Assignment1 - Jupyter Notebook (1)
pdf
keyboard_arrow_up
School
Cambridge College *
*We aren’t endorsed by this school
Course
NLP
Subject
Mechanical Engineering
Date
Feb 20, 2024
Type
Pages
6
Uploaded by LieutenantComputerGuanaco32
10/22/23, 11:30 PM
Assignment1 - Jupyter Notebook
localhost:8888/notebooks/Assignment1.ipynb#
1/6
In [6]:
In [10]:
1. Calculate the mean centering vector (a 5 x 1 vector)
In [11]:
2. Calculate the scaling vector (a 5 x 1 vector)
In [12]:
3. What steps you would take to apply the centering and scaling vectors to the X matrix?
a. Centering: Subtract the mean centering vector from each row of the X matrix.
b. Scaling: Divide each column of the centered matrix by its corresponding value in the scaling vector (standard deviation).
Out[10]:
Unnamed: 0
Oil
Density
Crispy
Fracture
Hardness
0
B110
16.5
2955
10
23
97
1
B136
17.7
2660
14
9
139
2
B171
16.2
2870
12
17
143
3
B192
16.7
2920
10
31
95
4
B225
16.3
2975
11
26
143
Out[11]:
Oil 17.202
Density 2857.600
Crispy 11.520
Fracture 20.860
Hardness 128.180
dtype: float64
Out[12]:
Oil 1.592007
Density 124.499980
Crispy 1.775571
Fracture 5.466073
Hardness 31.127578
dtype: float64
import
numpy as
np
import
pandas as
pd
import
matplotlib.pyplot as
plt
data =
pd.read_csv(
'./Downloads/food-texture.csv'
)
data.head()
mean_centering_vector =
data[[
'Oil'
, 'Density'
, 'Crispy'
, 'Fracture'
, 'Hardness'
]].mean()
mean_centering_vector
scaling_vector =
data[[
'Oil'
, 'Density'
, 'Crispy'
, 'Fracture'
, 'Hardness'
]].std()
scaling_vector
10/22/23, 11:30 PM
Assignment1 - Jupyter Notebook
localhost:8888/notebooks/Assignment1.ipynb#
2/6
4. Draw a scatter plot of Crispy vs. Fracture using all 50 observations from the raw data table.
In [16]:
plt.figure(figsize
=
(
10
, 6
))
plt.scatter(data[
'Crispy'
], data[
'Fracture'
], color
=
'blue'
)
plt.title(
'Scatter Plot of Crispy vs. Fracture (Raw Data)'
)
plt.xlabel(
'Crispy'
)
plt.ylabel(
'Fracture'
)
plt.grid(
True
)
plt.show()
10/22/23, 11:30 PM
Assignment1 - Jupyter Notebook
localhost:8888/notebooks/Assignment1.ipynb#
3/6
5. Draw a scatter plot of Crispy vs. Fracture after you have centered and scaled the data. What observations can you make comparing the two scatter plots?
In [17]:
Observations comparing the two scatter plots:
1. The shape and distribution of the data points remain consistent between the two plots. Centering and scaling have not changed the inherent relationships between the variables.
2. In the raw data plot, the data points are spread out over the original scales of the variables. In the centered and scaled plot, the data points are concentrated around the origin, as both axes now have a mean of zero and a
standard deviation of one.
3. The centered and scaled plot makes it easier to identify potential patterns or clusters in the data, as the scale is consistent across both axes.
centered_data =
data[[
'Oil'
, 'Density'
, 'Crispy'
, 'Fracture'
, 'Hardness'
]] -
mean_centering_vector
scaled_data =
centered_data /
scaling_vector
plt.figure(figsize
=
(
10
, 6
))
plt.scatter(scaled_data[
'Crispy'
], scaled_data[
'Fracture'
], color
=
'red'
)
plt.title(
'Scatter Plot of Crispy vs. Fracture (Centered and Scaled Data)'
)
plt.xlabel(
'Crispy (Centered and Scaled)'
)
plt.ylabel(
'Fracture (Centered and Scaled)'
)
plt.grid(
True
)
plt.show()
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
10/22/23, 11:30 PM
Assignment1 - Jupyter Notebook
localhost:8888/notebooks/Assignment1.ipynb#
4/6
6. Use Aspen ProMV (or a software tool of your choice) to construct a PCA model on this data. What is the R^2 for the first and second components? What is the total
R^2 using 2 components?
