Write a Java function to determine if the elements of an array can be split into two groups such that the sum of one group is a non-zero multiple of 10 and the sum of the other group is odd. Your solution must use recursive backtracking. * Example: * Given: {1, 2, 6, 3, 1} * Result: true because {1,2,6,1}, {3} is one possibility. * * Example: * Given: {4, 3, 5, 2} * Result: false * */ public static boolean splitOdd10(int[] num) { }
/**
* Write a Java function to determine if the elements of an array can be split into two groups such that the sum of one group is a non-zero multiple of 10 and the sum of the other group is odd.
Your solution must use recursive backtracking.
<code>
* Example:
* Given: {1, 2, 6, 3, 1}
* Result: true because {1,2,6,1}, {3} is one possibility.
*
* Example:
* Given: {4, 3, 5, 2}
* Result: false
* </code>
*/
public static boolean splitOdd10(int[] num) {
}

In this question we need to write a Java code to check whether we can split an array in two parts where sum of one array is multiple of 10 and sum of second array is odd or not. We need to use recursive backtracking to solve the given problem.
Step by step
Solved in 3 steps with 1 images

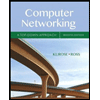
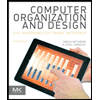
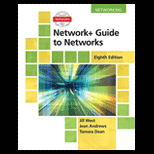
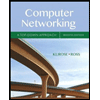
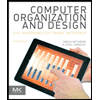
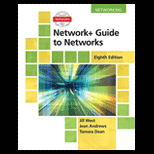
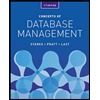
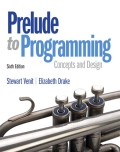
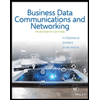