. Pet class package Library; public class Pet { String name; String type; int weight; public Pet() { System.out.println("Creating a new pet"); } @Override public void finalize() { System.out.println("In the pet destructor."); } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getType() { return type; } public void setType(String type) { this.type = type; } public int getWeight() { return weight; } public void setWeight(int weight) { this.weight = weight; } } 2.Main class package Library; import java.util.Random; import java.util.Scanner; public class MyPets { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("How many pets do you have?"); int numOfPets = Integer.valueOf(sc.nextLine()); Random r=new Random(); Pet[] pets = new Pet[numOfPets]; int index = 0; for(int i=1;i<= numOfPets;i++) { System.out.println("What is the name of your pets?"); String name = sc.nextLine(); System.out.println("What type of pet is "+name); String type = sc.nextLine(); int weight =r.nextInt(100); Pet p = new Pet(); p.setName(name); p.setType(type); p.setWeight(weight); pets[index] = p; index++; } System.out.println("These are the pets you have :"); for(Pet p : pets) { System.out.println("Pet Name : "+p.getName()); System.out.println("Pet Type : "+p.getType()); System.out.println("Pet Weight : "+p.getWeight()); System.out.println(); } for(Pet p : pets) { p.finalize(); p =null; } pets=null; System.out.println("Press any key to continue..."); } }
****will this write out in C++ the same that it will in java
1. Pet class
package Library;
public class Pet {
String name;
String type;
int weight;
public Pet() {
System.out.println("Creating a new pet");
}
@Override
public void finalize()
{
System.out.println("In the pet destructor.");
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
}
2.Main class
package Library;
import java.util.Random;
import java.util.Scanner;
public class MyPets {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("How many pets do you have?");
int numOfPets = Integer.valueOf(sc.nextLine());
Random r=new Random();
Pet[] pets = new Pet[numOfPets];
int index = 0;
for(int i=1;i<= numOfPets;i++) {
System.out.println("What is the name of your pets?");
String name = sc.nextLine();
System.out.println("What type of pet is "+name);
String type = sc.nextLine();
int weight =r.nextInt(100);
Pet p = new Pet();
p.setName(name);
p.setType(type);
p.setWeight(weight);
pets[index] = p;
index++;
}
System.out.println("These are the pets you have :");
for(Pet p : pets) {
System.out.println("Pet Name : "+p.getName());
System.out.println("Pet Type : "+p.getType());
System.out.println("Pet Weight : "+p.getWeight());
System.out.println();
}
for(Pet p : pets) {
p.finalize();
p =null;
}
pets=null;
System.out.println("Press any key to continue...");
}
}

Step by step
Solved in 3 steps with 1 images

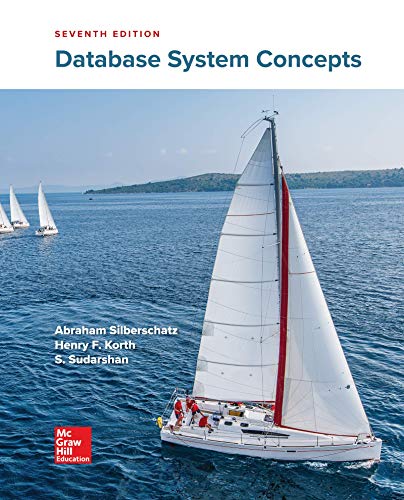
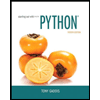
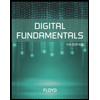
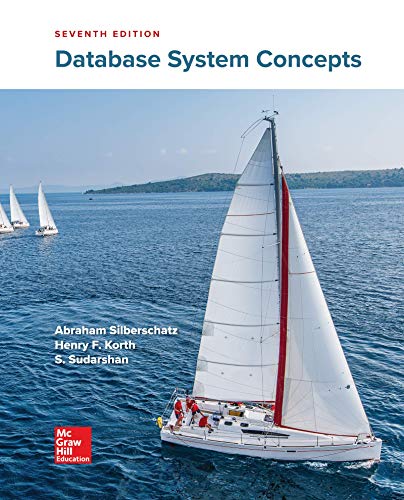
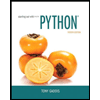
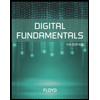
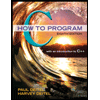
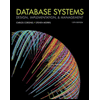
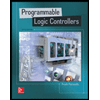