In [19]:
1. R^2 for the first component: 60.62%.
2. R^2 for the second component: 25.91%.
3. Total R^2 using the first two components: 86.54%.
This indicates that the first two components together explain approximately 86.54% of the variance in the centered and scaled data.
7. Report the R^2 value for each of the 5 variables after adding (a) one component and (b) two components.
In [20]:
The R^2 values (squared loadings) for each of the 5 variables are:
(a) After adding one component:
1. Oil: 20.93%
2. Density: 22.92%
3. Crispy: 28.34%
4. Fracture: 25.45%
5. Hardness: 2.35%
(b) After adding two components:
1. Oil: 34.66%
2. Density: 35.65%
3. Crispy: 32.25%
4. Fracture: 30.34%
Out[19]:
(0.6062426334584964, 0.2591411527599619, 0.8653837862184584)
Out[20]:
(array([0.20933684, 0.22919725, 0.28343663, 0.25449692, 0.02353236]),
array([0.34656178, 0.35646779, 0.32250651, 0.30344402, 0.6710199 ]))
from
sklearn.decomposition import
PCA
pca =
PCA(n_components
=
2
)
pca.fit(scaled_data)
r2_first_component =
pca.explained_variance_ratio_[
0
]
r2_second_component =
pca.explained_variance_ratio_[
1
]
total_r2_two_components =
sum
(pca.explained_variance_ratio_)
r2_first_component, r2_second_component, total_r2_two_components
loadings =
pca.components_.T
squared_loadings_one_component =
loadings[:, 0
] **
2
squared_loadings_two_components =
squared_loadings_one_component +
(loadings[:, 1
] **
2
)
squared_loadings_one_component, squared_loadings_two_components
10/22/23, 11:30 PM
Assignment1 - Jupyter Notebook
localhost:8888/notebooks/Assignment1.ipynb#
5/6
5. Hardness: 67.10%
8. Write down the values of the p1 loading vector. Also, create a bar plot of these values.
In [21]:
The values of the p1 loading vector for each variable are:
Out[21]:
array([-0.45753343, 0.4787455 , -0.53238767, 0.50447688, -0.15340262])
p1_loading_vector =
loadings[:, 0
]
variables =
[
'Oil'
, 'Density'
, 'Crispy'
, 'Fracture'
, 'Hardness'
]
plt.figure(figsize
=
(
10
, 6
))
plt.bar(variables, p1_loading_vector, color
=
'green'
)
plt.title(
'p1 Loading Vector'
)
plt.xlabel(
'Variables'
)
plt.ylabel(
'Loading Value'
)
plt.grid(axis
=
'y'
)
plt.show()
p1_loading_vector
10/22/23, 11:30 PM
Assignment1 - Jupyter Notebook
localhost:8888/notebooks/Assignment1.ipynb#
6/6
Oil: −0.4575 Density: 0.4787 Crispy: −0.5324 Fracture: 0.5045 Hardness: −0.1534
9. What are the characteristics of pastries with a large negative t1 value?
The t1 value is the score of the first principal component. A large negative t1 score would mean that the pastry has characteristics opposite to the direction of the positive p1 loading vector.
A large negative t1 value would suggest the pastry has a high oil content (since the loading for oil is negative). It would also suggest a low density (since the loading for density is positive). The pastry would likely be very crispy
(since the loading for crispy is negative).It would have a lower fracture angle (since the loading for fracture is positive). The hardness characteristic is less influential in this component, but a large negative t1 value would suggest a
slightly softer pastry (since the loading for hardness is negative).
