output with input: 5 5! = 5 * 4 * 3 * 2 * 1 = 120 def factorial_str(fact_counter, fact_value): output_string = '' if fact_counter == 0: # Base case: 0! = 1 output_string += '1' elif fact_counter == 1: # Base case: print 1 and result output_string += str(fact_counter) + ' = ' + str(fact_value) else: # Recursive case output_string += str(fact_counter) + ' * ' next_counter = fact_counter - 1 next_value = next_counter * fact_value output_string += str(fact_counter) + ' = ' + str(fact_value) return output_string user_val = int(input()) print('{}! = '.format(user_val), end="") print(factorial_str(user_val, user_val))
3.5.2: Recursive function: Writing the recursive case. Write code to complete factorial_str()'s recursive case. Sample output with input: 5 5! = 5 * 4 * 3 * 2 * 1 = 120 def factorial_str(fact_counter, fact_value): output_string = '' if fact_counter == 0: # Base case: 0! = 1 output_string += '1' elif fact_counter == 1: # Base case: print 1 and result output_string += str(fact_counter) + ' = ' + str(fact_value) else: # Recursive case output_string += str(fact_counter) + ' * ' next_counter = fact_counter - 1 next_value = next_counter * fact_value output_string += str(fact_counter) + ' = ' + str(fact_value) return output_string user_val = int(input()) print('{}! = '.format(user_val), end="") print(factorial_str(user_val, user_val))
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
3.5.2: Recursive function: Writing the recursive case.
Write code to complete factorial_str()'s recursive case.
Sample output with input: 5
Sample output with input: 5
5! = 5 * 4 * 3 * 2 * 1 = 120
def factorial_str(fact_counter, fact_value):
output_string = ''
if fact_counter == 0: # Base case: 0! = 1
output_string += '1'
elif fact_counter == 1: # Base case: print 1 and result
output_string += str(fact_counter) + ' = ' + str(fact_value)
else: # Recursive case
output_string += str(fact_counter) + ' * '
next_counter = fact_counter - 1
next_value = next_counter * fact_value
output_string += str(fact_counter) + ' = ' + str(fact_value)
return output_string
user_val = int(input())
print('{}! = '.format(user_val), end="")
print(factorial_str(user_val, user_val))

Transcribed Image Text:**Recursive Function: Writing the Recursive Case**
### Challenge Activity: Recursive function
In this challenge, you will write code to complete the `factorial_str()` recursive case. The sample output provided for an input of 5 should be:
```
5! = 5 * 4 * 3 * 2 * 1 = 120
```
#### Code to Complete the Recursive Case
```python
def factorial_str(fact_counter, fact_value):
output_string = ''
if fact_counter == 0: # Base case: 0! = 1
output_string += '1'
elif fact_counter == 1: # Base case: print 1 and result
output_string += str(fact_counter) + ' = ' + str(fact_value)
else: # Recursive case
output_string += str(fact_counter) + ' * '
next_counter = fact_counter - 1
next_value = next_counter * fact_value
output_string += factorial_str(next_counter, next_value) # Recursive call
return output_string
user_val = int(input())
print('{}! = '.format(user_val), end="")
print(factorial_str(user_val, user_val))
```
In this code:
1. **Function Definition**:
- The function `factorial_str()` takes two arguments: `fact_counter` and `fact_value`.
- `fact_counter` keeps track of the current number in the factorial sequence.
- `fact_value` keeps track of the product of the numbers in the sequence.
2. **Base Case Handling**:
- If `fact_counter` is 0 (i.e., 0!), it sets `output_string` to `'1'`.
- If `fact_counter` is 1, it constructs the final part of the string with the result.
3. **Recursive Case Handling**:
- If `fact_counter` is greater than 1, it appends the current number followed by a multiplication symbol (`*`) to the `output_string`.
- It then decreases the `fact_counter` by 1 and calculates the new `next_value`.
- The function recursively calls itself with the updated counter and value, appending the result to `output_string`.
4. **User Input and Output**:
- It takes a user input for the factorial value, prints the factorial sequence

Transcribed Image Text:### Educative Example on Recursion in Python
**Topic: CS 219T: Python Programming - Creating Recursion**
In this section, we are focusing on creating recursion in Python. Below is an example of a student's attempt to write a recursive function to calculate the factorial of a number.
#### Example of Student Submission and Evaluation
1. **Testing with Input: 5**
- **Student's Output:**
```
5! = 5 * 5 = 5
```
- **Expected Output:**
```
5! = 5 * 4 * 3 * 2 * 1 = 120
```
The student's output is incorrect because they did not apply the factorial recursively but rather multiplied the number by itself.
2. **Testing with Input: 8**
- **Student's Output:**
```
8! = 8 * 8 = 8
```
- **Expected Output:**
```
8! = 8 * 7 * 6 * 5 * 4 * 3 * 2 * 1 = 40320
```
Again, the student's output shows an incorrect application since the factorial sequence was not followed.
**Remarks:**
The student's solution fails to compute the correct factorials due to a misunderstanding of the factorial function's recursive nature. Instead of recursively reducing the multiplication to simpler base cases, the calculations were prematurely concluded.
In factorial calculations, the function should correctly demonstrate the factorial chain, reducing the problem step-by-step until reaching the base case `1! = 1`.
#### Explanation on Factorial Calculation:
Factorial of a number `n` (denoted as `n!`) is the product of all positive integers from 1 to `n`. Hence, for any integer `n`, the recursive definition can be stated as:
- `n! = n * (n-1)!`
- With the base condition that `1! = 1`.
**Example: Calculating 5!**
- 5! = 5 * 4!
- 4! = 4 * 3!
- 3! = 3 * 2!
- 2! = 2 * 1!
- 1! = 1
Thus,
- 5! = 5 * 4 * 3 * 2 * 1 = 120
These tests show the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
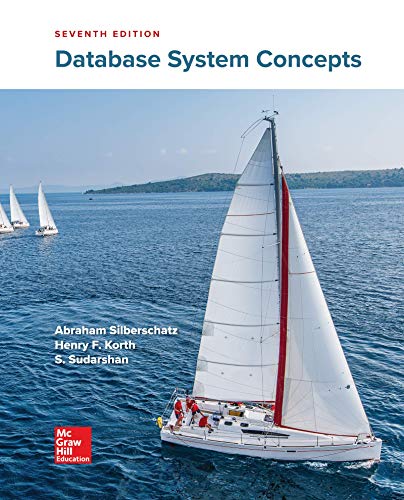
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
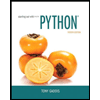
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
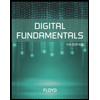
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
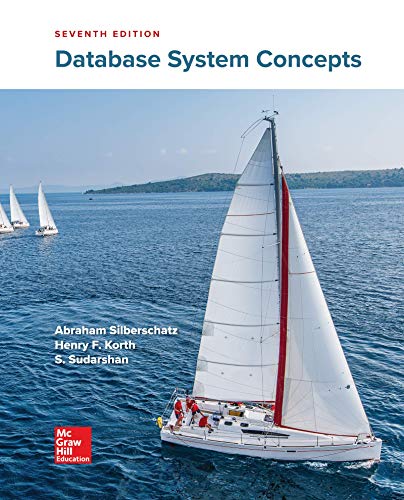
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
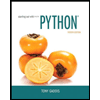
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
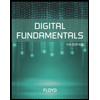
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
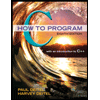
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
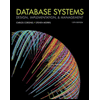
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
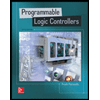
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education