hw3
py
keyboard_arrow_up
School
Arizona State University *
*We aren’t endorsed by this school
Course
591PYTHON
Subject
Electrical Engineering
Date
Jan 9, 2024
Type
py
Pages
3
Uploaded by UltraBoulderHare16
################################################################################
# Created on Fri Aug 24 13:36:53 2018 #
# #
# @author: olhartin@asu.edu; updates by sdm #
# Modified by: Rohith Kalyan Kavadapu #
# ASU ID: 1219703966 # #
# Program to solve resister network with voltage and/or current sources #
################################################################################
import numpy as np # needed for arrays
from numpy.linalg import solve # needed for matrices
from read_netlist import read_netlist # supplied function to read the netlist
import comp_constants as COMP # needed for the common constants
# this is the list structure that we'll use to hold components:
# [ Type, Name, i, j, Value ]
################################################################################
# How large a matrix is needed for netlist? This could have been calculated #
# at the same time as the netlist was read in but we'll do it here #
# Input: #
# netlist: list of component lists #
# Outputs: #
# node_cnt: number of nodes in the netlist #
# volt_cnt: number of voltage sources in the netlist #
################################################################################
def get_dimensions(netlist): #returns the bo of nodes and voltage sources volt_cnt = 0 #initializing the node count
i_array = []
j_array = []
for item in netlist:
i_array.append(item[COMP.I])
j_array.append(item[COMP.J])
if (item[0] == 1):
volt_cnt += 1 # incrementing voltage count i_max = max(i_array) # getting the max i value j_max = max(j_array) # getting the max j value
node_cnt = max(i_max,j_max) # getting the max node value which is the node count
# print(' Nodes ', node_cnt, ' Voltage sources ', volt_cnt)
return node_cnt,volt_cnt
################################################################################
# Function to stamp the components into the netlist #
# Input: #
# y_add: the admittance matrix #
# netlist: list of component lists #
# currents: the matrix of currents #
# node_cnt: the number of nodes in the netlist #
# Outputs: #
# node_cnt: the number of rows in the admittance matrix #
################################################################################
def stamper(y_add,netlist,currents,num_nodes):
# return the total number of rows in the matrix for
# error checking purposes
# add 1 for each voltage source...
(node_cnt,volt_cnt) = get_dimensions(netlist)
current_row = node_cnt - 1 # initializing current row so that the # elements corresponding to the voltage source
# can be added after this.
for comp in netlist: # for each component...
#print(' comp ', comp) # which one are we handling...
# extract the i,j and fill in the matrix...
# subtract 1 since node 0 is GND and it isn't included in the matrix
i = comp[COMP.I] - 1
j = comp[COMP.J] - 1
if ( comp[COMP.TYPE] == COMP.R ): # a resistor
if (i >= 0): # add on the diagonal
y_add[i,i] += 1.0/comp[COMP.VAL] # stamping the resistor values
if (j >= 0):
y_add[j,j] += 1.0/comp[COMP.VAL] if (i >=0 and j >=0):
y_add[i,j] -= 1.0/comp[COMP.VAL]
y_add[j,i] -= 1.0/comp[COMP.VAL]
if (comp[COMP.TYPE] == COMP.VS):
current_row += 1 # incrementing the current row so that the # voltage elements can be stamped at the end of
# stamping resistors
currents[current_row] = comp[COMP.VAL]
if (i >= 0):
y_add[current_row,i] = 1 # Stamping voltage elements
y_add[i,current_row] = 1
if (j >= 0):
y_add[current_row,j] = -1
y_add[j,current_row] = -1
if (comp[COMP.TYPE] == COMP.IS):
if (i >= 0):
currents[i,0] = -comp[COMP.VAL] # Stamping current values in
# current array
if (j >= 0):
currents[j,0] = comp[COMP.VAL]
voltage = solve(y_add,currents) # Solving the linear equation
#EXTRA STUFF HERE!
return (voltage) # should be same as number of rows!
################################################################################
# Start the main program now... #
################################################################################
# Read the netlist!
netlist = read_netlist() # Print the netlist so we can verify we've read it correctly
#for index in range(len(netlist)):
# print(netlist[index])
#print("\n")
(node_cnt,volt_cnt) = get_dimensions(netlist) # Get the no of nodes,no of voltage sources
admittance = np.zeros((node_cnt + volt_cnt, node_cnt + volt_cnt)) # initializing admittance
# arrays
currents = np.zeros((node_cnt+volt_cnt,1)) # initializing currents
voltage = stamper(admittance, netlist, currents, node_cnt) print(voltage)
#EXTRA STUFF H6ERE!