10. Replicate the calculation of t1 for pastry B554. Show each of the 5 terms that make up this linear combination.
In [23]:
The t1 score for pastry B554 is approximately 1.5424. The linear combination for t1 can be broken down into the following terms:
1. Oil Term: 0.7191
2. Density Term: 0.4707
3. Crispy Term: 0.4558
4. Fracture Term: −0.0794
5. Hardness Term: −0.0238
The t1 score is the sum of these terms. This breakdown gives insight into how each variable contributes to the t1 score for pastry B554 based on its relationship with the first principal component.
In [ ]:
Out[23]:
(1.5423623149809962,
array([ 0.71906014, 0.47067035, 0.45575714, -0.07937144, -0.02375388]))
pastry_b554 =
scaled_data[data[
'Unnamed: 0'
] ==
'B554'
].values[
0
]
t1_score_b554 =
pastry_b554.dot(p1_loading_vector)
terms_b554 =
pastry_b554 *
p1_loading_vector
t1_score_b554, terms_b554
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Related Questions
Draw the operating curves of the given system on the graph using all necessary curves in excel
ÖN = 5 so you must choose the correct H equation
arrow_forward
USE MATLAB
(Convolutional Code) The vector representation of a convolutional code is g1=[1 1]and g2=[1 0]. If the received sequence is 11 11 01 10, please use Viterbi Algorithm todecode it. Please show the full trellis diagram, including the updated trellis statemetrics.
arrow_forward
3. Use the second method of frame assignment, and find the Jacobian of the
following robot using the velocity propagation method (Ignore all theta related
offsets):
03
02 L2
01
Please answer the question precisely
and completely to use the second
method of frame assignment to find
the D-H table [alpha (i-1) , a (i-1) , d (i) ,
theta (i)], and find the jacobian matrix
using the velocity propagation method
arrow_forward
хоос»
0 Python 3 (p
Define a python function to determine the 2nd moment of area I, under the parabola, with respect to the centroidal-axis. The
parabolic curve is defined as z = ky2, where k = b/h². The centroidal axis has a given distance d with respect to z axis.
Hints: Use the parallel axis theorem. Find the 2nd moment of area against z axis first and then use the formula I₂ = I₁ + d²A to
calculate I, where d is a given value (no need to derive). Note that this question requires you to derive the equation first.
$
4
l) | Idle Mem: 260.77/12288.00 MB
Q
F4
R
y
1
1
0
do 5
[ ]: # Complete the function given the variables b,h,d and return the value as "Area","Iz" and "Ic"
# This is not about numerical integration, we only need the analytical solution.
# Don't change the predefined content, only fill your code in the region "YOUR CODE"
Mode: Command
%
9
F5
0
T
.
Markdown v
z = ky²
2
6
C
F6
Validate
Y
&
7
8:
F7
U
*
8
DII
F8
I
19
F9
b
O
)
Ln 1, Col 1 English (United States)…
arrow_forward
Let E, B, and S be respectively the Earth-, body-fixed, and sensor frames. These frames are
related through the following rotation vectors:
B
[ABE]" = (1/3,0, 0) rad
[ASB]° = (0,0, T) rad
Using the Euler symmetric parameters, compute the rotation vector [ASE]°.
arrow_forward
Figure 1 shows a six degree of freedom industrial robot.
i. Construct the coordinates frames based on the D-H representations.
ii. Complete the D-H parameter as illustrated in Table 1.
arrow_forward
Help with the MATLAB code to do the following:
Given an audio recording of vehicles, compute Power Spectral Density, 1/3 octave band level, octave band level, A-weighted Leq, C-weighted Leq, L10 (unweighted since sample is short), L90 (unweighted since sample is short). If the measurement is made at approximately 50 ft, and that the vehicles are moving at an average of 65 mph, what is the flow rate Q? If instead, the noise is dominated by 1-2 motorcycles, how fast are they going?
