1 3#include #include #include 4 #include #include using namespace std; 7 const int MAXRESULTS ▪ 20; // Max matches that can be found 9. const int MAXDICTWORDS = 30000; // Max words that can be read in 10 Bint loadDictionary(istream& dictfile, vector& dict) 11 12 { 13 return -1; // This compiles, but this is incorrect 14 15 Bint permute(string word, vector& dict, vector& results) 16 17 { 18 return -1; // This compiles, but this is incorrect 19 20 Evoid display(vector& results) 21 22 23 return; // This compiles, but this is incorrect 24 25 26 Bint main() { vector results(MAXRESULTS); vector dict(MAXDICTWORDS); ifstream dictfile; int nwords; 27 28 29 // file containing the list of words // number of words read from dictionary 30 31 32 string word; 33 dictfile.open("words.txt"); if (!dictfile) { 34 35 cout <« "File not found!" « endl; return (1); 36 37 38 39 40 nwords = loadDictionary(dictfile, dict); 41 42 dictfile.close(); 43 44 cout <« "Please enter a string for an anagram: 45 cin >> word; 46 int numMatches = permute (word, dict, results); if (!numMatches) 47 48 49 cout « "No matches found" <« endl; 50 else 51 display(results); 52 53 return e; 54
1 3#include #include #include 4 #include #include using namespace std; 7 const int MAXRESULTS ▪ 20; // Max matches that can be found 9. const int MAXDICTWORDS = 30000; // Max words that can be read in 10 Bint loadDictionary(istream& dictfile, vector& dict) 11 12 { 13 return -1; // This compiles, but this is incorrect 14 15 Bint permute(string word, vector& dict, vector& results) 16 17 { 18 return -1; // This compiles, but this is incorrect 19 20 Evoid display(vector& results) 21 22 23 return; // This compiles, but this is incorrect 24 25 26 Bint main() { vector results(MAXRESULTS); vector dict(MAXDICTWORDS); ifstream dictfile; int nwords; 27 28 29 // file containing the list of words // number of words read from dictionary 30 31 32 string word; 33 dictfile.open("words.txt"); if (!dictfile) { 34 35 cout <« "File not found!" « endl; return (1); 36 37 38 39 40 nwords = loadDictionary(dictfile, dict); 41 42 dictfile.close(); 43 44 cout <« "Please enter a string for an anagram: 45 cin >> word; 46 int numMatches = permute (word, dict, results); if (!numMatches) 47 48 49 cout « "No matches found" <« endl; 50 else 51 display(results); 52 53 return e; 54
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please complete the following functions:
1. int loadDictionary(istream& dictfile,
2. int permute(string word, vector<string>& dict, vector<string>& results)
3. void display(vector<string>& results)

Transcribed Image Text:```cpp
#include <iostream>
#include <fstream>
#include <istream>
#include <string>
#include <vector>
using namespace std;
const int MAXRESULTS = 20; // Max matches that can be found
const int MAXDICTWORDS = 30000; // Max words that can be read in
int loadDictionary(istream& dictfile, vector<string>& dict)
{
return -1; // This compiles, but this is incorrect
}
int permute(string word, vector<string>& dict, vector<string>& results)
{
return -1; // This compiles, but this is incorrect
}
void display(vector<string>& results)
{
return; // This compiles, but this is incorrect
}
int main()
{
vector<string> results(MAXRESULTS);
vector<string> dict(MAXDICTWORDS);
ifstream dictfile; // file containing the list of words
int nwords; // number of words read from dictionary
string word;
dictfile.open("words.txt");
if (!dictfile)
{
cout << "File not found!" << endl;
return 1;
}
nwords = loadDictionary(dictfile, dict);
dictfile.close();
cout << "Please enter a string for an anagram: ";
cin >> word;
int numMatches = permute(word, dict, results);
if (!numMatches)
cout << "No matches found" << endl;
else
display(results);
return 0;
}
```
### Explanation
The provided C++ code is a framework for a program that attempts to find anagrams of a given word using a dictionary of words read from a file. Here is a breakdown of the code:
- **Constants Definition**
- `MAXRESULTS`: Specifies the maximum number of anagram matches the program can find.
- `MAXDICTWORDS`: Specifies the maximum number of words that can be loaded from the dictionary file.
- **Functions**
- `loadDictionary`: Reads words from a file stream into a vector. Currently, it returns `-1` indicating the implementation is incomplete.
- `permute`: Takes a word and attempts to find its anagrams within a given dictionary. The return value and functionality are placeholders.
- `display`: Takes a vector of results and displays them. The current return statement is a placeholder.
- **Main Function**
- Sets

Transcribed Image Text:**Sample Runs**
Here are two examples of how the program might work:
```
Please enter a string for an anagram: opt
Matching word opt
Matching word pot
Matching word top
Please enter a string for an anagram: blah
No matches found
```
**Requirements**
You must write these three functions with the exact same function signature (include case):
1. ```cpp
int loadDictionary(istream &dictfile, vector<string>& dict);
```
Places each string in `dictfile` into the vector `dict`. Returns the number of words loaded into `dict`.
2. ```cpp
int permute(string word, vector<string>& dict, vector<string>& results);
```
Places all the permutations of `word`, which are found in `dict` into `results`. Returns the number of matched words found.
3. ```cpp
void display(vector<string>& results);
```
Displays `size` number of strings from `results`. The `results` can be printed in any order.
For words with double letters, you may find that different permutations match the same word in the dictionary. For example, if you find all the permutations of the string "kloo" using the algorithm we’ve discussed, you may find that the word "look" is found twice. The o's in "kloo" take turns in front. Your program should ensure that matches are unique; in other words, the results array returned from the `permute` function should have no duplicates.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
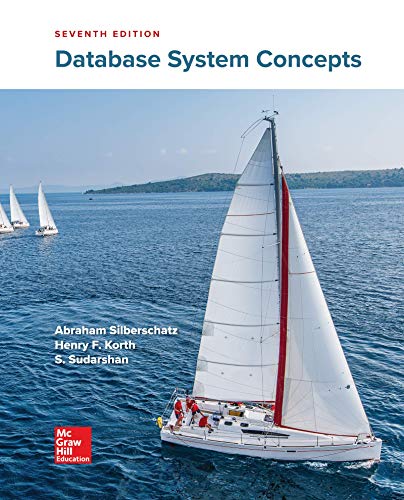
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
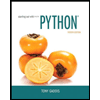
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
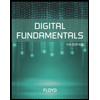
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
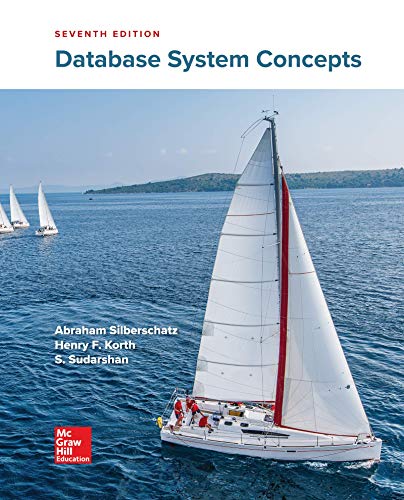
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
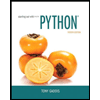
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
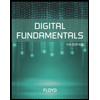
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
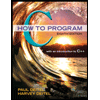
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
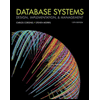
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
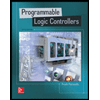
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education