3.5.1: Recursive function: Writing the base case. Add an if branch to complete double_pennies()'s base case. Sample output with inputs: 1 10 Number of pennies after 10 days: 1024 # Returns number of pennies if pennies are doubled num_days times def double_pennies(num_pennies, num_days): total_pennies = 0 if "solution goes here" else: total_pennies = double_pennies((num_pennies * 2), (num_days - 1)) return total_pennies # Program computes pennies if you have 1 penny today, # 2 pennies after one day, 4 after two days, and so on starting_pennies = int(input()) user_days = int(input()) print('Number of pennies after', user_days, 'days: ', end="") print(double_pennies(starting_pennies, user_days))
Sample output with inputs: 1 10
# Returns number of pennies if pennies are doubled num_days times
def double_pennies(num_pennies, num_days):
total_pennies = 0
if "solution goes here"
else:
total_pennies = double_pennies((num_pennies * 2), (num_days - 1))
return total_pennies
#
# 2 pennies after one day, 4 after two days, and so on
starting_pennies = int(input())
user_days = int(input())
print('Number of pennies after', user_days, 'days: ', end="")
print(double_pennies(starting_pennies, user_days))



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

After working this code, this is the error code that popped up resulted in half right. Traceback (most recent call last): File "main.py", line 292, in <module> print(double_pennies(starting_pennies, user_days)) File "main.py", line 264, in double_pennies total_pennies = double_pennies((num_pennies * 2), (num_days - 1)) File "main.py", line 264, in double_pennies total_pennies = double_pennies((num_pennies * 2), (num_days - 1)) File "main.py", line 264, in double_pennies total_pennies = double_pennies((num_pennies * 2), (num_days - 1)) [Previous line repeated 995 more times] File "main.py", line 260, in double_pennies if num_days == 1: RecursionError: maximum recursion depth exceeded in comparison
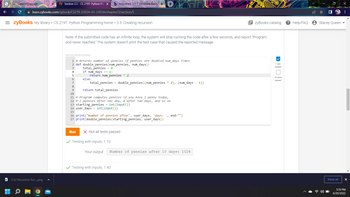
![**Recursive Function Example with Pennies Doubling**
**Testing with inputs: 1 10**
- **Your output:** Number of pennies after 10 days: 1024
**Testing with inputs: 1 40**
- **Your output:** Number of pennies after 40 days: 1099511627776
**Testing with inputs: 1 1**
- **Your output:** Number of pennies after 1 day: 2
**Test aborted**
- **Error Details:**
```
File "main.py", line 292, in <module>
print(double_pennies(starting_pennies, user_days))
File "main.py", line 264, in double_pennies
total_pennies = double_pennies((num_pennies * 2), (num_days - 1))
File "main.py", line 264, in double_pennies
total_pennies = double_pennies((num_pennies * 2), (num_days - 1))
File "main.py", line 264, in double_pennies
total_pennies = double_pennies((num_pennies * 2), (num_days - 1))
...
[Previous line repeated 995 more times]
File "main.py", line 260, in double_pennies
```
The error log shows that the program encountered a "recursion depth exceeded" error. This suggests that the recursive function `double_pennies` called itself too many times without reaching a base case, causing the program to exceed the maximum recursion depth allowed in Python.](https://content.bartleby.com/qna-images/question/788fc729-a951-45c4-9909-47eea7bbf82f/77d61ee1-3017-4e7a-a326-8724ffa1e988/fzr5okm_thumbnail.png)
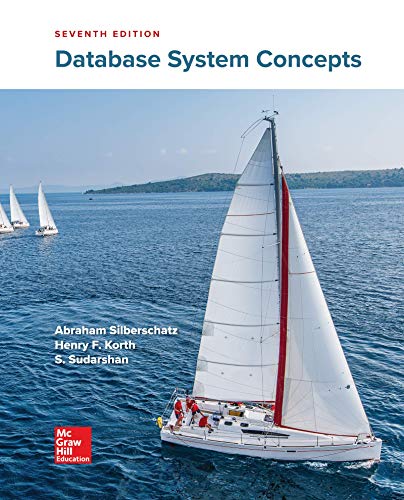
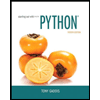
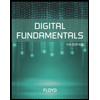
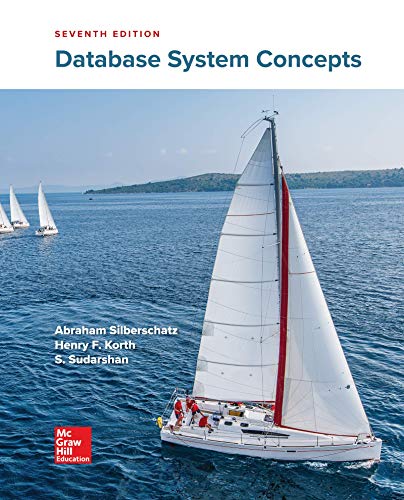
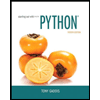
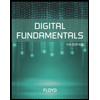
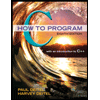
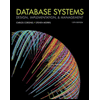
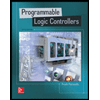