Given-
-The data are in counts
-Calibration factor is 133 uPa/count
-Sample rate is 44100Hz. -Remember that in air the dB reference is 20 uPa
-Pwelch requires that the inputs are doubles
arrow_forward
create matlab code and screen shot both files
arrow_forward
permanent-magnet (pm) genera x
Bb Blackboard Learn
L STAND-ALONE.mp4 - Google Dri x
O Google Drive: ülwgjuó jc lis u
O ME526-WindEnergy-L25-Shuja.p x
O File | C:/Users/Administrator/Desktop/KFUPM%20Term%232/ME526/ME526-WindEnergy-L25-Shuja.pdf
(D Page view
A Read aloud
T) Add text
V Draw
Y Highlight
O Erase
17
of 26
Wind Farms
Consider the arrangement of three wind turbines in the following schematic in which wind
turbine C is in the wakes of turbines A and B.
Given the following:
- Uo = 12 m/s
A
-XẠC = 500 m
-XBC = 200 m
- z = 60 m
- Zo = 0.3 m
U.
-r, = 20 m
B
- CT = 0.88
Compute the total velocity deficit, udef(C) and the velocity at wind turbine C, namely Vc.
Activate Windows
Go to Settings to activate Windows.
Wind Farms (Example Answer)
5:43 PM
A 4)) ENG
5/3/2022
I!
arrow_forward
Part III Capstone Problem
Interactive Physics - [Lab7Part3.IP]
Eile Edit World View Object Define Measure Script Window Help
Run StoplI Reset
圖|& 品凸?
Time
Length of Spring 22
6.00
dx
Center of Mass of Rectangle 2
5.000
Tension of Rope 3
Jain@
IFI
... N
ot
rot
***lad
Split
4.000
Velocity of Center of Mass of Rectangle 2
Vx Vx
V Vy
MM
Ve
- m/s
m/s
3.00
*** m/s
Vo
..* lad/s
2 00
Center of Mass of Rectangle 1
1.000
tol
rot
*.* rad
EVelocity of Center of Mass of Rectangle 1
Vx Vx
VVy
M
0.000
-m/s
w 30
m/s
w..
MI
Ve
母100
*** m/s
Vo
... rad/s
+
EAcceleration of Center of Mass of Rectangle 1
Ax Ax
A Ay
AUJAI
Ae
--- m/s^2
... m/s^2
-- m/s^2
.-- rad/s^2
3.00
Aø
Mass M1 = 2.25 kg is at the end of a rope that is 2.00 m in length. The initial angle with
respect to the vertical is 60.0° and M1 is initially at rest. Mass M1 is released and strikes M2
= 4.50 kg exactly horizontally. The collision is elastic. After collision, mass M2 is moving on
a frictionless surface, but runs into a rough patch 2.00…
arrow_forward
I want to run the SGP4 propagator for the ISS (ID = 25544) I got from spacetrack.org in MATLAB. I don't know where to get the inputs of the function. Where do I get the inFile and outFile that is mentioned in the following function.
% Purpose:
% This program shows how a Matlab program can call the Astrodynamic Standard libraries to propagate
% satellites to the requested time using SGP4 method.
%
% The program reads in user's input and output files. The program generates an
% ephemeris of position and velocity for each satellite read in. In addition, the program
% also generates other sets of orbital elements such as osculating Keplerian elements,
% mean Keplerian elements, latitude/longitude/height/pos, and nodal period/apogee/perigee/pos.
% Totally, the program prints results to five different output files.
%
%
% Usage: Sgp4Prop(inFile, outFile)
% inFile : File contains TLEs and 6P-Card (which controls start, stop times and step size)
% outFile : Base name for five output files
%…
arrow_forward
ZVA @
e SIM a
A moodle1.du.edu.om
Solve the second order
homogeneous differential
equation y " -6y'-7y = 0
with intial values
y(0) = 0; y'(0) = 1
%3D
Maximum file size: 200MB,
maximum number of files: 1
Files
II
arrow_forward
I am trying to plot an orbit in MATLAB. There is something wrong with my code because the final values I get are incorrect. The code is shown below. The correct values are in the image.
