PreparationForExamination_Term1
docx
keyboard_arrow_up
School
University of Miami *
*We aren’t endorsed by this school
Course
422
Subject
Mechanical Engineering
Date
Oct 30, 2023
Type
docx
Pages
10
Uploaded by CorporalRaven3623
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
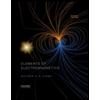
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
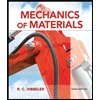
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
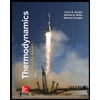
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
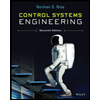
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
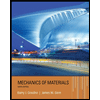
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
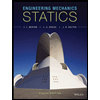
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
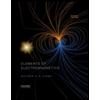
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
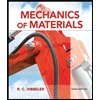
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
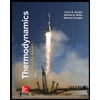
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
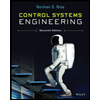
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
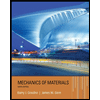
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
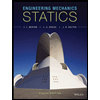
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Browse Popular Homework Q&A
Q: Use least common multiple or greatest common divisor to solve the problem.
Three taxi cabs make a…
Q: Find the least squares regression line for the data points. (Let x be the independent variable and y…
Q: Determine the parent function from which the graph of the function shown below can be obtained.…
Q: measure the depth to the water as 88.98 feet.
1) What is the depth of the water surface below land…
Q: 0.3456 g of a solid acid is dissolved in 50 mL of water in a flask and a few drops of…
Q: A deposit of $10000 is made into an account paying a nominal annual rate of 4.25%. If the
interest…
Q: Challenge #2: Sketch the derivative of the function, f(x).
T
X
Q: By January 2014 the US population had grown to 317.3 million and the US Federal Debt was a reported…
Q: +2 -3
a. solid calcium nitride plus heat
Ca Na 3 Ca +2N
→
b. solid lead (IV) carbonate plus heat…
Q: An investment of $9000 grows to $10,296.59 in 4 years. Find the annual rate of return for annual…
Q: Dilly Farm Supply is located in a small town in the rural west. Data regarding the store's…
Q: Question
The graph of f(x) is given below. Which of the three conditions for continuity are not true…
Q: The random variable x is the number of
x | P(X=x)
0
1
2
3
4
5
6
7
3.5
Find the mean of the…
Q: In Question 5 (and potentially in Question 6), your analysis considered a price ceiling at a price…
Q: A thin film of oil, on the surface of glass , results in destructive interference. If the wavelength…
Q: B
68
m/BCA=
C
Don't forget to put the degree symbol in each answer.
m/ACD=
D
m/BAC =
m/D=
m/CAD=
Q: Classify the following reaction: H₂SO₄(aq) + Fe(s) → H₂(g) + FeSO₄(aq)
A) synthesis (or combination)…
Q: 45; and interpret the results.
46. Supply. A supply function for a certain product is given by
S(p)…
Q: (a) Prepare an installment payments schedule for the first 4 years.
(b) Prepare the entries for (1)…
Q: A man standing 1.50 m in front of a shaving mirror produces an inverted image 20.2 cm in front of…
Q: Milan drove 240 miles using 9 gallons of gas. At this rate, how many gallons of gas would he need to…
Q: calculate the relative rate of a reaction (in seconds) that takes 1 minute and 49 seconds.
Q: 0.40 M solution of hydrocyanic acid, HCN, has a Ka of 4.93 x 10-10. What is the pH of this…
Q: O Using the Customer database (Customer Database has one table Customer), Create Class Library…
Q: 8. What carbonyl compound and what phosphorous ylide might you use to prepare the following…