CHALLENGE ACTIVITY 6.5.1: Function call in expression. Assign max_sum with the greater of num_a and num_b, PLUS the greater of num_y and num_z. Use just one statement. Hint: Call find_max() twice in an expression. Sample output with inputs: 5.0 10.0 3.0 7.0 max_sum is: 17.0
CHALLENGE ACTIVITY 6.5.1: Function call in expression. Assign max_sum with the greater of num_a and num_b, PLUS the greater of num_y and num_z. Use just one statement. Hint: Call find_max() twice in an expression. Sample output with inputs: 5.0 10.0 3.0 7.0 max_sum is: 17.0
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
CHALLENGE ACTIVITY
6.5.1: Function call in expression.
Assign max_sum with the greater of num_a and num_b, PLUS the greater of num_y and num_z. Use just one statement. Hint: Call find_max() twice in an expression.
Sample output with inputs: 5.0 10.0 3.0 7.0
Sample output with inputs: 5.0 10.0 3.0 7.0
max_sum is: 17.0

Transcribed Image Text:344614.2153518.gx3zqy7
1 def find_max (num_1, num_2):
max_val = 0.0
1 test
passed
# if numl is greater than num2,
if (num_1 > num_2):
max_val = num_1
else:
5
# then numl is the maxVal.
All tests
6
# Otherwise,
passed
max_val = num_2
return max_val
7
# num2 is the maxVal
9
10 max_sum = 0.0
11
12 num_a = float (input ())
13 num_b = float(input ())
14 num_y
15 num_z
float(input())
float (input ())
16
17
'' Your solution goes here '
Run
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
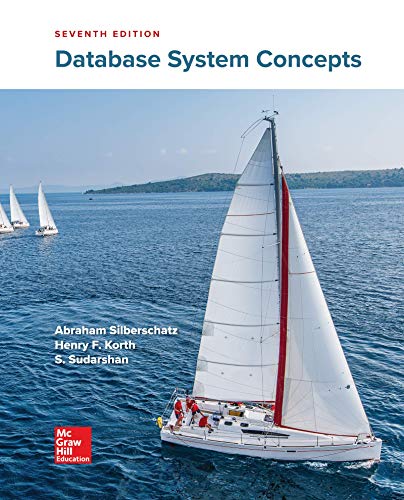
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
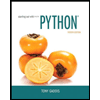
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
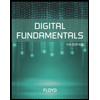
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
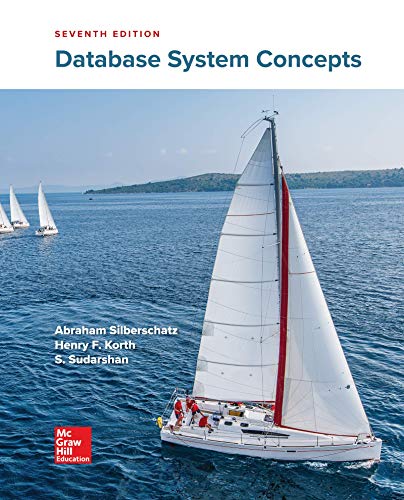
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
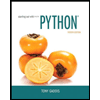
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
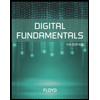
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
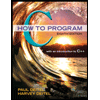
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
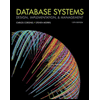
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
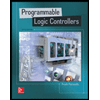
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education