mu = 3.986*10^5; % Earth's gravitational parameter [km^3/s^2]
% Transforming orbital elements to cartesian coordinate system for LEOa_1 = 6782.99;e_1 = 0.000685539;inc_1 = 51.64;v_1 = 5;argp_1 = 30;raan_1 = 10;
[x_1, y_1, z_1, vx_1, vy_1, vz_1] = kep2cart(a_1, e_1, inc_1, raan_1, ... argp_1, v_1);
Y_1 = [x_1, y_1, z_1, vx_1, vy_1, vz_1];
% time_span for two revolutions (depends on the orbit)t1 = [0 (180*60)];
% Setting tolerancesoptions = odeset('RelTol',1e-12,'AbsTol',1e-12);
% Using ODE45 to numerically integrate for LEO[t_1, state_1] = ode45(@OrbitProp, t1, Y_1, options);
function dYdt = OrbitProp(t, Y)
mu = 3.986*10^5; % Earth's gravitational parameter [km^3/s^2]
% State Vector
x = Y(1); % [km]
y = Y(2); % [km]
z = Y(3); % [km]
vx = Y(4);…
arrow_forward
PLS DO FAST 2 THUMBS UP WILL BE GIVEN THANK U MAKE SURE NOT TO COPY FEOM ELSE WHERE THEY ARE WRONG THX
arrow_forward
How do you transform from ECEF to ECI? I have the r vector in ecef and eci and the modified julian date. How do you use the rotation matrices to get to r_eci.
r_ecef = [-1016.215157; 5431.875007; -3174.1];
r_eci = [-2010.4; -5147.4; -3174.1];
MJD = 57923.6666667; % Modified Julian Date
arrow_forward
Fourier Methods Question
arrow_forward
C
14:40
Two cables AB and AC are acting on the pole with forces
FAB 760N and FAC = 480N with parameters defining the
attachment points shown in the table. We want to write the
vector FAB in cartesian vector form.
X
-
X2
Y₂
FAB
FAC
X1
Y1
X2
Y2
=
Y₁
IN
parameters value units
FAB
760
N
FAC 480
L
8
3
wamap.org
33
A
3
4
Z
F₁
AB
î+
B
N
Ζεεειειε
m
m
m
Write the vector FAB in Cartensian Vector Notation.
Round your final answers to 3 significant figures.
m
L
X1
3+
arrow_forward
How would you solve for the equation of motion using Lagrange equations ?
arrow_forward
Find the local maximum and minimum values and saddle point(s) of the function. You are encouraged to use a calculator or computer to graph the function with a domain and viewpoint that reveals all the important aspects of the function. (Enter your answers as comma-separated lists. If an answer does not exist, enter DNE.)
f(x, y) = 9 sin(x) sin(y), −? < x < ?, −? < y < ?
local maximum value(s)
local minimum value(s)
saddle point(s)
(x, y)
=
arrow_forward
I was given a practice question for transforming equation. In the image I will have a slide the lecture note of an example of it being done but I do not understand what was done exactly. Please explain how to answer the question. Thank you
arrow_forward
Fantastick.
of 6)
A Microsoft PowerPoint - chapter 2 x
O Rotational system.pdf
O Essay on Life in a Big City for Stu x
10 Advantages of Living
A https://moodle1.du.edu.om/mod/quiz/attempt.php?attempt340840&cmid%3D2247468&page=D4
- Moodle English (en) -
01(1)
18 N-m-s/rad
1 N-m-s/rad T(1) 02(1)
$ kg-m2
3 kg-m2
3 N-m/rad
9 N-m/rad
(a)
Figure P2.16a
John Wiley & Sons, Inc. All rights reserved.
The transfer function where the output Theta, is
0,(s)
H(s) =
T(s)
s² +5s +1
s* +30s` +80s² +s +15
a.
%3D
S
0,(s)
b. H(s)=-
s´+5s +1
%3D
25s +30s +80s² +s+15
4.
T(s)
0,(s)
H(s)= -
T(s)
5s² +9s +9
15s* +30s+95s² + s+ 27
c.