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Analyse several voltage and current situations in different loading scenarios in a simplified radial (transformer to consumption) medium-voltage power system example. There are only 2 nodes in the 35 kV line – node 0 from the 110/35 kV and node 1 with one consumer. The physical characteristics of the line and the loading scenarios are individualized for each student – please see the attached file Excel “WM9P3 Task1 data” for your particular data set.
Briefly reflect on the complexity of the calculations for known input or output voltage, as well as for fixed or voltage-dependent consumption in this simple power system model.
Analyse and discuss the sensitivity of the voltage drop [in % of amplitude and the degrees of phase] with respect to the current level [in %] and to the power factor.
arrow_forward
Which of the following statements is/are true?
(A In purely inductive circuit, the current leads voltage.
(B) In purely resistive circuit, the current lags voltage.
C In purely resistive circuit, the current is in phase with voltage.
D In purely capacitive circuit, the voltage lags current.
arrow_forward
Solve for all parts. Will give thumbs up
arrow_forward
The squaring function, shifted 7 units upward.
arrow_forward
Problem_#03] For the ripple counter below, complete the timing diagram for eight clock
pulses.
HIGH
Jo
CLK
C
Ko
K1
Problem_#04] Construct a timing diagram showing sixteen clock pulses.
HIGH
000
Jo
J2
CLK
C
C
C
Ko
K1
K2
arrow_forward
give a brief short explanation about what tesla does and do a intex refrencing with APA 7th style also provide the refrence list .thanks ( 20 words )
arrow_forward
Difference in isolator and circuit breaker in short.
arrow_forward
eatons
lates in the i
1. Most appliances of the type commonly used in bathrooms, such as hair dryers, elec
shavers, and curling irons, have 2-wire cords. These appliances are
insulated or
protected.
arrow_forward
Explain this diagram
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
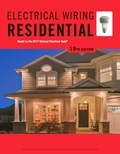
EBK ELECTRICAL WIRING RESIDENTIAL
Electrical Engineering
ISBN:9781337516549
Author:Simmons
Publisher:CENGAGE LEARNING - CONSIGNMENT
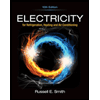
Electricity for Refrigeration, Heating, and Air C...
Mechanical Engineering
ISBN:9781337399128
Author:Russell E. Smith
Publisher:Cengage Learning
Related Questions
- Analyse several voltage and current situations in different loading scenarios in a simplified radial (transformer to consumption) medium-voltage power system example. There are only 2 nodes in the 35 kV line – node 0 from the 110/35 kV and node 1 with one consumer. The physical characteristics of the line and the loading scenarios are individualized for each student – please see the attached file Excel “WM9P3 Task1 data” for your particular data set. Briefly reflect on the complexity of the calculations for known input or output voltage, as well as for fixed or voltage-dependent consumption in this simple power system model. Analyse and discuss the sensitivity of the voltage drop [in % of amplitude and the degrees of phase] with respect to the current level [in %] and to the power factor.arrow_forwardWhich of the following statements is/are true? (A In purely inductive circuit, the current leads voltage. (B) In purely resistive circuit, the current lags voltage. C In purely resistive circuit, the current is in phase with voltage. D In purely capacitive circuit, the voltage lags current.arrow_forwardSolve for all parts. Will give thumbs uparrow_forward
- The squaring function, shifted 7 units upward.arrow_forwardProblem_#03] For the ripple counter below, complete the timing diagram for eight clock pulses. HIGH Jo CLK C Ko K1 Problem_#04] Construct a timing diagram showing sixteen clock pulses. HIGH 000 Jo J2 CLK C C C Ko K1 K2arrow_forwardgive a brief short explanation about what tesla does and do a intex refrencing with APA 7th style also provide the refrence list .thanks ( 20 words )arrow_forward
- Difference in isolator and circuit breaker in short.arrow_forwardeatons lates in the i 1. Most appliances of the type commonly used in bathrooms, such as hair dryers, elec shavers, and curling irons, have 2-wire cords. These appliances are insulated or protected.arrow_forwardExplain this diagramarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- EBK ELECTRICAL WIRING RESIDENTIALElectrical EngineeringISBN:9781337516549Author:SimmonsPublisher:CENGAGE LEARNING - CONSIGNMENTElectricity for Refrigeration, Heating, and Air C...Mechanical EngineeringISBN:9781337399128Author:Russell E. SmithPublisher:Cengage Learning
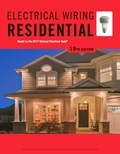
EBK ELECTRICAL WIRING RESIDENTIAL
Electrical Engineering
ISBN:9781337516549
Author:Simmons
Publisher:CENGAGE LEARNING - CONSIGNMENT
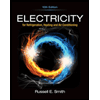
Electricity for Refrigeration, Heating, and Air C...
Mechanical Engineering
ISBN:9781337399128
Author:Russell E. Smith
Publisher:Cengage Learning