4.
5s +9s +9
0,(s)
d. H(s)=
T(s)
15s +32s +95s² +99s+ 27
arrow_forward
Don't Use Chat GPT Will Upvote And Give Handwritten Solution Please
arrow_forward
A mechanic changing a tire rolls a wheel along the ground towards the car. The radius of the
wheel is 42cm, and the speed of the wheel as it rolls is 2 revolutions per second.
Height Above Ground
(m)
radiu
HIDE
wheel spet
Time
The diagram above illustrates the vertical motion of a point on the tire over time. It is possible to model
the height of this point using a sinusoidal function of the form h(t)=-a sin[b(t-c)]+d.
a) Determine the length of time required for one revolution of the tire.
b) State the numerical value for each of the parameters a, b, c & d.
And write a function representing the motion of the point in the form h(t)= -a sin[b(t−c)]+d.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
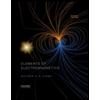
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
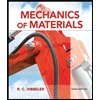
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
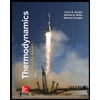
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
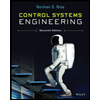
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
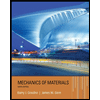
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
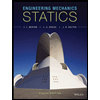
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- Draw the operating curves of the given system on the graph using all necessary curves in excel ÖN = 5 so you must choose the correct H equationarrow_forwardUSE MATLAB (Convolutional Code) The vector representation of a convolutional code is g1=[1 1]and g2=[1 0]. If the received sequence is 11 11 01 10, please use Viterbi Algorithm todecode it. Please show the full trellis diagram, including the updated trellis statemetrics.arrow_forward3. Use the second method of frame assignment, and find the Jacobian of the following robot using the velocity propagation method (Ignore all theta related offsets): 03 02 L2 01 Please answer the question precisely and completely to use the second method of frame assignment to find the D-H table [alpha (i-1) , a (i-1) , d (i) , theta (i)], and find the jacobian matrix using the velocity propagation methodarrow_forward
- хоос» 0 Python 3 (p Define a python function to determine the 2nd moment of area I, under the parabola, with respect to the centroidal-axis. The parabolic curve is defined as z = ky2, where k = b/h². The centroidal axis has a given distance d with respect to z axis. Hints: Use the parallel axis theorem. Find the 2nd moment of area against z axis first and then use the formula I₂ = I₁ + d²A to calculate I, where d is a given value (no need to derive). Note that this question requires you to derive the equation first. $ 4 l) | Idle Mem: 260.77/12288.00 MB Q F4 R y 1 1 0 do 5 [ ]: # Complete the function given the variables b,h,d and return the value as "Area","Iz" and "Ic" # This is not about numerical integration, we only need the analytical solution. # Don't change the predefined content, only fill your code in the region "YOUR CODE" Mode: Command % 9 F5 0 T . Markdown v z = ky² 2 6 C F6 Validate Y & 7 8: F7 U * 8 DII F8 I 19 F9 b O ) Ln 1, Col 1 English (United States)…arrow_forwardLet E, B, and S be respectively the Earth-, body-fixed, and sensor frames. These frames are related through the following rotation vectors: B [ABE]" = (1/3,0, 0) rad [ASB]° = (0,0, T) rad Using the Euler symmetric parameters, compute the rotation vector [ASE]°.arrow_forwardFigure 1 shows a six degree of freedom industrial robot. i. Construct the coordinates frames based on the D-H representations. ii. Complete the D-H parameter as illustrated in Table 1.arrow_forward
- Help with the MATLAB code to do the following: Given an audio recording of vehicles, compute Power Spectral Density, 1/3 octave band level, octave band level, A-weighted Leq, C-weighted Leq, L10 (unweighted since sample is short), L90 (unweighted since sample is short). If the measurement is made at approximately 50 ft, and that the vehicles are moving at an average of 65 mph, what is the flow rate Q? If instead, the noise is dominated by 1-2 motorcycles, how fast are they going? Given- -The data are in counts -Calibration factor is 133 uPa/count -Sample rate is 44100Hz. -Remember that in air the dB reference is 20 uPa -Pwelch requires that the inputs are doublesarrow_forwardcreate matlab code and screen shot both filesarrow_forwardpermanent-magnet (pm) genera x Bb Blackboard Learn L STAND-ALONE.mp4 - Google Dri x O Google Drive: ülwgjuó jc lis u O ME526-WindEnergy-L25-Shuja.p x O File | C:/Users/Administrator/Desktop/KFUPM%20Term%232/ME526/ME526-WindEnergy-L25-Shuja.pdf (D Page view A Read aloud T) Add text V Draw Y Highlight O Erase 17 of 26 Wind Farms Consider the arrangement of three wind turbines in the following schematic in which wind turbine C is in the wakes of turbines A and B. Given the following: - Uo = 12 m/s A -XẠC = 500 m -XBC = 200 m - z = 60 m - Zo = 0.3 m U. -r, = 20 m B - CT = 0.88 Compute the total velocity deficit, udef(C) and the velocity at wind turbine C, namely Vc. Activate Windows Go to Settings to activate Windows. Wind Farms (Example Answer) 5:43 PM A 4)) ENG 5/3/2022 I!arrow_forward
- Part III Capstone Problem Interactive Physics - [Lab7Part3.IP] Eile Edit World View Object Define Measure Script Window Help Run StoplI Reset 圖|& 品凸? Time Length of Spring 22 6.00 dx Center of Mass of Rectangle 2 5.000 Tension of Rope 3 Jain@ IFI ... N ot rot ***lad Split 4.000 Velocity of Center of Mass of Rectangle 2 Vx Vx V Vy MM Ve - m/s m/s 3.00 *** m/s Vo ..* lad/s 2 00 Center of Mass of Rectangle 1 1.000 tol rot *.* rad EVelocity of Center of Mass of Rectangle 1 Vx Vx VVy M 0.000 -m/s w 30 m/s w.. MI Ve 母100 *** m/s Vo ... rad/s + EAcceleration of Center of Mass of Rectangle 1 Ax Ax A Ay AUJAI Ae --- m/s^2 ... m/s^2 -- m/s^2 .-- rad/s^2 3.00 Aø Mass M1 = 2.25 kg is at the end of a rope that is 2.00 m in length. The initial angle with respect to the vertical is 60.0° and M1 is initially at rest. Mass M1 is released and strikes M2 = 4.50 kg exactly horizontally. The collision is elastic. After collision, mass M2 is moving on a frictionless surface, but runs into a rough patch 2.00…arrow_forwardI want to run the SGP4 propagator for the ISS (ID = 25544) I got from spacetrack.org in MATLAB. I don't know where to get the inputs of the function. Where do I get the inFile and outFile that is mentioned in the following function. % Purpose: % This program shows how a Matlab program can call the Astrodynamic Standard libraries to propagate % satellites to the requested time using SGP4 method. % % The program reads in user's input and output files. The program generates an % ephemeris of position and velocity for each satellite read in. In addition, the program % also generates other sets of orbital elements such as osculating Keplerian elements, % mean Keplerian elements, latitude/longitude/height/pos, and nodal period/apogee/perigee/pos. % Totally, the program prints results to five different output files. % % % Usage: Sgp4Prop(inFile, outFile) % inFile : File contains TLEs and 6P-Card (which controls start, stop times and step size) % outFile : Base name for five output files %…arrow_forwardZVA @ e SIM a A moodle1.du.edu.om Solve the second order homogeneous differential equation y " -6y'-7y = 0 with intial values y(0) = 0; y'(0) = 1 %3D Maximum file size: 200MB, maximum number of files: 1 Files IIarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
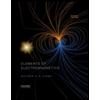
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
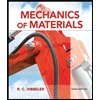
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
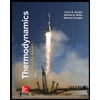
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
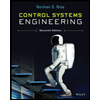
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
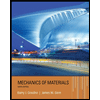
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
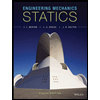
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY